C PROGRAMMING HELP! I tried a threaded reply and bartleby wasn't having it. Soooo: Please create the header file as specified and implement it into my script. Thank you for your help, it is seriously appreciated! The header file deals with the following instruction prompt: Find the roots of the second order nontrivial solution. D2y+a1Dy+a2 = 0 => λ 2+a1 λ +a2 = 0 Create a header file for this step. I believe the portion of the script that needs to be turned into a utilized header file is located in the main() function. Thank you so much for your help, pasted is a copy of my script so far: #include #include void compute_coefficients(double lambda1, double lambda2, double *C1, double *C2, double *C_alpha, double *theta); void display_output(double lambda1, double lambda2, double C1, double C2, double C_alpha, double theta); int main() { int a, b, c; double lambda1, lambda2, C1, C2, C_alpha, theta; printf("Enter a, b, and c where a*D2y + b*Dy + c*y = 0:\n"); scanf("%d%d%d", &a, &b, &c); // Compute the roots of the characteristic equation double discriminant = b * b - 4 * a * c; if (discriminant < 0) { // Complex roots lambda1 = -b / (double)(2 * a); lambda2 = sqrt(-discriminant) / (2 * a); } else if (discriminant == 0) { // Repeated real roots lambda1 = lambda2 = -b / (double)(2 * a); } else { // Distinct real roots lambda1 = (-b + sqrt(discriminant)) / (2 * a); lambda2 = (-b - sqrt(discriminant)) / (2 * a); } // Compute coefficients and display output based on type of roots compute_coefficients(lambda1, lambda2, &C1, &C2, &C_alpha, &theta); display_output(lambda1, lambda2, C1, C2, C_alpha, theta); return 0; } void compute_coefficients(double lambda1, double lambda2, double *C1, double *C2, double *C_alpha, double *theta) { if (lambda1 == lambda2) { // Repeated real roots *C1 = 1; *C2 = 0; *C_alpha = lambda1; *theta = 0; } else if (fabs(lambda1 - lambda2) < 1e-10) { // Distinct real roots (with rounding tolerance) *C1 = 1; *C2 = lambda1; *C_alpha = 0; *theta = 0; } else { // Complex conjugate roots *C1 = 1; *C2 = 0; *C_alpha = sqrt(lambda1 * lambda1 + lambda2 * lambda2); *theta = atan2(lambda2, lambda1); } } void display_output(double lambda1, double lambda2, double C1, double C2, double C_alpha, double theta) { printf("The roots are:\n"); printf("lambda1 = %lf\n", lambda1); printf("lambda2 = %lf\n", lambda2); if (lambda1 == lambda2) { // Repeated real roots printf("The solution is: y(t) = (C1 + C2 * t) * e^(%lf t)\n", lambda1); printf("Please enter initial conditions y(0) and y'(0):\n"); double y0, y_prime0; scanf("%lf %lf", &y0, &y_prime0); C1= y0; C2 = y_prime0 - lambda1 * y0; printf("The solution is: y(t) = ( %lf + %lf * t ) * e^(%lf t)\n", C1, C2, lambda1); } else if (fabs(lambda1 - lambda2) < 1e-10) { // Distinct real roots (with rounding tolerance) printf("The solution is: y(t) = (C1 + C2 * t) * e^(%lf t)\n", lambda1); printf("Please enter initial conditions y(0) and y'(0):\n"); double y0, y_prime0; scanf("%lf %lf", &y0, &y_prime0); C1 = y0; C2 = y_prime0 - lambda1 * y0; printf("The solution is: y(t) = ( %lf + %lf * t ) * e^(%lf t)\n", C1, C2, lambda1); } else { // Complex conjugate roots printf("The solution is: y(t) = e^(%lf t) * (C1 * cos(%lf t) + C2 * sin(%lf t))\n", C_alpha, theta, theta); printf("Please enter initial conditions y(0) and y'(0):\n"); double y0, y_prime0; scanf("%lf %lf", &y0, &y_prime0); C1 = y0; C2 = (y_prime0 - lambda1 * y0) / lambda2; printf("The solution is: y(t) = e^(%lf t) * ( %lf * cos(%lf t) + %lf * sin(%lf t))\n", C_alpha, C1, theta, C2, theta); } } Thank you for your help again! Attached is a copy of the question in the assignment as it may clarify things.
C
Please create the header file as specified and implement it into my script. Thank you for your help, it is seriously appreciated!
The header file deals with the following instruction prompt:
Find the roots of the second order nontrivial solution.
D2y+a1Dy+a2 = 0 => λ 2+a1 λ +a2 = 0
Create a header file for this step.
