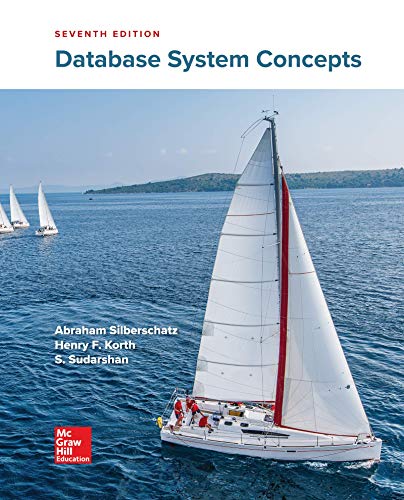
Instructions
Objectives:
- Use a while loop
- Use multiple loop controlling conditions
- Use a boolean method
- Use the increment operator
- Extra credit: Reuse earlier code and call two methods from main
Details:
This assignment will be completed using the Eclipse IDE. Cut and paste your code from Eclipse into the Assignment text window.
This is another password
There will be four acceptable user-password combinations:
- alpha - alpha1
- beta - beta1
- gamma - gamma1
- delta - delta1
If the user types in one of the correct username-password combinations, then the program will output: “Login successful.” Here are a couple of example runs (but your code needs to work for all four user-password combinations):
Username: beta
Type your current password: beta1
Login successful.
Username: delta
Type your current password: delta1
Login successful.
If the user types the wrong username-password combination, then the program will output “Username or password incorrect” and give them another chance. Your program should give them three chances. The first two times the program will also output “Try again,” but on the third unsuccessful try it will output “Too many attempts” and end.
Here is an example of what your program should output when there are three unsuccessful login attempts:
Username: mary
Type your current password: secret
Username or password incorrect.
Try again.
Username: alpha
Type your current password: supersecret
Username or password incorrect.
Try again.
Username: angela
Type your current password: beta1
Username or password incorrect.
Too many attempts.
Here are two examples of initial failed logins followed by a successful login:
Username: alpha
Type your current password: beta1
Username or password incorrect.
Try again.
Username: alpha
Type your current password: alpha1
Login successful.
Username: alpha
Type your current password: beta
Username or password incorrect.
Try again.
Username: mary
Type your current password: beta1
Username or password incorrect.
Try again.
Username: gamma
Type your current password: gamma1
Login successful.
In order to make this happen, ask for the username and password inside a while loop in the main method. Do the checks for a correct combination, and remain in the loop as long as the combinations are not correct – but only for three iterations. Use the increment operator to count the number of attempts. It is going to be tricky to print out the right messages for different situations.
Instead of checking all the username-password combinations in the main method, write a method called userPasswordMatch that takes a username and password as input. Send the username and password values from the main method to userPasswordMatch. If the combination matches one of the four acceptable user-password combinations, userPasswordMatch should return true, otherwise it should return false. (A reason to do this is to make it possible to add new users without touching the main method.) (Hint: You can use the return value of userPasswordMatch to control the while loop.) Don’t do unnecessary tests, i.e. if a test is successful, don’t continue to do more tests.
