Chapters Covered: • Chapters 1, 2, and 3 Concepts tested in this project • To work with cout and cin objects • To work with variables, constants, and literals • To work with string object • Use of different data types • To follow programming style • Use of basic arithmetic operators • Use of output manipulators (setw, fixed, setprecision) Write a program that uses the script above as a guide without roles, i.e., robot computer and visitor human, to prototype robot greeting in C++. Project Specifications Input • Visitor’s name • An age • Two numbers Output: The program should display the following data: • Complete script described above • Your name as the programmer • Assignment/Project number • Due date Processing Requirements 1. The program should declare and initialize (i.e., create and assign values for) variables and constants to hold (at least) the following data: • Robot Name. This variable will hold the Robot Name. Initialize the variable with “Nao” or a name of your choice. • Visitor Name, this variable will hold the user’s name. • Age. This variable will hold a person’s or a pet’s age. • A variable for Programmer’s Name. Initialize the variable with your full name. • A constant variable for Assignment Number. Initialize the variable with the value 1. • A constant variable for Due Date. Initialize the variable with the due date of this assignment. • Declare Constant variables for o Days of Month. Initialize the variable with the value 30 o Human Year. Initialize the variable with the value 1 o Goldfish Year. Initialize the variable with the value 5 o Dog’s age. Initialize the variable with the value 7 o Examples how to declare constants: ▪ const int ONE_DOG_YEAR = 7; ▪ const int DAYS_PER_MONTH = 30; o Examples how to declare and initialize variables: o string programmer = "Kate Smiths" o string dueDate = "09/06/21"; o int projectNum = 1; o double dogYear = 0; 2. Use the following data for the computations in the program: • 1 month = 30 days • Dog’s age = 7 times human’s age, • Goldfish age = 5 times human’s age 3. Use the above variables when creating the output of the program, for example: cout << “My name is “ << robotName; Where robotName is a variable defined in your program. 4. Your program should do all calculations using C++ expressions (Don’t use calculators for any calculations) 5. Use C++ constants to store all permanent data. Test Plan Test your program with at least two more test cases. Use the given data as an example. Record your data for input and output in the following table. Make sure your tests cover all the possible scenarios.
Chapters Covered: • Chapters 1, 2, and 3 Concepts tested in this project • To work with cout and cin objects • To work with variables, constants, and literals • To work with string object • Use of different data types • To follow programming style • Use of basic arithmetic operators • Use of output manipulators (setw, fixed, setprecision) Write a program that uses the script above as a guide without roles, i.e., robot computer and visitor human, to prototype robot greeting in C++. Project Specifications Input • Visitor’s name • An age • Two numbers Output: The program should display the following data: • Complete script described above • Your name as the programmer • Assignment/Project number • Due date Processing Requirements 1. The program should declare and initialize (i.e., create and assign values for) variables and constants to hold (at least) the following data: • Robot Name. This variable will hold the Robot Name. Initialize the variable with “Nao” or a name of your choice. • Visitor Name, this variable will hold the user’s name. • Age. This variable will hold a person’s or a pet’s age. • A variable for Programmer’s Name. Initialize the variable with your full name. • A constant variable for Assignment Number. Initialize the variable with the value 1. • A constant variable for Due Date. Initialize the variable with the due date of this assignment. • Declare Constant variables for o Days of Month. Initialize the variable with the value 30 o Human Year. Initialize the variable with the value 1 o Goldfish Year. Initialize the variable with the value 5 o Dog’s age. Initialize the variable with the value 7 o Examples how to declare constants: ▪ const int ONE_DOG_YEAR = 7; ▪ const int DAYS_PER_MONTH = 30; o Examples how to declare and initialize variables: o string programmer = "Kate Smiths" o string dueDate = "09/06/21"; o int projectNum = 1; o double dogYear = 0; 2. Use the following data for the computations in the program: • 1 month = 30 days • Dog’s age = 7 times human’s age, • Goldfish age = 5 times human’s age 3. Use the above variables when creating the output of the program, for example: cout << “My name is “ << robotName; Where robotName is a variable defined in your program. 4. Your program should do all calculations using C++ expressions (Don’t use calculators for any calculations) 5. Use C++ constants to store all permanent data. Test Plan Test your program with at least two more test cases. Use the given data as an example. Record your data for input and output in the following table. Make sure your tests cover all the possible scenarios.
Programming with Microsoft Visual Basic 2017
8th Edition
ISBN:9781337102124
Author:Diane Zak
Publisher:Diane Zak
Chapter9: Sequential Access Files And Menus
Section: Chapter Questions
Problem 11E
Related questions
Question
100%
Chapters Covered:
• Chapters 1, 2, and 3
Concepts tested in this project
• To work with cout and cin objects
• To work with variables, constants, and literals
• To work with string object
• Use of different data types
• To follow programming style
• Use of basic arithmetic operators
• Use of output manipulators (setw, fixed, setprecision)
Write a program that uses the script above as a guide without roles, i.e., robot computer and visitor human, to prototype robot greeting in C++.
Project Specifications
Input
• Visitor’s name
• An age
• Two numbers
Output: The program should display the following data:
• Complete script described above
• Your name as the programmer
• Assignment/Project number
• Due date
Processing Requirements
1. The program should declare and initialize (i.e., create and assign values for) variables and constants to hold (at least) the following data:
• Robot Name. This variable will hold the Robot Name. Initialize the variable with “Nao” or a name of your choice.
• Visitor Name, this variable will hold the user’s name.
• Age. This variable will hold a person’s or a pet’s age.
• A variable for Programmer’s Name. Initialize the variable with your full name.
