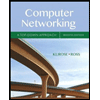
Need functions made in C# please!
a)
Write a function/method called “addStock” that takes:
⦁ An array of Stocks
⦁ The number of stocks in the array
⦁ A string representing a tickerName
⦁ A string representing the company the tickerName represents
⦁ A double representing the price of the stock
⦁ A double representing the quantity of stock owned.
The function must create and add the stock to the array if there is space. The function must return “true” if the addition was successful and “false” otherwise.
b)
Write a function/method called “listOwnedStocks” that takes:
⦁ An array of Stocks
⦁ The number of stocks in the array
The function must return a string containing the information on each stock in the array.
c)
Write a function called “buyStocks” that takes :
⦁ An array of Stocks
⦁ The number of stocks in the array
⦁ A string representing a tickerName
⦁ A double called ‘bought” representing the number of stocks bought
The function must find a stock in the array with that tickerName and add ‘bought’ to the quantity of that stock owned. The function must return a Boolean value where “true” means that the stock was found and updated. It must return “false” when the stock was not found.
d) Write a function called “deleteStock” that takes:
⦁ An array of items
⦁ The number of items in the array
⦁ A string representing a tickerName
The function must remove/delete the stock from the array if a stock with that tickerName exists. It must return a Boolean value where “true” means that the stock was found and deleted. It must return “false” when the stock was not found.

Step by stepSolved in 2 steps

- Exercise 2 This exercise is designed to assess student’s skills of writing programs using arrays and loops. Write a C++ program using array and loop that prompts a user to enter 15 Students’ marks in ProgramDesign and Implementation (PDI) Module in an array. The program sorts the students’ marks in descending order using a loop and displays the marks of all students as well as lowest, highest, average marks, and the class result based on the average module marks. The result criteria is given below:Average is less than 40 = Poor ResultAverage is between 40 to 49 = Fair ResultAverage is less than 50 to 59= Good ResultAverage is less than 60 to 69 = Very Good ResultAverage is between 70 to 79 = Excellent ResultAverage is 80 or greater = Outstanding ResultNote: You must demonstrate the use of arrays and loops. Your code must be properly commented Sample Output:Welcome to Result Management SystemEnter PDI Marks of Student 1: 50Enter PDI Marks of Student 2: 35Enter PDI Marks of Student 3:…arrow_forwardJava a) You are writing a method named getAverage that runs in the main class and is called by the main method. Its only parameter is a 2D double array named values, and it returns a double that is the average of all the values stored in it. Write the complete method header for this method. Include the opening brace for the method. b) Given a String variable named firstName that has been declared and initialized, write the complete statement needed to make firstName all lowercase.arrow_forwardWrite a function/method called “addStock” that takes: An array of Stocks The number of stocks in the array A string representing a tickerName A string representing the company the tickerName represents A double representing the price of the stock A double representing the quantity of stock owned. The function must create and add the stock to the array if there is space. The function must return “true” if the addition was successful and “false” otherwise.arrow_forward
- C++ CODE HELP NEEDED NEED MISSING CODE THAT WILL Read an integer as the number of Goat objects. Assign myGoats with an array of that many Goat objects. For each object, call object's Read() followed by the object's Print(). Ex: If the input is 2 5 87 7 71, then the output is: Goat's age: 5 Goat's weight: 87 Goat's age: 7 Goat's weight: 71 Goat with age 7 and weight 71 is deallocated. Goat with age 5 and weight 87 is deallocated. #include <iostream>using namespace std; class Goat { public: Goat(); void Read(); void Print(); ~Goat(); private: int age; int weight;};Goat::Goat() { age = 0; weight = 0;}void Goat::Read() { cin >> age; cin >> weight;}void Goat::Print() { cout << "Goat's age: " << age << endl; cout << "Goat's weight: " << weight << endl;}Goat::~Goat() { // Covered in section on Destructors. cout << "Goat with age " << age << " and weight " <<…arrow_forwardIn C++Using the code provided belowDo the Following: Modify the Insert Tool