ing to be working with partially filled arrays that are parallel with each other. That means that the row index in multiple arrays identifies different pieces of data for the same person. This is a simple payroll system that just calculates
C++ PLEASE HELP MUST MATCH OUTPUT IN PICS
In this lab, you're going to be working with partially filled arrays that are parallel with each other. That means that the row index in multiple arrays identifies different pieces of data for the same person. This is a simple payroll system that just calculates gross pay given a set of employees, hours worked for the week and hourly rate.
Parallel Arrays
First, you have to define several arrays in your main program
- employee names for each employee
- hourly pay rate for each employee
- total hours worked for each employee
- gross pay for each employee
You can use a global SIZE of 50 to initialize these arrays
Second, you will need a two dimension (2-D) array to record the hours worked each day for an employee. The number of rows for the 2-D array should be the same as the arrays above since each row corresponds to an employee. The number of columns represents days of the week (7 last I looked)
Functions Needed
In this lab, you must read in the employee names first because this identifies how many elements will be in each array. Then you will have to read in the hourly pay rate for each employee
You must implement two functions: getEmployeeNames and getHourlyPay
/* TODO: Create a function called
getEmployeeNames
Arguments :
Name array(first and last name)
Maximum size
Return the number of names filled (Have the user enter "quit" to end input early).
*/
/* TODO: Create a function called
getHourlyPay
Arguments :
NameArray(filled in Step 1)
HourlyPayArray(starts empty)
NumberOfNamesFilled(from Step1)
*/
Two additional functions: getHighestPay and getLowestPay should use the gross pay array and the number of employees to figure out the highest and lowest pay respectively. HINT: return the index of the highest/lowest gross pay so that when you print the answer in main, you can access the gross pay and the name
Nested Loops for Daily Hours
Once you have the names and hourly pay rate, you have to ask the user to enter the hours worked for each employee. Expect to read in hours for 7 days, the user will put 0 for days off. This will require a loop that traverses the rows (each employee) and another loop that traverses the hours worked for the days
Another set of nested loops are needed to sum up the daily hours into total hours worked and use that to calculate the gross pay for the week.
Once you have this, you now have everything you need to create the table which contains only the employee names, the total hours worked and the gross pay (no need to traverse the 2-D array again!)
Program Output
Employee Name, Total Hours and Gross Pay