• A constant variable for Assignment Number. Initialize the variable with the value 1.
• A constant variable for Due Date. Initialize the variable with the due date of this assignment.
• Declare Constant variables for
o Days of Month. Initialize the variable with the value 30
o Human Year. Initialize the variable with the value 1
o Goldfish Year. Initialize the variable with the value 5
o Dog’s age. Initialize the variable with the value 7
o Examples how to declare constants:
▪ const int ONE_DOG_YEAR = 7;
▪ const int DAYS_PER_MONTH = 30;
o Examples how to declare and initialize variables:
o string programmer = "Kate Smiths"
o string dueDate = "09/06/21";
o int projectNum = 1;
o double dogYear = 0;
2. Use the following data for the computations in the program:
• 1 month = 30 days
• Dog’s age = 7 times human’s age,
• Goldfish age = 5 times human’s age
3. Use the above variables when creating the output of the program, for example:
cout << “My name is “ << robotName;
Where robotName is a variable defined in your program.
4. Your program should do all calculations using C++ expressions (Don’t use calculators for any calculations)
5. Use C++ constants to store all permanent data.
Test Plan
Test your program with at least two more test cases. Use the given data as an example. Record your data for input and output in the following table. Make sure your tests cover all the possible scenarios.
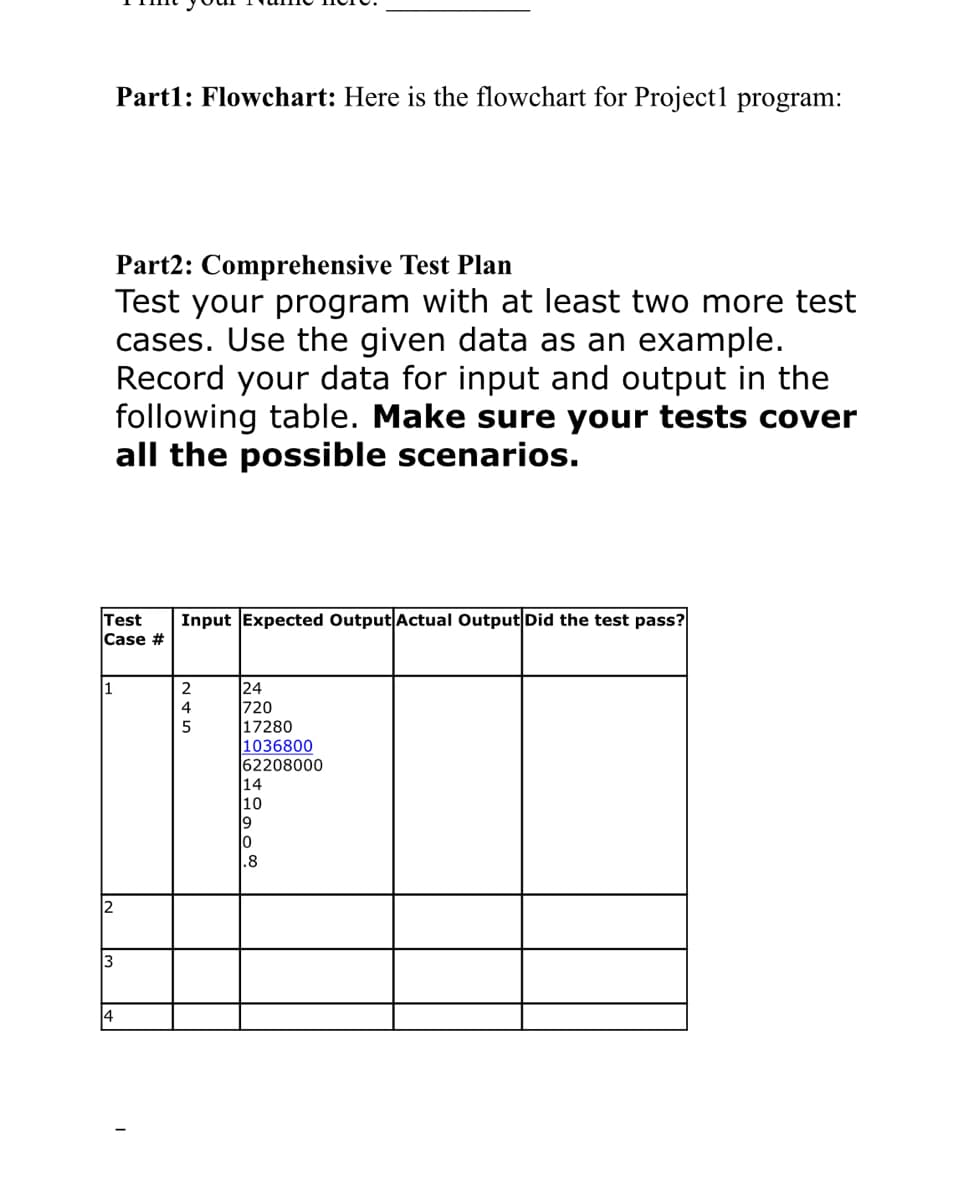
Transcribed Image Text:Part1: Flowchart: Here is the flowchart for Project1 program:
Part2: Comprehensive Test Plan
Test your program with at least two more test
cases. Use the given data as an example.
Record your data for input and output in the
following table. Make sure your tests cover
all the possible scenarios.
Test
Case #
Input Expected Output Actual Output Did the test pass?
1
24
720
17280
1036800
62208000
14
10
4
.8
3
4
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
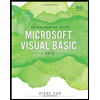
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
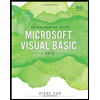
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning