C++ program Create class methods (Setters and Getters) that load the data values. The Setter methods will validate the input data based on the criteria below and load the data into the class if valid. For character arrays, the data must be within the specified data size. (See #3 below) For zipCode, the number must be between 0 and 99999. City and State need to be converted and stored in uppercase. To get started loading the c-strings, review table 10-3 from Week 5. Getters The book does not seem to provide a good example of what a (getter) method that returns a char array should look like. Section 9.9 is very close but uses string as examples instead of c-strings. The best approach is to return a pointer to the c-string- Described as below: char* getName(); // declaration - also known as prototype // Customer is my Class name char* Customer::getName() { // defined member function - Return a pointer to the c-string return name; } cout << YOUR_INSTANCE_HERE.getName() << endl; Setters As for creating a setter method - you can define unsized char arrays in the method header bool Customer::setName(char inputName[]) { // code here.. } 3. Write a program that will allow me to enter data for the class and create and call a method that displays the data for the class. During the input process, pass the input data to the setter method and confirm that the data entered is valid based on the class method return value. If not valid,prompt for me to re-enter the data until it does pass the class method's validation.(A do-while loop works well for this situation) I only need to enter the data for 1 instance of the class. The best way to do this is for the setter methods to return bool instead of void. If the data is valid, return true. Otherwise return false. There is no need to loop for multiple instances of the object. Do not use cin statements inside the class method definitions. Below is the original structure to use to create your class definition. const int NAME_SIZE = 20; const int STREET_SIZE = 30; const int CITY_SIZE = 20; const int STATE_CODE_SIZE = 3; struct Customer { long customerNumber; char name[NAME_SIZE]; char streetAddress_1[STREET_SIZE]; char streetAddress_2[STREET_SIZE]; char city[CITY_SIZE]; char state[STATE_CODE_SIZE]; int zipCode;}; Hints: The const variables need to be defined outside of the class definition. All access to the data in the class must use public methods that you define. DO NOT DIRECTLY ACCESS the customer data items. The class level input methods should return a boolean to confirm the data was loaded and passed any size or data ranges. The customerNumber setter does not need to return a value - It can be a void setter. Set the customerNumber to 1 via the code - not user input. There is no input loop so only 1 set of customer's data is to be entered.
C++
Create class methods (Setters and Getters) that load the data values. The Setter methods will validate the input data based on the criteria below and load the data into the class if valid.
For character arrays, the data must be within the specified data size. (See #3 below)
For zipCode, the number must be between 0 and 99999.
City and State need to be converted and stored in uppercase.
To get started loading the c-strings, review table 10-3 from Week 5.
Getters
The book does not seem to provide a good example of what a (getter) method that returns a char array should look like. Section 9.9 is very close but uses string as examples instead of c-strings.
The best approach is to return a pointer to the c-string- Described as below:
char* getName(); // declaration - also known as prototype
// Customer is my Class name char* Customer::getName() { // defined member function - Return a pointer to the c-string
return name;
}
cout << YOUR_INSTANCE_HERE.getName() << endl;
Setters
As for creating a setter method - you can define unsized char arrays in the method header
bool Customer::setName(char inputName[]) {
// code here..
}
3. Write a program that will allow me to enter data for the class and create and call a method that displays the data for the class.
During the input process, pass the input data to the setter method and confirm that the data entered is valid based on the class method return value. If not valid,prompt for me to re-enter the data until it does pass the class method's validation.(A do-while loop works well for this situation) I only need to enter the data for 1 instance of the class. The best way to do this is for the setter methods to return bool instead of void. If the data is valid, return true. Otherwise return false.
There is no need to loop for multiple instances of the object. Do not use cin statements inside the class method definitions.
Below is the original structure to use to create your class definition.
const int NAME_SIZE = 20;
const int STREET_SIZE = 30;
const int CITY_SIZE = 20;
const int STATE_CODE_SIZE = 3;
struct Customer {
long customerNumber;
char name[NAME_SIZE];
char streetAddress_1[STREET_SIZE];
char streetAddress_2[STREET_SIZE];
char city[CITY_SIZE];
char state[STATE_CODE_SIZE];
int zipCode;};
Hints: The const variables need to be defined outside of the class definition.
All access to the data in the class must use public methods that you define. DO NOT DIRECTLY ACCESS the customer data items.
The class level input methods should return a boolean to confirm the data was loaded and passed any size or data ranges. The customerNumber setter does not need to return a value - It can be a void setter.
Set the customerNumber to 1 via the code - not user input. There is no input loop so only 1 set of customer's data is to be entered.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

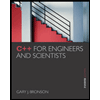
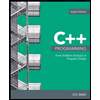
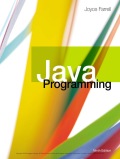
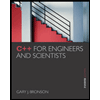
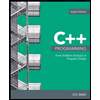
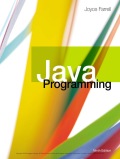