Can you fix the code? Its saying that fatal error no such file as iostream. It is in C++. #include #include using namespace std; const int QUARTERS = 4; //function prototype void printSalesData(double [][QUARTERS], int); int main() { double salesData[3][QUARTERS] {0}; for(int div=0; div<3; ++div){ for(int qtr=0; qtr>salesData[div][qtr]; } } //call statement printSalesData(salesData, 3); return 0; } //function definition void printSalesData(double salesData[][QUARTERS], int numOfDiv) { cout<<"Corpration Sales Report"<
Can you fix the code? Its saying that fatal error no such file as iostream. It is in C++.
#include <iostream>
#include <iomanip>
using namespace std;
const int QUARTERS = 4;
//function prototype
void printSalesData(double [][QUARTERS], int);
int main()
{
double salesData[3][QUARTERS] {0};
for(int div=0; div<3; ++div){
for(int qtr=0; qtr<QUARTERS; ++qtr){
cin>>salesData[div][qtr];
}
}
//call statement
printSalesData(salesData, 3);
return 0;
}
//function definition
void printSalesData(double salesData[][QUARTERS], int numOfDiv) {
cout<<"Corpration Sales Report"<<endl;
//print table header
cout<<setw(10)<<"DIV"<<setw(10)<<"Q1"<<setw(10)<<"Q2"<<setw(10)<<"Q3"<<setw(10)<<"Q4"<<endl;
for(int div=0; div<numOfDiv; ++div) {
//print div number
cout << setw(10) << div;
for(int qtr=0; qtr<QUARTERS; ++qtr) {
cout << setw(10)<<salesData[div][qtr] ;
}
cout<<endl;
}
}
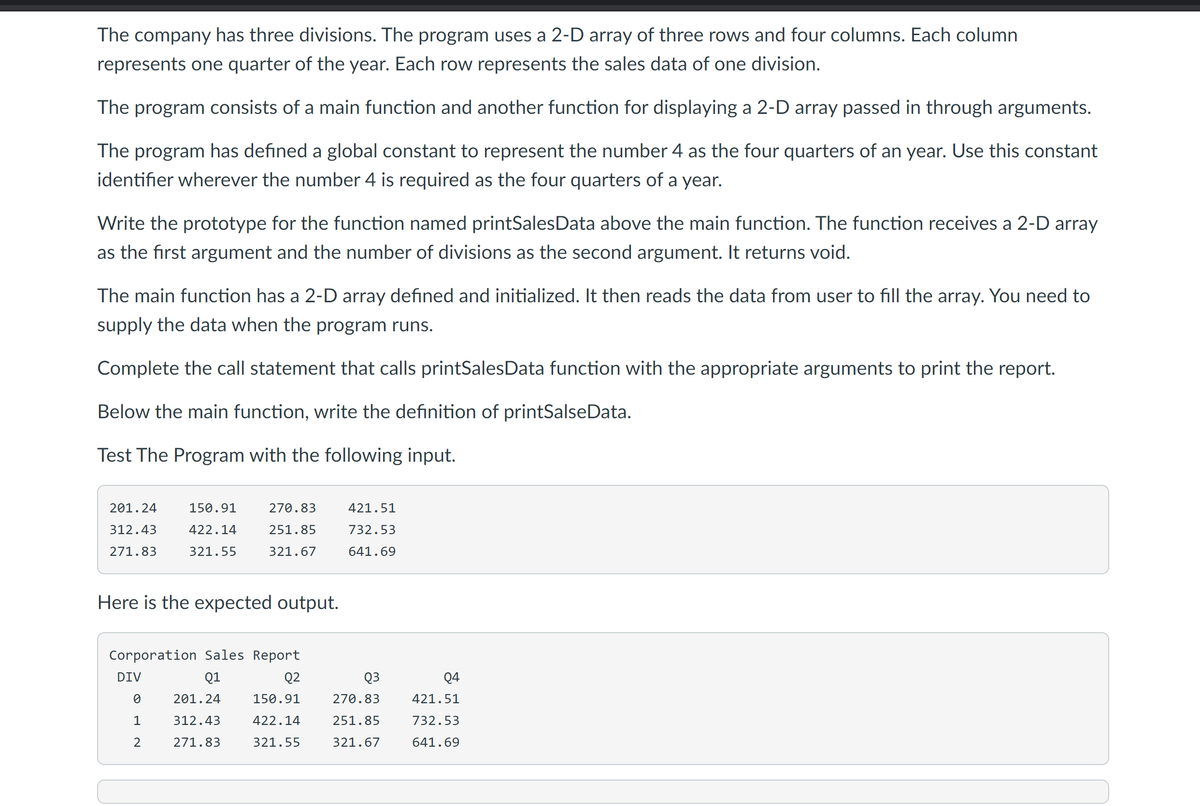

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

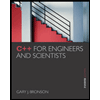
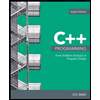
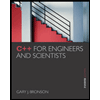
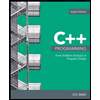