I believe the portion of the script that needs to be turned into a utilized header file is located in the main() function. Thank you so much for your help, pasted is a copy of my script so far:
#include <stdio.h>
#include <math.h>
void compute_coefficients(double lambda1, double lambda2, double *C1, double *C2, double *C_alpha, double *theta);
void display_output(double lambda1, double lambda2, double C1, double C2, double C_alpha, double theta);
int main()
{
int a, b, c;
double lambda1, lambda2, C1, C2, C_alpha, theta;
printf("Enter a, b, and c where a*D2y + b*Dy + c*y = 0:\n");
scanf("%d%d%d", &a, &b, &c);
// Compute the roots of the characteristic equation
double discriminant = b * b - 4 * a * c;
if (discriminant < 0) { // Complex roots
lambda1 = -b / (double)(2 * a);
lambda2 = sqrt(-discriminant) / (2 * a);
} else if (discriminant == 0) { // Repeated real roots
lambda1 = lambda2 = -b / (double)(2 * a);
} else { // Distinct real roots
lambda1 = (-b + sqrt(discriminant)) / (2 * a);
lambda2 = (-b - sqrt(discriminant)) / (2 * a);
}
// Compute coefficients and display output based on type of roots
compute_coefficients(lambda1, lambda2, &C1, &C2, &C_alpha, &theta);
display_output(lambda1, lambda2, C1, C2, C_alpha, theta);
return 0;
}
void compute_coefficients(double lambda1, double lambda2, double *C1, double *C2, double *C_alpha, double *theta)
{
if (lambda1 == lambda2) { // Repeated real roots
*C1 = 1;
*C2 = 0;
*C_alpha = lambda1;
*theta = 0;
} else if (fabs(lambda1 - lambda2) < 1e-10) { // Distinct real roots (with rounding tolerance)
*C1 = 1;
*C2 = lambda1;
*C_alpha = 0;
*theta = 0;
} else { // Complex conjugate roots
*C1 = 1;
*C2 = 0;
*C_alpha = sqrt(lambda1 * lambda1 + lambda2 * lambda2);
*theta = atan2(lambda2, lambda1);
}
}
void display_output(double lambda1, double lambda2, double C1, double C2, double C_alpha, double theta)
{
printf("The roots are:\n");
printf("lambda1 = %lf\n", lambda1);
printf("lambda2 = %lf\n", lambda2);
if (lambda1 == lambda2) { // Repeated real roots
printf("The solution is: y(t) = (C1 + C2 * t) * e^(%lf t)\n", lambda1);
printf("Please enter initial conditions y(0) and y'(0):\n");
double y0, y_prime0;
scanf("%lf %lf", &y0, &y_prime0);
C1= y0;
C2 = y_prime0 - lambda1 * y0;
printf("The solution is: y(t) = ( %lf + %lf * t ) * e^(%lf t)\n", C1, C2, lambda1);
} else if (fabs(lambda1 - lambda2) < 1e-10) { // Distinct real roots (with rounding tolerance)
printf("The solution is: y(t) = (C1 + C2 * t) * e^(%lf t)\n", lambda1);
printf("Please enter initial conditions y(0) and y'(0):\n");
double y0, y_prime0;
scanf("%lf %lf", &y0, &y_prime0);
C1 = y0;
C2 = y_prime0 - lambda1 * y0;
printf("The solution is: y(t) = ( %lf + %lf * t ) * e^(%lf t)\n", C1, C2, lambda1);
} else { // Complex conjugate roots
printf("The solution is: y(t) = e^(%lf t) * (C1 * cos(%lf t) + C2 * sin(%lf t))\n", C_alpha, theta, theta);
printf("Please enter initial conditions y(0) and y'(0):\n");
double y0, y_prime0;
scanf("%lf %lf", &y0, &y_prime0);
C1 = y0;
C2 = (y_prime0 - lambda1 * y0) / lambda2;
printf("The solution is: y(t) = e^(%lf t) * ( %lf * cos(%lf t) + %lf * sin(%lf t))\n", C_alpha, C1, theta, C2, theta);
}
}
Thank you for your help again! Attached is a copy of the question in the assignment as it may clarify things.
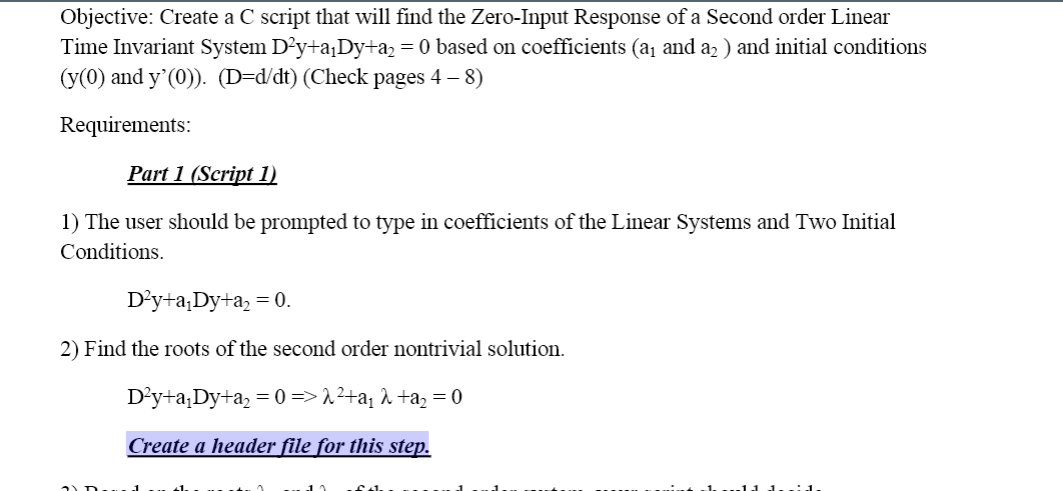

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

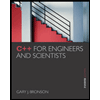
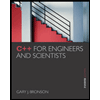