Can I get help with this question ?: write a simple Java class, CD, that models a Compact Disc. Part 1: basic setting up of the CD class The information stored about each CD will include its title, called title; the artist on the CD, called artist; the number of tracks on the CD, called numberOfTracks; the cost of the CD to the library, called costInPence. There should also be a constructor which allows you to pass arguments to initialise these four attributes in the order specified above. For this Part and Part 2, do not add extra parameters. accessor and mutator methods for each field, and a printReport() method which prints, to the terminal window, details of each field. Each piece of information must be printed on a separate line AND in the order given below AND using the exact format given below (though, of course, replacing these example values below with your own values): Title: Greatest Hits Artist: Queen Number of tracks: 12 Cost in pence: 1500
Can I get help with this question ?: write a simple Java class, CD, that models a Compact Disc.
Part 1: basic setting up of the CD class
The information stored about each CD will include
- its title, called title;
- the artist on the CD, called artist;
- the number of tracks on the CD, called numberOfTracks;
- the cost of the CD to the library, called costInPence.
There should also be
- a constructor which allows you to pass arguments to initialise these four attributes in the order specified above. For this Part and Part 2, do not add extra parameters.
- accessor and mutator methods for each field, and
- a printReport() method which prints, to the terminal window, details of each field. Each piece of information must be printed on a separate line AND in the order given below AND using the exact format given below (though, of course, replacing these example values below with your own values):
Title: Greatest Hits
Artist: Queen
Number of tracks: 12
Cost in pence: 1500
Note that any number printed by this method should simply be output as a whole number without any additional symbols or formatting.
Part 2: adding functionality to the CD class
- add three new fields:
- inStock, to store whether the CD is presently in stock or whether it has been borrowed;
- borrower to store the name of the person who is presently borrowing the CD, and
- numberOfTimesBorrowed to store the number of times this CD has been borrowed.
- write a method borrow() which allows a person to borrow a CD and which takes as a parameter the name of the borrower;
- write a method reportInStock() to print to the terminal window whether the CD is in stock or whether it has been borrowed. If it has been borrowed, it should also report the name of the borrower;
- write a method returnCD() which allows a person to return a borrowed CD.
Now amend your printReport() method in the light of your latest changes and make changes so that output is displayed as shown below (but replacing these example values below with your own values)
Title: Greatest Hits
Artist: Queen
Number of tracks: 12
Cost in pence: 1500
In stock: false
Borrower: Dr Bass
Number of times borrowed: 579
Part 3: So far, testing of the
Next
- add a field, hireCost, the cost to a borrower to hire that CD;
- if you think that an extra argument should also be included in the constructor formal parameter list to initialize hireCost then add that parameter at the end of the existing formal parameter list.
- write a method calculateEarnings() which calculates how much money this particular CD has earnt the library;
- do not add an extra field to keep track of the running earnings total.
- Now amend your printReport() method to include this new information i.e. both the hire cost and earnings to date, on two separate lines placed after the other printed information.
Part 4: Introduce a new class, called Borrower to the project. Its purpose is to represent the borrower of the CD. It should have
- two fields surname and libraryId; where the latter is a mix of letters and numbers, and
- a suitable constructor with parameters for only these two fields in the order specified above as well as appropriate accessor methods.
For this last part below, you will modify existing code so that your CD object uses information stored in the Borrower object.
To do this:
- comment out the field declaration for your borrower field;
- add a new field with the old name i.e. borrower, now of type Borrower, to this CD class;
- this means that instead of a field for the name of a borrower, you now have a field that points to a Borrower object;
- amend your borrow() method as follows:
- comment out the present method header;
- add a new method header which takes as its parameter a pointer to a Borrower
To test your code
- create a Borrower object;
- create a CD object, and pass to its constructor, values for the three fields (from Part 1) & the cost of hiring;
- call the method borrow() and pass to it either the identifier name for the Borrower object e.g. borrower1 or, more easily, left click on the Borrower object on the Object Bench;
- now inspect the CD object using the Object Inspector. If you then double click on the borrower field, a new Object Inspector window will open and you should see the fields of the Borrower object you created -- this will confirm that your code for Part 4 is working correctly.
Finally, amend the printReport()method of the CD class so that it also outputs the surname of the borrower which is stored in the Borrower object. To do this, you need to comment out one line of code and replace it with a new line of code.

Step by step
Solved in 3 steps

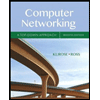
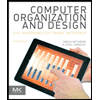
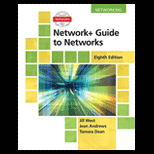
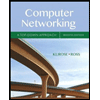
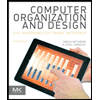
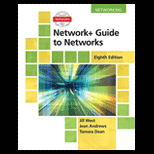
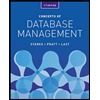
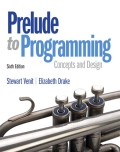
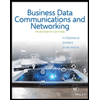