Function to ask the user if they want to expand the tool holder to accommodate additional tools Add the code to the insert tool function to increase the capacity of the toolbox (Dynamic Array) USE THE FOLLOWING CODE and MODIFY IT: #define _SECURE_SCL_DEPRECATE 0 #include <iostream> #include <string> #include <cstdlib> using namespace std; class GChar { public: static const int DEFAULT_CAPACITY = 5; //constructor GChar(string name = "john", int capacity = DEFAULT_CAPACITY); //copy constructor GChar(const GChar& source); //Overload Assignment GChar& operator=(const GChar& source); //Destructor ~GChar(); //Insert a New Tool void insert(const std::string& toolName); private: //data members string name; int capacity; int used; string* toolHolder; }; //constructor GChar::GChar(string n, int cap) { name = n; capacity = cap; used = 0; toolHolder = new…arrow_forwardTopic: Array covered in Chapter 7 Do not use any topic not covered in lecture. Write a C++ program to store and process an integer array. The program creates an array (numbers) of integers that can store up to 10 numbers. The program then asks the user to enter up to a maximum of 10 numbers, or enter 999 if there are less than 10 numbers. The program should store the numbers in the array. Then the program goes through the array to display all even (divisible by 2) numbers in the array that are entered by the user. Then the program goes through the array to display all odd (not divisible by 2) numbers in the array that are entered by the user. At the end, the program displays the total sum of all numbers that are entered by the user.arrow_forward
- In C++ struct myGrades { string class; char grade; }; Declare myGrades as an array that can hold 5 sets of data and then set a class string in each position as follows: “Math” “Computers” “Science” “English” “History” and give each class a letter gradearrow_forwardC++ Create a function that will read the following .txt file contents and put them into an array: "apple", "avocado", "apricot","banana", "blackberry", "blueberry", "boysenberry","cherry", "cantaloupe", "cranberry", "coconut", "cucumber", "currant","date", "durian", "dragonfruit","elderberry",arrow_forward1- Write a user-defined function that accepts an array of integers. The function should generate the percentage each value in the array is of the total of all array values. Store the % value in another array. That array should also be declared as a formal parameter of the function. 2- In the main function, create a prompt that asks the user for inputs to the array. Ask the user to enter up to 20 values, and type -1 when done. (-1 is the sentinel value). It marks the end of the array. A user can put in any number of variables up to 20 (20 is the size of the array, it could be partially filled). 3- Display a table like the following example, showing each data value and what percentage each value is of the total of all array values. Do this by invoking the function in part 1.arrow_forward
- C++ Coding: ArraysTrue and False Code function definitions for eoNum() and output(): Both eoNum() and output() are recursive functions. output() stores the even/odd value in an array. Store 0 if the element in the data array is even and store 1 if the element in the data array is odd. eoNum() displays all the values in an array to the console.arrow_forwardIn C++ Create a function named printArray (). The function has two parameters . The first parameter is an array called finalGrades [ containing integer elements . The second argument is an integer named size OfArray , the number of elements in the array. The function prints the contents of arrayit does not return a value. Write the function header and the function body to print the contents of the array.arrow_forward# dates and times with lubridateinstall.packages("nycflights13") library(tidyverse)library(lubridate)library(nycflights13) Qustion: Create a function called date_quarter that accepts any vector of dates as its input and then returns the corresponding quarter for each date Examples: “2019-01-01” should return “Q1” “2011-05-23” should return “Q2” “1978-09-30” should return “Q3” Etc. Use the flight's data set from the nycflights13 package to test your function by creating a new column called quarter using mutate()arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
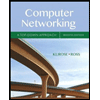
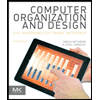
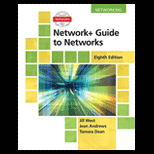
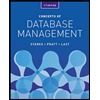
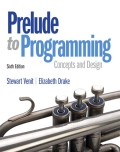
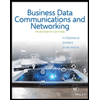