C Programming Question: Will you fix and update function playGame & makeMove using the instructions I provided. There are errors in my code. You do not have to provide an example. The erros in my code needs to be fixed. Please send a picture of the fixed code. Thank you! void playGame() { struct Player playerX; struct Player playerO; printf("Player X, please enter your name\n"); scanf("%s", playerX.playerName); playerX.playerNum = PLAYER_X; playerX.discCount = TWO; printf("Player O, please enter your name\n"); scanf("%s", playerO.playerName); playerO.playerNum = PLAYER_O; playerO.discCount = TWO; printf("%s and %s, let's play Othello!\n", playerX.playerName, playerO.playerName); // Othello board char board[ROW][COL]; // this is really a memory location of board[0][0] // black (X) always goes first int currentPlayer = PLAYER_X; int loop = ZERO; // call function initializeBoard initializeBoard(board); while(loop < FOUR) { // call function displayBoard displayBoard(board); // request the player's move if(currentPlayer == PLAYER_X) { makeMove(&playerX, board); currentPlayer = PLAYER_O; } else if(currentPlayer == PLAYER_O) { makeMove(&playerO, board); currentPlayer = PLAYER_X; } displayStats(playerX); displayStats(playerO); loop++; } } void makeMove(struct Player* player, char playerName, int playerNum, char board[ROW][COL]); { char move[THREE]; int valid = FALSE; // loop until the player enters a valid move while(valid == FALSE) { printf("%s, enter your move location (e.g. B6)\n", player->playerName); scanf("%s", move); printf("%s, you entered %s\n", player->playerName, move); // clears the buffer of extra characters getchar(); // fflush(stdin); int length = (int)strlen(move); if(length == TWO) valid = isValid(move, board); else valid = FALSE; if(valid == FALSE) printf("Invalid move, try again\n\n"); else printf("Valid move\n\n"); } } // good int isValid (char move[THREE], char board[ROW][COL]) { int valid = FALSE; // good valid = isOpen(move, board); // not sure return valid; //good }
C
Will you fix and update function playGame & makeMove using the instructions I provided.
There are errors in my code. You do not have to provide an example. The erros in my code needs to be fixed.
Please send a picture of the fixed code. Thank you!
void playGame()
{
struct Player playerX;
struct Player playerO;
printf("Player X, please enter your name\n");
scanf("%s", playerX.playerName);
playerX.playerNum = PLAYER_X;
playerX.discCount = TWO;
printf("Player O, please enter your name\n");
scanf("%s", playerO.playerName);
playerO.playerNum = PLAYER_O;
playerO.discCount = TWO;
printf("%s and %s, let's play Othello!\n", playerX.playerName, playerO.playerName);
// Othello board
char board[ROW][COL]; // this is really a memory location of board[0][0]
// black (X) always goes first
int currentPlayer = PLAYER_X;
int loop = ZERO;
// call function initializeBoard
initializeBoard(board);
while(loop < FOUR)
{
// call function displayBoard
displayBoard(board);
// request the player's move
if(currentPlayer == PLAYER_X)
{
makeMove(&playerX, board);
currentPlayer = PLAYER_O;
}
else if(currentPlayer == PLAYER_O)
{
makeMove(&playerO, board);
currentPlayer = PLAYER_X;
}
displayStats(playerX);
displayStats(playerO);
loop++;
}
}
void makeMove(struct Player* player, char playerName, int playerNum, char board[ROW][COL]);
{
char move[THREE];
int valid = FALSE;
// loop until the player enters a valid move
while(valid == FALSE)
{
printf("%s, enter your move location (e.g. B6)\n", player->playerName);
scanf("%s", move);
printf("%s, you entered %s\n", player->playerName, move);
// clears the buffer of extra characters
getchar();
// fflush(stdin);
int length = (int)strlen(move);
if(length == TWO)
valid = isValid(move, board);
else
valid = FALSE;
if(valid == FALSE)
printf("Invalid move, try again\n\n");
else
printf("Valid move\n\n");
}
}
// good
int isValid (char move[THREE], char board[ROW][COL])
{
int valid = FALSE; // good
valid = isOpen(move, board); // not sure
return valid; //good
}
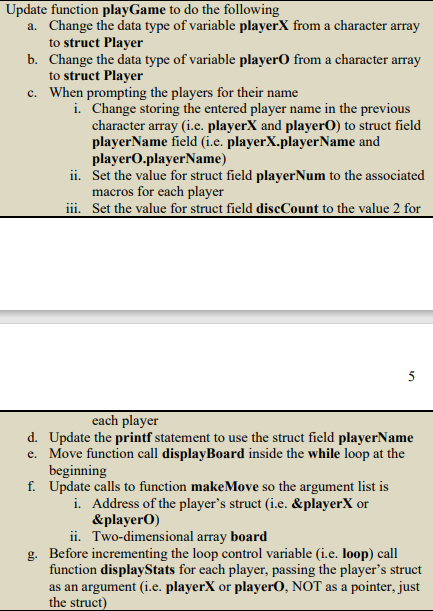
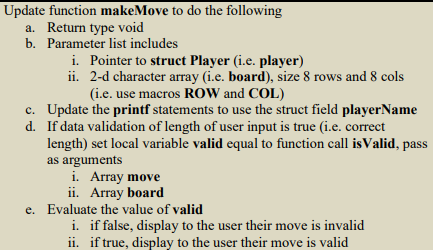

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

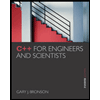
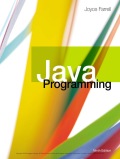
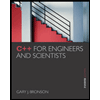
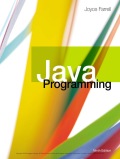
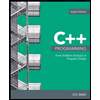
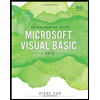