C++ Program 1. Design a class that manages a Pet Food Company's quarterly summarized activity. It should contain the following: ( This is all private data and should be coded with the proper C/C++ naming convention ) Company Name - char[40] // This is a static member Quarter - int // This is a static member. Validate to be 1-4 in the setter methodDivision Name - char[40] Bonus Budget Rate - float - set to 0.02 Total Sales - floatTotal Expenses - float 1a. Create a public method named netIncome() which will return total sales - total expenses as float. 2. Create public class methods (Setters and Getters) that load the data values. 3. Do not create getter/setter methods for the BonusBudgetRate item. It can be set in a constructor or initialized to the default value of 0.02. There is no other reference to it. 4. This class needs a header file and a corresponding cpp file. 5. Create a 'driver' program that contains the main() method and includes the header file for PetFoodCompany class. In the driver program, create an instance of the object PetFoodCompany and name this instance to indicate it is about dog food. Set the Quarter to 1 and the Company Name to a name of your choosing. Set the Division Name to "Alpo" or something similar. Display the Company Name, Division Name, and Quarter values Prompt for total sales and total expenses for this object For this instance - display the Company Name, Quarter, Division Name and result of netIncome(). Create a 2nd instance of the object PetFoodCompany and name this instance related to cat food. Set the Division Name to "Purina" or something similar. Prompt for total sales and total expenses for this object For this instance - display the Company Name, Quarter, Division Name and result of netIncome(). Note: Because the items CompanyName and Quarter are declared static - they only need to be set once. Below is example output: Company Name is myCompanyName Current Quarter is 1Division Name is Alpo Current Quarter is 1 Enter Total Sales: 1000 Enter Total Expenses: 600 Net Income = 400 Company Name is myCompanyName Current Quarter is 1Division Name is Purina Enter Total Sales: 1000 Enter Total Expenses: 600 Net Income = 400 The deliverable is a working program (CPPs & H files) and a UML diagram of the this class.
C++ Program 1. Design a class that manages a Pet Food Company's quarterly summarized activity. It should contain the following: ( This is all private data and should be coded with the proper C/C++ naming convention ) Company Name - char[40] // This is a static member Quarter - int // This is a static member. Validate to be 1-4 in the setter methodDivision Name - char[40] Bonus Budget Rate - float - set to 0.02 Total Sales - floatTotal Expenses - float 1a. Create a public method named netIncome() which will return total sales - total expenses as float. 2. Create public class methods (Setters and Getters) that load the data values. 3. Do not create getter/setter methods for the BonusBudgetRate item. It can be set in a constructor or initialized to the default value of 0.02. There is no other reference to it. 4. This class needs a header file and a corresponding cpp file. 5. Create a 'driver' program that contains the main() method and includes the header file for PetFoodCompany class. In the driver program, create an instance of the object PetFoodCompany and name this instance to indicate it is about dog food. Set the Quarter to 1 and the Company Name to a name of your choosing. Set the Division Name to "Alpo" or something similar. Display the Company Name, Division Name, and Quarter values Prompt for total sales and total expenses for this object For this instance - display the Company Name, Quarter, Division Name and result of netIncome(). Create a 2nd instance of the object PetFoodCompany and name this instance related to cat food. Set the Division Name to "Purina" or something similar. Prompt for total sales and total expenses for this object For this instance - display the Company Name, Quarter, Division Name and result of netIncome(). Note: Because the items CompanyName and Quarter are declared static - they only need to be set once. Below is example output: Company Name is myCompanyName Current Quarter is 1Division Name is Alpo Current Quarter is 1 Enter Total Sales: 1000 Enter Total Expenses: 600 Net Income = 400 Company Name is myCompanyName Current Quarter is 1Division Name is Purina Enter Total Sales: 1000 Enter Total Expenses: 600 Net Income = 400 The deliverable is a working program (CPPs & H files) and a UML diagram of the this class.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter12: Adding Functionality To Your Classes
Section: Chapter Questions
Problem 4PP
Related questions
Question
C++ Program
1. Design a class that manages a Pet Food Company's quarterly summarized activity. It should contain the following:
( This is all private data and should be coded with the proper C/C++ naming convention )
Company Name - char[40] // This is a static member Quarter - int // This is a static member. Validate to be 1-4 in the setter methodDivision Name - char[40]Bonus Budget Rate - float - set to 0.02
Total Sales - floatTotal Expenses - float
1a. Create a public method named netIncome() which will return total sales - total expenses as float.
2. Create public class methods (Setters and Getters) that load the data values.
3. Do not create getter/setter methods for the BonusBudgetRate item. It can be set in a constructor or initialized to the default value of 0.02. There is no other reference to it.
4. This class needs a header file and a corresponding cpp file.
5. Create a 'driver' program that contains the main() method and includes the header file for PetFoodCompany class.
In the driver program, create an instance of the object PetFoodCompany and name this instance to indicate it is about dog food. Set the Quarter to 1 and the Company Name to a name of your choosing.Set the Division Name to "Alpo" or something similar. Display the Company Name, Division Name, and Quarter values
Prompt for total sales and total expenses for this object
For this instance - display the Company Name, Quarter, Division Name and result of netIncome().
Create a 2nd instance of the object PetFoodCompany and name this instance related to cat food.
Set the Division Name to "Purina" or something similar. Prompt for total sales and total expenses for this object For this instance - display the Company Name, Quarter, Division Name and result of netIncome().
Note: Because the items CompanyName and Quarter are declared static - they only need to be set once.
Below is example output:
Company Name is myCompanyName
Current Quarter is 1Division Name is Alpo
Current Quarter is 1
Enter Total Sales: 1000
Enter Total Expenses: 600
Net Income = 400
Net Income = 400
Company Name is myCompanyName
Current Quarter is 1Division Name is Purina
Enter Total Sales: 1000
Current Quarter is 1Division Name is Purina
Enter Total Sales: 1000
Enter Total Expenses: 600
Net Income = 400
Net Income = 400
The deliverable is a working program (CPPs & H files) and a UML diagram of the this class.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 9 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
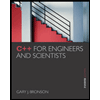
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
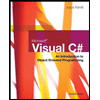
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
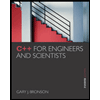
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
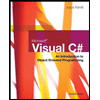
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,