Circular linked list is a form of the linked list data structure where all nodes are connected as in a circe, which means there is no NULL at the end. Circular lists are generally used in applications which needs to go around the list repeatedly. struct Node insertToNull (struct Node last, int data) I" This function is only for empty list struct Node • insertStart (struct Node last, int data) n this question, you are going to implement the insert functions of a circular linked ist in C. The Node struct, print function and the main function with its output is given below: struct Node int data; struct Node "next: struct Node. insertEnd (struet Node last, ant data) void print(struct Node "tailNode) struct Node "p; if (tallNode NULL) struct Node insertSubseg (struct Node last, int data, int item) puts("Empty"): return; p- tailNode + next; do printf("d ".padata); P-p+ next; hile(p t- tailINode next); void main(void) struct Node *tailNode - NULL; tailNode - insertToNull(tailNode, 6); tailNode - insertStart (tailNode, 4); tailNode - insertEnd(taiINode, 8); tailNode - insertEnd (tailNode, 12); tailNode - insertSubseq(tailNode, 9, 8); Output: 468912 print(tailNode): Please follow the rules given below. • Take an extemal pointer which points to n nes of the list, not the head. This will help you to easily insert in the beginning or at the end of the list. • F the functions given below. • I the given search argument cannot be found in the list, inserSuse function should issue an error message.
Circular linked list is a form of the linked list data structure where all nodes are connected as in a circe, which means there is no NULL at the end. Circular lists are generally used in applications which needs to go around the list repeatedly. struct Node insertToNull (struct Node last, int data) I" This function is only for empty list struct Node • insertStart (struct Node last, int data) n this question, you are going to implement the insert functions of a circular linked ist in C. The Node struct, print function and the main function with its output is given below: struct Node int data; struct Node "next: struct Node. insertEnd (struet Node last, ant data) void print(struct Node "tailNode) struct Node "p; if (tallNode NULL) struct Node insertSubseg (struct Node last, int data, int item) puts("Empty"): return; p- tailNode + next; do printf("d ".padata); P-p+ next; hile(p t- tailINode next); void main(void) struct Node *tailNode - NULL; tailNode - insertToNull(tailNode, 6); tailNode - insertStart (tailNode, 4); tailNode - insertEnd(taiINode, 8); tailNode - insertEnd (tailNode, 12); tailNode - insertSubseq(tailNode, 9, 8); Output: 468912 print(tailNode): Please follow the rules given below. • Take an extemal pointer which points to n nes of the list, not the head. This will help you to easily insert in the beginning or at the end of the list. • F the functions given below. • I the given search argument cannot be found in the list, inserSuse function should issue an error message.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 18SA
Related questions
Question
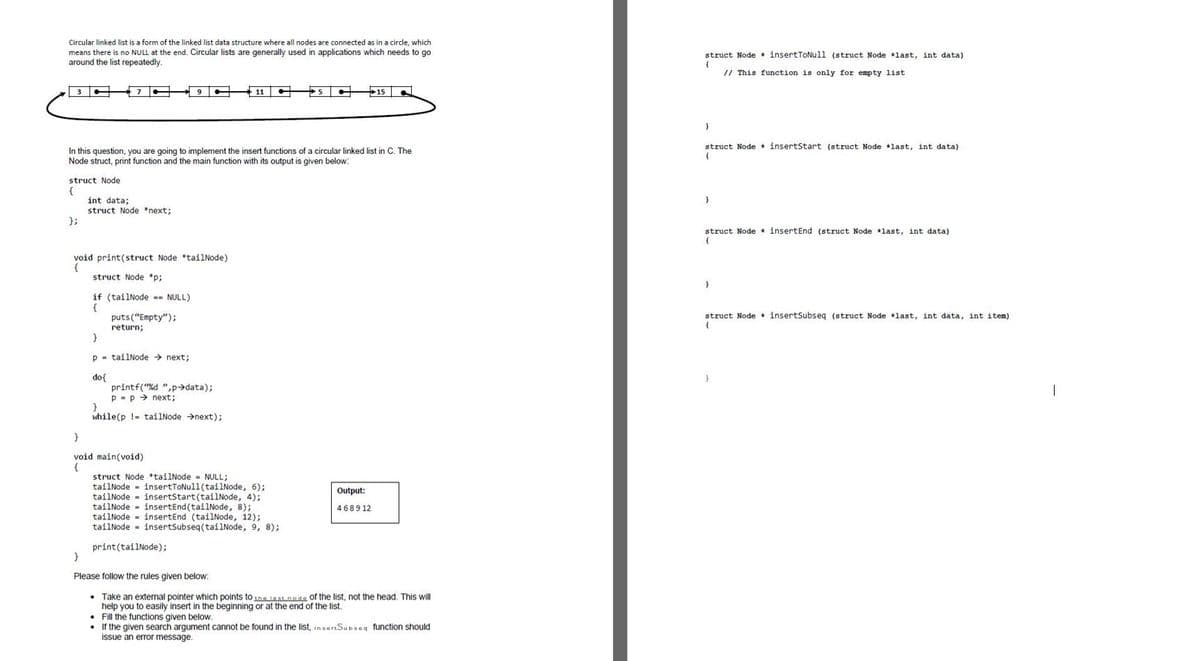
Transcribed Image Text:Circular linked list is a form of the linked list data structure where all nodes are connected as in a circle, which
means there is no NULL at the end. Circular lists are generally used in applications which needs to go
around the list repeatedly.
struct Node * insertTONull (struct Node *last, int data)
// This function is only for empty list
11
5
15
struct Node insertStart (struct Node +last, int data)
In this question, you are going to implement the insert functions of a circular linked list in C. The
Node struct, print function and the main function with its output is given below:
struct Node
{
int data;
struct Node *next;
};
struct Node insertEnd (struct Node *last, int data)
void print(struct Node *tailNode)
struct Node *p;
if (tailNode -- NULL)
struct Node * insertSubseq (struct Node *last, int data, int item)
puts("Empty");
return;
p - tailNode → next;
do{
printf("%d ",p→data);
p - p > next;
while(p !- tailNode →next);
void main(void)
{
struct Node *tailNode - NULL;
tailNode - insertTONull(tailNode, 6);
tailNode - insertStart(tailNode, 4);
tailNode - insertEnd (tailNode, 8);
tailNode - insertEnd (tailNode, 12);
tailNode - insertSubseq(tailNode, 9, 8);
Output:
4689 12
print(tailNode);
Please follow the rules given below:
• Take an external pointer which points to the iast node of the list, not the head. This will
help you to easily insert in the beginning or at the end of the list.
• Fill the functions given below.
• If the given search argument cannot be found in the list, insertSubseg function should
issue an error message.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
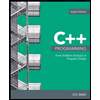
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
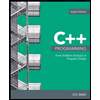
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning