Can you please rewrite this code in c++? import java.util.Queue; import java.util.Scanner; import java.util.concurrent.LinkedBlockingQueue; class AirportSimulation { public static boolean isPlaneComingIntoQueue(int avgInterval) { if (Math.random() < (1.0 / avgInterval)) return true; else return false; } public static boolean isPlaneCrashed(int in, int out, int interval) { if (out - in > interval) { return true; } else { return false; } } public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("The amount of time needed for one plane to land: "); int landTime = in.nextInt(); System.out.print("the amount of time needed for one plane to take off: "); int takeoffTime = in.nextInt(); System.out.print("the average amount of time between arrival of planes to the landing queue: "); int avgArrivalInterval = in.nextInt(); System.out.print("the average amount of time between arrival of planes to the takeoff queue: "); int avgDepartureInterval = in.nextInt(); System.out.print( "the maximum amount of time that a plane can stay in the landing queue without running out of fuel and crashing: "); int crashLimit = in.nextInt(); System.out.print("the total length of time to be simulated: "); int totalTime = in.nextInt(); int totalTimeSpentInLandingQueue = 0, totalTimeSpentInTakeoffQueue = 0; int numPlanesLanded = 0, numPlanesTookoff = 0; int numPlanesCrashed = 0; Queue landingQueue = new LinkedBlockingQueue(); Queue takeoffQueue = new LinkedBlockingQueue(); for (int i = 0; i < totalTime; ++i) { if (isPlaneComingIntoQueue(avgArrivalInterval)) { landingQueue.add(i); } if (isPlaneComingIntoQueue(avgDepartureInterval)) { takeoffQueue.add(i); } while (true) { while (!landingQueue.isEmpty() && isPlaneCrashed(landingQueue.peek(), i, crashLimit)) { landingQueue.remove(); numPlanesCrashed++; } if (!landingQueue.isEmpty()) { int nextPlane = landingQueue.peek(); landingQueue.remove(); numPlanesLanded++; totalTimeSpentInLandingQueue += (i - nextPlane); int j; for (j = i; j < landTime + i && j < totalTime; ++j) { if (isPlaneComingIntoQueue(avgArrivalInterval)) { landingQueue.add(j); } if(isPlaneComingIntoQueue(avgDepartureInterval)) { takeoffQueue.add(j); } } i = j; if (i >= totalTime) { break; } } else { break; } } if (!takeoffQueue.isEmpty()) { int nextPlane = takeoffQueue.peek(); takeoffQueue.remove(); numPlanesTookoff++; totalTimeSpentInTakeoffQueue += (i - nextPlane); int j; for (j = i; j < takeoffTime + i && j < totalTime; ++j) { if (isPlaneComingIntoQueue(avgArrivalInterval)) { landingQueue.add(j); } if (isPlaneComingIntoQueue(avgDepartureInterval)) { takeoffQueue.add(j); } } i = j; } } while (!landingQueue.isEmpty() && isPlaneCrashed(landingQueue.peek(), totalTime, crashLimit)) { landingQueue.remove(); numPlanesCrashed++; } System.out.println("The number of planesthat took off in the simulated time is " + numPlanesTookoff); System.out.println("the number of planes that landed in the simulated time is " + numPlanesLanded); System.out.println("the number of planes that crashed because they ran out of fuel before they could land is " + numPlanesCrashed); System.out.println("the average time that a plane spent in the takeoff queue is " + totalTimeSpentInTakeoffQueue / (double) numPlanesTookoff); System.out.println("the average time that a plane spent in the landing queue is " + totalTimeSpentInLandingQueue / (double) numPlanesLanded); } }
Can you please rewrite this code in c++?
