The topic is Booth's algorithm - Explain this C programming code - i have a seminar. //header files #include #include #include int a=0,b=0,c=0,a1=0,b1=0,com[5]={1,0,0,0,0}; int anum[5]={0},anumcp[5] ={0},bnum[5]={0}; int acomp[5]={0},bcomp[5]={0},pro[5]={0},res[5]={0}; // binary function void binary(){ a1 = fabs(a); b1 = fabs(b); int r, r2, i, temp; //for loop for binary for(i = 0; i < 5; i++){ r = a1 % 2; a1 = a1 / 2; r2 = b1 % 2; b1 = b1 / 2; anum[i] = r; anumcp[i] = r; bnum[i] = r2; if(r2 == 0){ bcomp[i] = 1; } if(r == 0){ acomp[i] =1; } } //2's complimanting part for the program c = 0; for( i = 0; i < 5; i++){ res[i] = com[i]+ bcomp[i] + c; if(res[i]>=2){ c = 1; } else c = 0; res[i] = res[i]%2; } for(i = 4; i>= 0; i--){ bcomp[i] = res[i]; } //For negative inputs by user if(a= 0; i--){ res[i] =0; } for( i = 0; i < 5; i++){ res[i] = com[i]+ acomp[i] + c; if(res[i]>=2){ c = 1; } else c = 0; res[i] = res[i]%2; } for(i = 4; i>= 0; i--){ anum[i] = res[i]; anumcp[i] = res[i]; } } if(b=2){ c = 1; } else c = 0; res[i] = res[i]%2; } for(i = 4; i>= 0; i--){ pro[i] = res[i]; printf("%d",pro[i]); } printf(":"); for(i = 4; i>= 0; i--){ printf("%d",anumcp[i]); } } void arshift(){ // arithmetic shift right int temp = pro[4], temp2 = pro[0],i; for(i = 1; i = 0; i--){ printf("%d",pro[i]); } printf(":"); for(i = 4; i>= 0; i--){ printf("%d",anumcp[i]); } } void main(){ int i, q=0; printf("\t\tBOOTH'S MULTIPLICATION ALGORITHM"); printf("\nEnter 2 numbers to multiply: "); printf("\nNumber's must be less than 16"); //simulating for 2 numbers each below 16 do{ printf("\nEnter A: "); scanf("%d",&a); printf("Enter B: "); scanf("%d",&b); }while(a>=16 || b>=16); printf("\n product which is expected = %d", a*b); binary(); printf("\n\n Equivalents of Binary are: "); printf("\nA = "); for(i = 4; i>= 0; i--){ printf("%d",anum[i]); } printf("\nB = "); for(i = 4; i>= 0; i--){ printf("%d",bnum[i]); } printf("\nB'+ 1 = "); for(i = 4; i>= 0; i--){ printf("%d",bcomp[i]); } printf("\n\n"); for(i=0;i"); arshift(); q = anum[i]; } else if(anum[i] == 1 && q == 0){ //subtract and shift for 10 printf("\n-->"); printf("\nSUB B: "); add(bcomp); //add two's complement to implement subtraction arshift(); q = anum[i]; } else{ //add ans shift for 01 printf("\n-->"); printf("\nADD B: "); add(bnum); arshift(); q = anum[i]; } } printf("\nProduct is = "); for(i = 4; i>= 0; i--){ printf("%d",pro[i]); } for(i = 4; i>= 0; i--){ printf("%d",anumcp[i]); } getch(); }
The topic is Booth's algorithm - Explain this C programming code - i have a seminar. //header files #include #include #include int a=0,b=0,c=0,a1=0,b1=0,com[5]={1,0,0,0,0}; int anum[5]={0},anumcp[5] ={0},bnum[5]={0}; int acomp[5]={0},bcomp[5]={0},pro[5]={0},res[5]={0}; // binary function void binary(){ a1 = fabs(a); b1 = fabs(b); int r, r2, i, temp; //for loop for binary for(i = 0; i < 5; i++){ r = a1 % 2; a1 = a1 / 2; r2 = b1 % 2; b1 = b1 / 2; anum[i] = r; anumcp[i] = r; bnum[i] = r2; if(r2 == 0){ bcomp[i] = 1; } if(r == 0){ acomp[i] =1; } } //2's complimanting part for the program c = 0; for( i = 0; i < 5; i++){ res[i] = com[i]+ bcomp[i] + c; if(res[i]>=2){ c = 1; } else c = 