Hello, I am having trouble with this homework assignment for C++ (See below for the output) 2. Implement the following: a. A class named Food. 1. dynamic data member: name 2. accessors and mutators 3. the big three b. A class named Cake that inherits from Food. 1. dynamic data member: topping (type of icing such as chocolate) 2. accessors and mutators 3. the big three c. Create an object of type Food and output its data member. d. Create an object of type Cake and output its data members. e. Test all inherited functions in main. (Cannot change the given int main. Any alteration is not accepted) int main() { cout << endl; Food f1("Bread"); f1.output(); cout << "\nf2: Copy Constructor" << endl; Food f2 = f1; f2.output(); cout << "\nf3: Assignment Overload" << endl; Food f3; f3 = f2; f3.output(); cout << "\nFood: Mutator" << endl; f1.setName("Pizza"); f2.setName("Sandwich"); f1.output(); f2.output(); f3.output(); cout << "\nCake:\n" << endl; Cake c1("Ice Cream Cake", "Chocolate Icing"); c1.output(); cout << "\ns2: Copy Constructor" << endl; Cake c2(c1); c2.output(); cout << "\ns3: Assignment Overload" << endl; Cake c3; c3 = c2; c3.output(); cout << "\nCake: Mutator" << endl; c1.setName("Cheese cake"); c1.setTopping("Strawberry"); c2.setName("Potato Cake"); c2.setTopping("Vanilla"); c1.output(); c2.output(); c3.output(); cout << endl; return 0; } Output from the main function above: (This is what the output should be. Anything else will not be accepted) Bread f2: Copy Constructor Bread f3: Assignment Overload Bread Food: Mutator Pizza Sandwich Bread Cake: Ice Cream Cake, Chocolate Icing s2: Copy Constructor Ice Cream Cake, Chocolate Icing s3: Assignment Overload Ice Cream Cake, Chocolate Icing Cake: Mutator Cheesecake, Strawberry Potato Cake, Vanilla Ice Cream Cake, Chocolate Icing
Hello, I am having trouble with this homework assignment for C++ (See below for the output)
2. Implement the following:
a. A class named Food.
1. dynamic data member: name
2. accessors and mutators
3. the big three
b. A class named Cake that inherits from Food.
1. dynamic data member: topping (type of icing such as chocolate)
2. accessors and mutators
3. the big three
c. Create an object of type Food and output its data member.
d. Create an object of type Cake and output its data members.
e. Test all inherited functions in main. (Cannot change the given int main. Any alteration is not accepted)
int main() {
cout << endl;
Food f1("Bread");
f1.output();
cout << "\nf2: Copy Constructor" << endl;
Food f2 = f1;
f2.output();
cout << "\nf3: Assignment Overload" << endl;
Food f3;
f3 = f2;
f3.output();
cout << "\nFood: Mutator" << endl;
f1.setName("Pizza");
f2.setName("Sandwich");
f1.output();
f2.output();
f3.output();
cout << "\nCake:\n" << endl;
Cake c1("Ice Cream Cake", "Chocolate Icing");
c1.output();
cout << "\ns2: Copy Constructor" << endl;
Cake c2(c1);
c2.output();
cout << "\ns3: Assignment Overload" << endl;
Cake c3;
c3 = c2;
c3.output();
cout << "\nCake: Mutator" << endl;
c1.setName("Cheese cake");
c1.setTopping("Strawberry");
c2.setName("Potato Cake");
c2.setTopping("Vanilla");
c1.output();
c2.output();
c3.output();
cout << endl;
return 0;
}
Output from the main function above: (This is what the output should be. Anything else will not be accepted)
Bread
f2: Copy Constructor
Bread
f3: Assignment Overload
Bread
Food: Mutator
Pizza
Sandwich
Bread
Cake:
Ice Cream Cake, Chocolate Icing
s2: Copy Constructor
Ice Cream Cake, Chocolate Icing
s3: Assignment Overload
Ice Cream Cake, Chocolate Icing
Cake: Mutator
Cheesecake, Strawberry
Potato Cake, Vanilla
Ice Cream Cake, Chocolate Icing

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

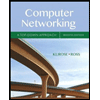
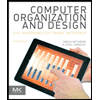
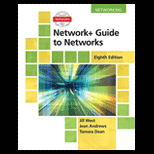
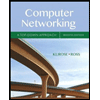
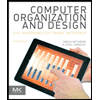
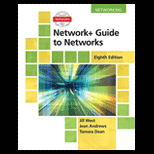
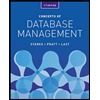
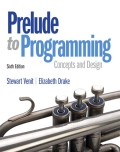
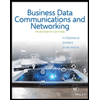