import java.util.Queue;
import java.util.Scanner;
import java.util.concurrent.LinkedBlockingQueue;
class AirportSimulation {
public static boolean isPlaneComingIntoQueue(int avgInterval)
{
if (Math.random() < (1.0 / avgInterval))
return true;
else
return false;
}
public static boolean isPlaneCrashed(int in, int out, int interval)
{
if (out - in > interval)
{ return true; }
else { return false; }
}
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
System.out.print("The amount of time needed for one plane to land: ");
int landTime = in.nextInt();
System.out.print("the amount of time needed for one plane to take off: "); int takeoffTime = in.nextInt();
System.out.print("the average amount of time between arrival of planes to the landing queue: ");
int avgArrivalInterval = in.nextInt();
System.out.print("the average amount of time between arrival of planes to the takeoff queue: ");
int avgDepartureInterval = in.nextInt();
System.out.print( "the maximum amount of time that a plane can stay in the landing queue without running out of fuel and crashing: ");
int crashLimit = in.nextInt();
System.out.print("the total length of time to be simulated: ");
int totalTime = in.nextInt();
int totalTimeSpentInLandingQueue = 0, totalTimeSpentInTakeoffQueue = 0; int numPlanesLanded = 0, numPlanesTookoff = 0;
int numPlanesCrashed = 0; Queue<Integer> landingQueue = new LinkedBlockingQueue<Integer>();
Queue<Integer> takeoffQueue = new LinkedBlockingQueue<Integer>(); for (int i = 0; i < totalTime; ++i)
{
if (isPlaneComingIntoQueue(avgArrivalInterval)) {
landingQueue.add(i);
}
if (isPlaneComingIntoQueue(avgDepartureInterval))
{
takeoffQueue.add(i);
}
while (true)
{
while (!landingQueue.isEmpty() && isPlaneCrashed(landingQueue.peek(), i, crashLimit))
{ landingQueue.remove();
numPlanesCrashed++;
}
if (!landingQueue.isEmpty())
{
int nextPlane = landingQueue.peek();
landingQueue.remove();
numPlanesLanded++;
totalTimeSpentInLandingQueue += (i - nextPlane); int j;
for (j = i; j < landTime + i && j < totalTime; ++j)
{
if (isPlaneComingIntoQueue(avgArrivalInterval))
{
landingQueue.add(j);
}
if(isPlaneComingIntoQueue(avgDepartureInterval))
{
takeoffQueue.add(j);
}
}
i = j;
if (i >= totalTime) {
break;
}
}
else {
break;
}
}
if (!takeoffQueue.isEmpty())
{
int nextPlane = takeoffQueue.peek();
takeoffQueue.remove();
numPlanesTookoff++;
totalTimeSpentInTakeoffQueue += (i - nextPlane);
int j;
for (j = i; j < takeoffTime + i && j < totalTime; ++j)
{
if (isPlaneComingIntoQueue(avgArrivalInterval))
{
landingQueue.add(j);
}
if (isPlaneComingIntoQueue(avgDepartureInterval))
{
takeoffQueue.add(j);
}
}
i = j;
}
}
while (!landingQueue.isEmpty() && isPlaneCrashed(landingQueue.peek(), totalTime, crashLimit))
{
landingQueue.remove();
numPlanesCrashed++;
}
System.out.println("The number of planesthat took off in the simulated time is " + numPlanesTookoff);
System.out.println("the number of planes that landed in the simulated time is " + numPlanesLanded);
System.out.println("the number of planes that crashed because they ran out of fuel before they could land is " + numPlanesCrashed); System.out.println("the average time that a plane spent in the takeoff queue is " + totalTimeSpentInTakeoffQueue / (double) numPlanesTookoff);
System.out.println("the average time that a plane spent in the landing queue is " + totalTimeSpentInLandingQueue / (double) numPlanesLanded); }
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

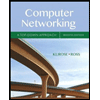
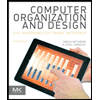
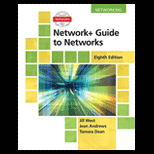
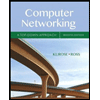
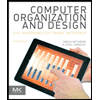
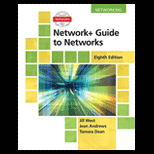
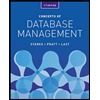
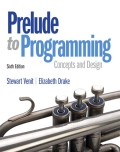
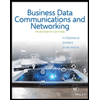