can you add some event in this program like 14 aug ,eid,christmas etc, Step 1 Program Plan:- Initialize all the required header files. Initialize a global array for the the days of all months also define the function to know whether the given year is leap year or not. if the year is leap update the days of feb to 29 . Define the function for finding the dayCode. Finally print the calender of the given year. Step 2 The following is the required C program for Calender Application- #include #define TRUE 1 #define FALSE 0 int daysOfMonths[]= {0,31,28,31,30,31,30,31,31,30,31,30,31}; char *Months[]= { " ", "\n\n\nJanuary", "\n\n\nFebruary", "\n\n\nMarch", "\n\n\nApril", "\n\n\nMay", "\n\n\nJune", "\n\n\nJuly", "\n\n\nAugust", "\n\n\nSeptember", "\n\n\nOctober", "\n\n\nNovember", "\n\n\nDecember" }; int Year(void) { int year; printf("Please enter a year (example: 1999) : "); scanf("%d", &year); return year; } int dayCode(int year) { int daycode; int d1, d2, d3; d1 = (year - 1.)/ 4.0; d2 = (year - 1.)/ 100.; d3 = (year - 1.)/ 400.; daycode = (year + d1 - d2 + d3) %7; return daycode; } int IsLeapYear(int year) { if(year% 4 == FALSE && year%100 != FALSE || year%400 == FALSE) { daysOfMonths[2] = 29; return TRUE; } else { daysOfMonths[2] = 28; return FALSE; } } void calendar(int year, int daycode) { int month, day; for ( month = 1; month <= 12; month++ ) { printf("%s", Months[month]); printf("\n\nSun Mon Tue Wed Thu Fri Sat\n" ); //finding the position for the first date. for ( day = 1; day <= 1 + daycode * 5; day++ ) { printf(" "); } // Print all the dates for one month for ( day = 1; day <= daysOfMonths[month]; day++ ) { printf("%2d", day ); // Is day before Sat? Else start next line Sun. if ( ( day + daycode ) % 7 > 0 ) printf(" " ); else printf("\n " ); } // Set position for next month daycode = ( daycode + daysOfMonths[month] ) % 7; } } int main(void) { int year, daycode, leapyear; year = Year(); daycode = dayCode(year); IsLeapYear(year); calendar(year, daycode); printf("\n"); }
can you add some event in this program like 14 aug ,eid,christmas etc,
Step 1
Program Plan:-
- Initialize all the required header files.
- Initialize a global array for the the days of all months
- also define the function to know whether the given year is leap year or not.
- if the year is leap update the days of feb to 29 .
- Define the function for finding the dayCode.
- Finally print the calender of the given year.
Step 2
The following is the required C program for Calender Application-
#include<stdio.h>
#define TRUE 1
#define FALSE 0
int daysOfMonths[]= {0,31,28,31,30,31,30,31,31,30,31,30,31};
char *Months[]=
{
" ",
"\n\n\nJanuary",
"\n\n\nFebruary",
"\n\n\nMarch",
"\n\n\nApril",
"\n\n\nMay",
"\n\n\nJune",
"\n\n\nJuly",
"\n\n\nAugust",
"\n\n\nSeptember",
"\n\n\nOctober",
"\n\n\nNovember",
"\n\n\nDecember"
};
int Year(void)
{
int year;
printf("Please enter a year (example: 1999) : ");
scanf("%d", &year);
return year;
}
int dayCode(int year)
{
int daycode;
int d1, d2, d3;
d1 = (year - 1.)/ 4.0;
d2 = (year - 1.)/ 100.;
d3 = (year - 1.)/ 400.;
daycode = (year + d1 - d2 + d3) %7;
return daycode;
}
int IsLeapYear(int year)
{
if(year% 4 == FALSE && year%100 != FALSE || year%400 == FALSE)
{
daysOfMonths[2] = 29;
return TRUE;
}
else
{
daysOfMonths[2] = 28;
return FALSE;
}
}
void calendar(int year, int daycode)
{
int month, day;
for ( month = 1; month <= 12; month++ )
{
printf("%s", Months[month]);
printf("\n\nSun Mon Tue Wed Thu Fri Sat\n" );
//finding the position for the first date.
for ( day = 1; day <= 1 + daycode * 5; day++ )
{
printf(" ");
}
// Print all the dates for one month
for ( day = 1; day <= daysOfMonths[month]; day++ )
{
printf("%2d", day );
// Is day before Sat? Else start next line Sun.
if ( ( day + daycode ) % 7 > 0 )
printf(" " );
else
printf("\n " );
}
// Set position for next month
daycode = ( daycode + daysOfMonths[month] ) % 7;
}
}
int main(void)
{
int year, daycode, leapyear;
year = Year();
daycode = dayCode(year);
IsLeapYear(year);
calendar(year, daycode);
printf("\n");
}

Step by step
Solved in 2 steps

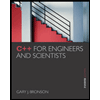
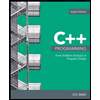
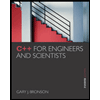
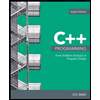
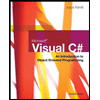