C++ Write the program that outputs the following menu. The program should allow a user to input numbers into an array of integers. The array size should be 10. The first number should be stored in the zero position of the array. The next number should be stored in the next available location. Each option below should be written using a procedure, except Quit. Remember to comment the procedures with (given, task and return). (MENU) 1) Input number. 2) Sort numbers. 3) Output numbers 4) Quit Option 1) Allows the user to input a number and store it into the array. If the array is full no more numbers should be input. When the user select option 1 and the array is full it should output (Memory Full!). This option will only allow one number to be entered. If the user want to input another number they will need to select option 1 again. Option 2) This option should take the array and sort it in ascending order. Use the selection sort to do this. Option 3) This option should output the items in the array to the screen. Option 4) Quit the program. Example Input: Example output:
C++ Write the program that outputs the following menu. The program should allow a user to input numbers into an array of integers. The array size should be 10. The first number should be stored in the zero position of the array. The next number should be stored in the next available location. Each option below should be written using a procedure, except Quit. Remember to comment the procedures with (given, task and return). (MENU) 1) Input number. 2) Sort numbers. 3) Output numbers 4) Quit Option 1) Allows the user to input a number and store it into the array. If the array is full no more numbers should be input. When the user select option 1 and the array is full it should output (Memory Full!). This option will only allow one number to be entered. If the user want to input another number they will need to select option 1 again. Option 2) This option should take the array and sort it in ascending order. Use the selection sort to do this. Option 3) This option should output the items in the array to the screen. Option 4) Quit the program. Example Input: Example output:
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
C++
Write the program that outputs the following menu. The program should allow a user to input numbers into an array of integers. The array size should be 10. The first number should be stored in the zero position of the array. The next number should be stored in the next available location. Each option below should be written using a procedure, except Quit. Remember to comment the procedures with (given, task and return).
(MENU)
1) Input number.
2) Sort numbers.
3) Output numbers
4) Quit
Option 1) Allows the user to input a number and store it into the array. If the array is full no more numbers should be input. When the user select option 1 and the array is full it should output (Memory Full!). This option will only allow one number to be entered. If the user want to input another number they will need to select option 1 again.
Option 2) This option should take the array and sort it in ascending order. Use the selection sort to do this.
Option 3) This option should output the items in the array to the screen.
Option 4) Quit the program.
Example Input:
Example output:
Extra Credit (will be covered in class after due date):
Write the function called factorial. The function is passed in an integer. This function should use recursion to solve the problem. Five factorial is 5 * 4 * 3 * 2 * 1 = 120.
Example run:
Input a number -> 5
The factorial of 5 is 120
Input a number -> 5
The factorial of 5 is 120
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Recommended textbooks for you
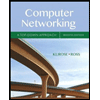
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
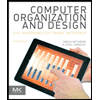
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
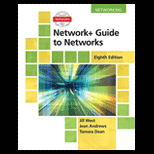
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
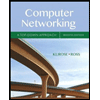
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
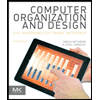
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
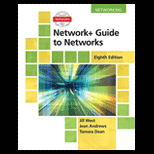
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
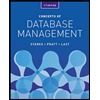
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
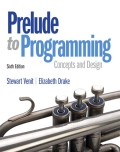
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
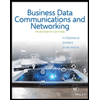
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY