Case Manipulator – Write a C++ program with three functions: upper, lower, and flip. Each function should accept a C-string as an argument. The upper function should step through all the characters in the string, converting each to uppercase. The lower function should step through all the characters in the string converting, each to lowercase. The flip steps through the string, testing each character to determine whether it is upper or lowercase. If upper, it should convert to lower. If lower, it should convert to upper. The main function should accept one string from the user, then pass it to each of the functions. Output: The original string, the uppercase, lowercase, and flipped case strings should all be displayed. The above is my assignment. Below I will post the code that I have so far. I am getting an error message when it comes to displaying the alternative strings and am unsure how to fix it. #include #include #include using namespace std; void upper(string original) { for(int i = 0; i < original.length(); i++) { original[i] = toupper(original[i]); } cout << original << endl; } void lower(string original) { for(int i = 0; i < original.length(); i++) { original[i] = tolower(original[i]); } cout << original << endl; } void flip(string original) { for(int i = 0; i < original.length(); i++) { if(isupper(original[i])) { original[i] = tolower(original[i]); } else { original[i] = toupper(original[i]); } } cout << original << endl; } int main() { string original; cout << "Enter a string: "; cin >> original; cout << "Uppercase String: " << upper(original) << endl; cout << "Lowercase String: " << lower(original) << endl; cout << "Flipped String: " << flip(original) << endl; return 0; }
Case Manipulator – Write a C++ program with three functions: upper, lower, and flip. Each function should accept a C-string as an argument. The upper function should step through all the characters in the string, converting each to uppercase. The lower function should step through all the characters in the string converting, each to lowercase. The flip steps through the string, testing each character to determine whether it is upper or lowercase. If upper, it should convert to lower. If lower, it should convert to upper.
The main function should accept one string from the user, then pass it to each of the functions.
Output: The original string, the uppercase, lowercase, and flipped case strings should all be displayed.
The above is my assignment. Below I will post the code that I have so far. I am getting an error message when it comes to displaying the alternative strings and am unsure how to fix it.
#include <iostream>
#include <cstring>
#include <cctype>
using namespace std;
void upper(string original)
{
for(int i = 0; i < original.length(); i++)
{
original[i] = toupper(original[i]);
}
cout << original << endl;
}
void lower(string original)
{
for(int i = 0; i < original.length(); i++)
{
original[i] = tolower(original[i]);
}
cout << original << endl;
}
void flip(string original)
{
for(int i = 0; i < original.length(); i++)
{
if(isupper(original[i]))
{
original[i] = tolower(original[i]);
}
else
{
original[i] = toupper(original[i]);
}
}
cout << original << endl;
}
int main()
{
string original;
cout << "Enter a string: ";
cin >> original;
cout << "Uppercase String: " << upper(original) << endl;
cout << "Lowercase String: " << lower(original) << endl;
cout << "Flipped String: " << flip(original) << endl;
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

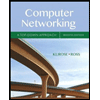
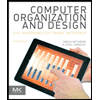
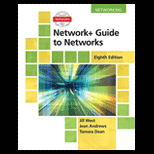
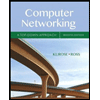
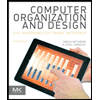
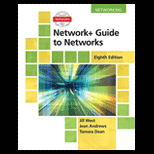
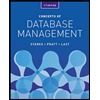
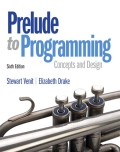
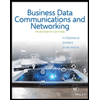