Extra credit (+10 points):
If the user successfully logs in, then let them change their password like last week. If they successfully log in, then ask them twice for a new password. Work last week’s code into this week’s program, and reuse the passwordChecker method from last week (fix it if necessary) using the same criteria for acceptable passwords.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- PLEASE READ-THIS IS NOT FOR A GRADE!⚠️⚠️⚠️ Using the code below how would you code a Program that should display the average for the first semester and the second semester.Also posted an example Code: import tkinter # Let's create the Tkinter window. window = tkinter.Tk() window.title("GUI") # You will first create a division with the help of Frame class and align them on TOP and BOTTOM with pack() method. top_frame = tkinter.Frame(window).pack() bottom_frame = tkinter.Frame(window).pack(side = "bottom") # Once the frames are created then you are all set to add widgets in both the frames. btn1 = tkinter.Button(top_frame, text = "Button1", fg = "red").pack() #'fg or foreground' is for coloring the contents (buttons) btn2 = tkinter.Button(top_frame, text = "Button2", fg = "green").pack() btn3 = tkinter.Button(bottom_frame, text = "Button3", fg = "purple").pack(side = "left") #'side' is used to left or right align the widgets btn4 = tkinter.Button(bottom_frame, text =…arrow_forwardThe parameter is incorrect. The picture size is not supported. Each image must have a resolution of 40 to 2600 pixels.arrow_forwardWhat may happen if you hovered the mouse over an unstable section of code for a long time?arrow_forward
- Label and Button Widgets. Update the tkhello3.py script so that there are three new buttons in addition to the QUIT button. Pressing any of the three buttons will result in changing the text label so that it will then contain the text of the Button (widget) that was pressed. Hint: you will need three separate handlers or, customize one handler with arguments preset (still three function objects). In pythonarrow_forwardGUI calculator in python - The user enters two integers into the text fields. - When the Add button is pressed, the sum of values in the text fields are shown after the Equals: as a label. - The Clear button clears the values in the text fields and the result of the previous calculation. The cleared values can be blank or zero. - The Quit button closes the GUI window.arrow_forwardDesign and implement an application that prints the verses of thesong “The Twelve Days of Christmas,” in which each verse addsone line. The first two verses of the song areOn the 1st day of Christmas my true love gave to meA partridge in a pear tree.On the 2nd day of Christmas my true love gave to meTwo turtle doves, andA partridge in a pear tree.Use a switch statement in a loop to control which lines getprinted. Hint: Order the cases carefully and avoid the breakstatement. Use a separate switch statement to put the appropriate suffix on the day number (1st, 2nd, 3rd, etc.). The final verseof the song involves all 12 days, as follows:On the 12th day of Christmas, my true love gave to meTwelve drummers drumming,Eleven pipers piping,Ten lords a leaping,Nine ladies dancing,Eight maids a milking,Seven swans a swimming,Six geese a laying,Five golden rings,Four calling birds,Three French hens,Two turtle doves, andA partridge in a pear treearrow_forward
- For this lab, you will be doing the following in C#: 1) Create a Home form with buttons that when clicked, will open the Account, Email, and eventually the Contact forms. The Account form should be opened in "View" mode. 2) Add code to the Login form so the Account form opens when the user clicks "Create New Account". The Account form should be opened in "Modify" mode. 3) Add code to the Login form that validates the user input before allowing the user the access the Home form. For now, just verify the user enters "user1" and "12345" for the username and password respectively.arrow_forwardAn error message popped up when I tried to run the code. "deposit" is not defined "withdraw" is not defined *it was run on visual codearrow_forwardAt runtime, the visibility of controls is decided by their characteristics in the same way that other controls are.arrow_forward
- Prompt: Create a virtual pet care game where players can choose a cute pet and then feed, train, or play-with it. The game is based on a simple game loop, where at the beginning of each loop, the game notifies the player of the current pet status (hungry, sleepy, bored, etc.) The player should then select the corresponding actions to improve the status of the pet. After each action, the game loop is repeated and the pet values are updated. The status should be displayed along with the corresponding messages as well as the menu of actions. Design Requirements: Implement the Pet class Create the base Pet class. It should manage variables that keep track of the pet's state, such as how hungry/sleepy/bored/happy the pet is currently. Include a function that updates the pet's state values to reflect the passage of time. If any of the variables are above some threshold (such as 40 out of 100), the function should output the corresponding message such as "<Pet name> is hungry!" Each…arrow_forwardDo this in JAVA Programmingarrow_forwardWhat may happen if you hovered the mouse over an unstable section of code for a long time?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
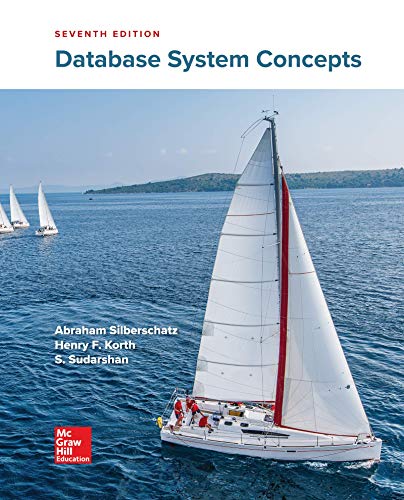
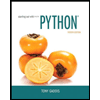
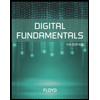
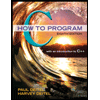
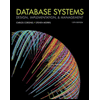
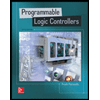