Use the output information below to space your output in the three arrays that will be printed: employee names, total hours and gross pay.
cout << setw(20) << "Employee Name" << setw(12) << "Total Hours" << setw(10) << "Gross Pay" << endl;
(include <iomanip> in your code to use setw)
Again, you are working with parallel arrays, so use the same index to access elements in each array for an employee.
Employee Name, Total Hours and Gross Pay
Finally, use the functions that you wrote to calculate the highest and lowest gross pay and print this info according to the test case output. These functions only need the gross pay array and the number of elements in it. Each function should return the index of the target value in the gross pay array
STARTER CODE
// TODO:Add function prototypes for all the declared functions
// TODO:Declare two functions to compute the highestPay and lowestPay
// HINT:Each should return the index of the correct value in the grossPay array
/* TODO:Create a function called
getEmployeeNames
Arguments :
Name array(first and last name)
Maximum size
Return the number of names filled.
*/
/* TODO:Create a function called
getHourlyPay
Arguments :
NameArray(filled in Step 1)
HourlyPayArray(starts empty)
NumberOfNamesFilled(from Step1)
*/
int main()
{
//TODO:Declare an array of strings for the employeeNames able to hold a maximum of SIZE employees
//TODO:Declare arrays of double hourlyPay and grossPay, each able to hold a maximum of SIZE employees
//TODO:Declare a 2-D array of doubles for the hours. It should hold a maximum of SIZE employees, each having 7 days
//TODO:Read in the employee names and return the numberOfEmployees
//TODO:Call your function to read in the hourly pay for each of the employees
//TODO:Read in the daily hours for each employee. There will be 7 entries per week
//NOTE:This prints the headers with a set width for each field. Follow this format for the entries
cout << setw(20) << "Employee Name" << setw(12) << " Hours" << setw(10) << "Gross Pay" << endl;
//TODO:For each employee, print: name, total hours and gross pay
//TODO:Call the function that finds the highest gross pay and print the corresponding employee name and gross pay
//HINT:consider finding the Index of the value rather than returning the actual highest gross pay
//TODO:Call the function to find the lowest gross pay and print the corresponding employee name and gross pay
return 0;
}
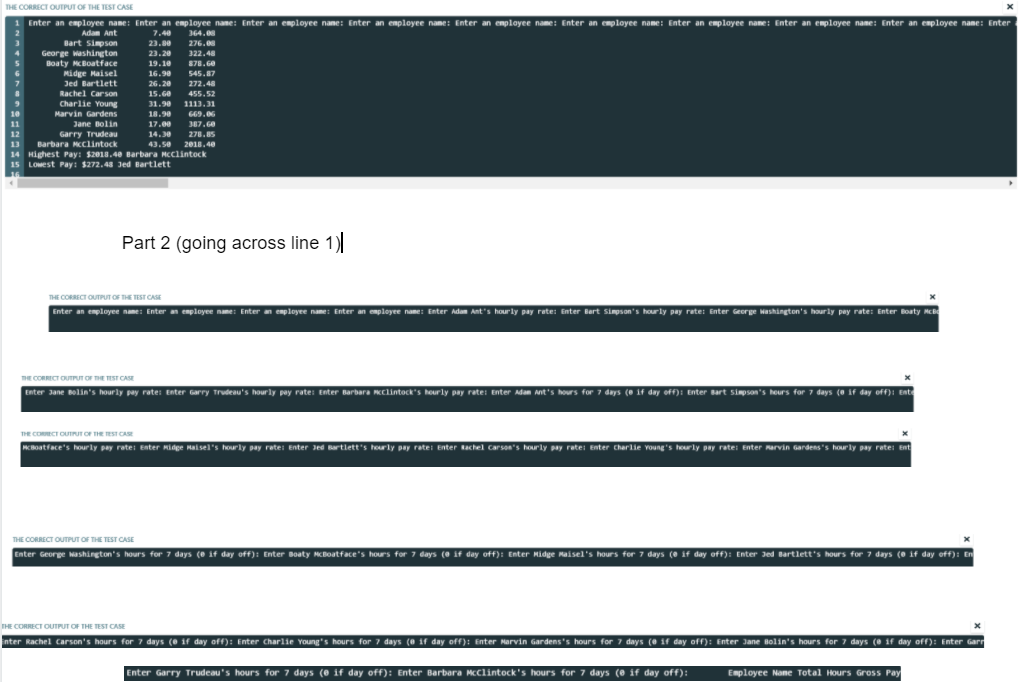
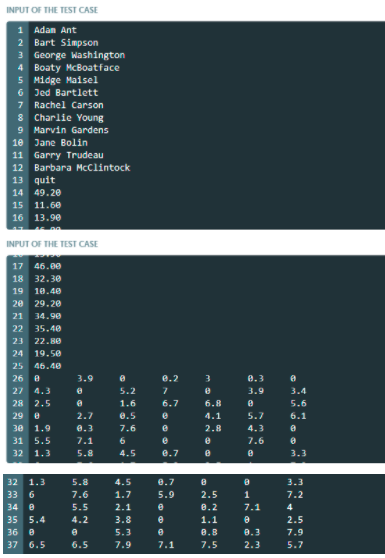

Step by step
Solved in 3 steps with 1 images

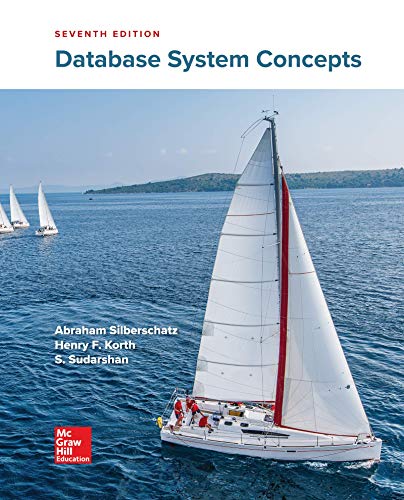
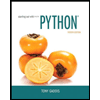
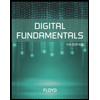
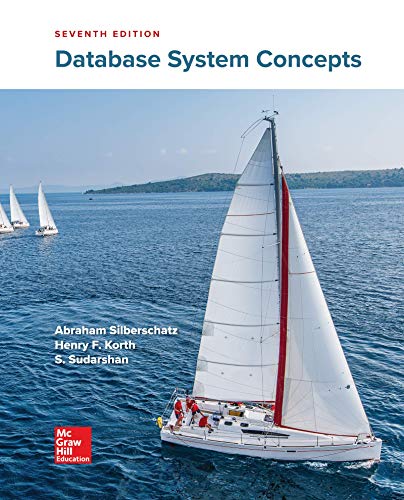
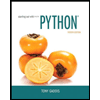
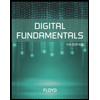
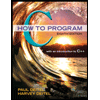
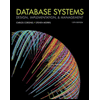
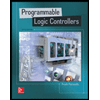