0; res[i] = res[i]%2; } for(i = 4; i>= 0; i--){ bcomp[i] = res[i]; } //For negative inputs by user if(a= 0; i--){ res[i] =0; } for( i = 0; i < 5; i++){ res[i] = com[i]+ acomp[i] + c; if(res[i]>=2){ c = 1; } else c = 0; res[i] = res[i]%2; } for(i = 4; i>= 0; i--){ anum[i] = res[i]; anumcp[i] = res[i]; } } if(b=2){ c = 1; } else c = 0; res[i] = res[i]%2; } for(i = 4; i>= 0; i--){ pro[i] = res[i]; printf("%d",pro[i]); } printf(":"); for(i = 4; i>= 0; i--){ printf("%d",anumcp[i]); } } void arshift(){ // arithmetic shift right int temp = pro[4], temp2 = pro[0],i; for(i = 1; i = 0; i--){ printf("%d",pro[i]); } printf(":"); for(i = 4; i>= 0; i--){ printf("%d",anumcp[i]); } } void main(){ int i, q=0; printf("\t\tBOOTH'S MULTIPLICATION ALGORITHM"); printf("\nEnter 2 numbers to multiply: "); printf("\nNumber's must be less than 16"); //simulating for 2 numbers each below 16 do{ printf("\nEnter A: "); scanf("%d",&a); printf("Enter B: "); scanf("%d",&b); }while(a>=16 || b>=16); printf("\n product which is expected = %d", a*b); binary(); printf("\n\n Equivalents of Binary are: "); printf("\nA = "); for(i = 4; i>= 0; i--){ printf("%d",anum[i]); } printf("\nB = "); for(i = 4; i>= 0; i--){ printf("%d",bnum[i]); } printf("\nB'+ 1 = "); for(i = 4; i>= 0; i--){ printf("%d",bcomp[i]); } printf("\n\n"); for(i=0;i"); arshift(); q = anum[i]; } else if(anum[i] == 1 && q == 0){ //subtract and shift for 10 printf("\n-->"); printf("\nSUB B: "); add(bcomp); //add two's complement to implement subtraction arshift(); q = anum[i]; } else{ //add ans shift for 01 printf("\n-->"); printf("\nADD B: "); add(bnum); arshift(); q = anum[i]; } } printf("\nProduct is = "); for(i = 4; i>= 0; i--){ printf("%d",pro[i]); } for(i = 4; i>= 0; i--){ printf("%d",anumcp[i]); } getch(); }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
The topic is Booth's algorithm -
Explain this C programming code - i have a seminar.
//header files
#include
#include
#include
int a=0,b=0,c=0,a1=0,b1=0,com[5]={1,0,0,0,0};
int anum[5]={0},anumcp[5] ={0},bnum[5]={0};
int acomp[5]={0},bcomp[5]={0},pro[5]={0},res[5]={0};
// binary function
void binary(){
a1 = fabs(a);
b1 = fabs(b);
int r, r2, i, temp;
//for loop for binary
for(i = 0; i < 5; i++){
r = a1 % 2;
a1 = a1 / 2;
r2 = b1 % 2;
b1 = b1 / 2;
anum[i] = r;
anumcp[i] = r;
bnum[i] = r2;
if(r2 == 0){
bcomp[i] = 1;
}
if(r == 0){
acomp[i] =1;
}
}
//2's complimanting part for the program
c = 0;
for( i = 0; i < 5; i++){
res[i] = com[i]+ bcomp[i] + c;
if(res[i]>=2){
c = 1;
}
else
c = 0;
res[i] = res[i]%2;
}
for(i = 4; i>= 0; i--){
bcomp[i] = res[i];
}
//For negative inputs by user
if(a= 0; i--){
res[i] =0;
}
for( i = 0; i < 5; i++){
res[i] = com[i]+ acomp[i] + c;
if(res[i]>=2){
c = 1;
}
else
c = 0;
res[i] = res[i]%2;
}
for(i = 4; i>= 0; i--){
anum[i] = res[i];
anumcp[i] = res[i];
}
}
if(b=2){
c = 1;
}
else
c = 0;
res[i] = res[i]%2;
}
for(i = 4; i>= 0; i--){
pro[i] = res[i];
printf("%d",pro[i]);
}
printf(":");
for(i = 4; i>= 0; i--){
printf("%d",anumcp[i]);
}
}
void arshift(){
// arithmetic shift right
int temp = pro[4], temp2 = pro[0],i;
for(i = 1; i = 0; i--){
printf("%d",pro[i]);
}
printf(":");
for(i = 4; i>= 0; i--){
printf("%d",anumcp[i]);
}
}
void main(){
int i, q=0;
printf("\t\tBOOTH'S MULTIPLICATION ALGORITHM");
printf("\nEnter 2 numbers to multiply: ");
printf("\nNumber's must be less than 16");
//simulating for 2 numbers each below 16
do{
printf("\nEnter A: ");
scanf("%d",&a);
printf("Enter B: ");
scanf("%d",&b);
}while(a>=16 || b>=16);
printf("\n product which is expected = %d", a*b);
binary();
printf("\n\n Equivalents of Binary are: ");
printf("\nA = ");
for(i = 4; i>= 0; i--){
printf("%d",anum[i]);
}
printf("\nB = ");
for(i = 4; i>= 0; i--){
printf("%d",bnum[i]);
}
printf("\nB'+ 1 = ");
for(i = 4; i>= 0; i--){
printf("%d",bcomp[i]);
}
printf("\n\n");
for(i=0;i");
arshift();
q = anum[i];
}
else if(anum[i] == 1 && q == 0){
//subtract and shift for 10
printf("\n-->");
printf("\nSUB B: ");
add(bcomp);
//add two's complement to implement subtraction
arshift();
q = anum[i];
}
else{
//add ans shift for 01
printf("\n-->");
printf("\nADD B: ");
add(bnum);
arshift();
q = anum[i];
}
}
printf("\nProduct is = ");
for(i = 4; i>= 0; i--){
printf("%d",pro[i]);
}
for(i = 4; i>= 0; i--){
printf("%d",anumcp[i]);
}
getch();
}
Output
![BOOTH'S MULTIPLICATION ALGORITHM
Enter two numbers to multiply:
Both must be less than 16
Enter A: 12
Enter B: 14
Expected product = 168
Binary Equivalents are:
A = 01100
B = 01110
B'+ 1 = 10010
AR-SHIFT: 00000:00110
-->
AR-SHIFT: 00000:00011
|-->
SUB B: 10010:00011
|AR-SHIFT: 11001:00001
|-->
AR-SHIFT: 11100:10000
|-->
ADD B: 01010:10000
AR-SHIFT: 00101:01000
Product is = 0010101000
|...Program finished with exit code 0
Press ENTER to exit console.]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd0496d2e-fc14-403b-b2ff-4cc548464417%2Ff85c6588-3c67-49f3-ae83-eae65d321afd%2Fyf2o04w_processed.jpeg&w=3840&q=75)
Transcribed Image Text:BOOTH'S MULTIPLICATION ALGORITHM
Enter two numbers to multiply:
Both must be less than 16
Enter A: 12
Enter B: 14
Expected product = 168
Binary Equivalents are:
A = 01100
B = 01110
B'+ 1 = 10010
AR-SHIFT: 00000:00110
-->
AR-SHIFT: 00000:00011
|-->
SUB B: 10010:00011
|AR-SHIFT: 11001:00001
|-->
AR-SHIFT: 11100:10000
|-->
ADD B: 01010:10000
AR-SHIFT: 00101:01000
Product is = 0010101000
|...Program finished with exit code 0
Press ENTER to exit console.]
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
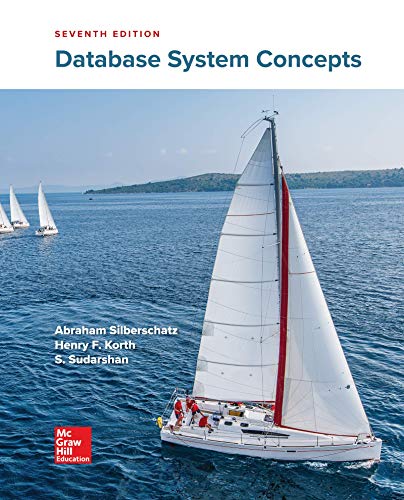
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
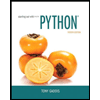
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
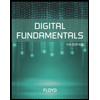
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
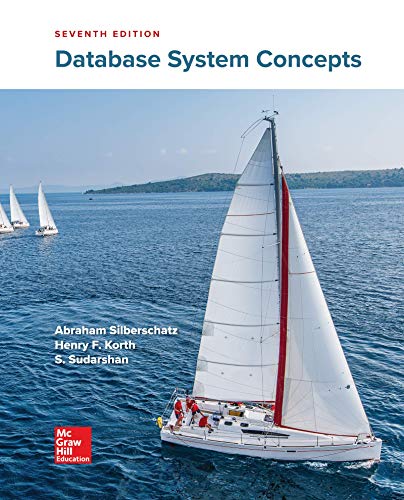
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
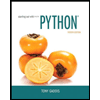
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
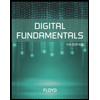
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
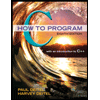
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
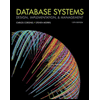
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
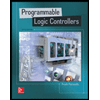
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education