class CircularArray: def __init__(self, lin, st, sz): # Initializing Variables self.start = st self.size = sz self.cir = []*len (lin) # This method will check whether the array is palindrome or not def palindromeCheck(self): #Hard # To Do pass # Remove this line print("= ======Test 4====== ") lin_arr3 = [10, 20, 30, 20, 10, None, None] c3 = CircularArray (lin_arr3, 3, 5) c3.printForward() # This should print: 10, 20, 30, 20, 10 c3.palindromeCheck() # This should print: This array is a palindrome print("==========Test_5==== lin_arr4 = [10, 20, 30, 20, None, None, None] c4 = CircularArray (lin_arr4, 3, 4) c4.printForward() # This should print: 10, 20, 30, 20 c4.palindromeCheck() # This should print: This array is NOT a palindrome
class CircularArray: def __init__(self, lin, st, sz): # Initializing Variables self.start = st self.size = sz self.cir = []*len (lin) # This method will check whether the array is palindrome or not def palindromeCheck(self): #Hard # To Do pass # Remove this line print("= ======Test 4====== ") lin_arr3 = [10, 20, 30, 20, 10, None, None] c3 = CircularArray (lin_arr3, 3, 5) c3.printForward() # This should print: 10, 20, 30, 20, 10 c3.palindromeCheck() # This should print: This array is a palindrome print("==========Test_5==== lin_arr4 = [10, 20, 30, 20, None, None, None] c4 = CircularArray (lin_arr4, 3, 4) c4.printForward() # This should print: 10, 20, 30, 20 c4.palindromeCheck() # This should print: This array is NOT a palindrome
Chapter7: Using Methods
Section: Chapter Questions
Problem 20RQ
Related questions
Question
Use python and do not use any library.
Remove the pass line and complete the code after #todo section
![class CircularArray:
def __init__(self, lin, st, sz):
# Initializing Variables
self.start = st
self.size = sz
self.cir = []*len (lin)
# This method will check whether the array is palindrome or not
def palindromeCheck(self): #Hard
# To Do
pass # Remove this line
print("= ======Test 4===
lin_arr3 = [10, 20, 30, 20, 10, None, None]
c3 = CircularArray (lin_arr3, 3, 5)
c3.printForward() # This should print: 10, 20, 30, 20, 10
c3.palindromeCheck()
# This should print: This array is a palindrome
print("==========Test 5=======
lin_arr4 = [10, 20, 30, 20, None, None, None]
c4 = CircularArray (lin_arr4, 3, 4)
c4.printForward() # This should print: 10, 20, 30, 20
c4.palindromeCheck() # This should print: This array is NOT a palindrome](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F32ab6fba-ef35-4c9b-b666-79b92f56e24b%2F406b9d70-0a08-47d3-8b74-584b3a7e1f8e%2Fltzqe8s_processed.jpeg&w=3840&q=75)
Transcribed Image Text:class CircularArray:
def __init__(self, lin, st, sz):
# Initializing Variables
self.start = st
self.size = sz
self.cir = []*len (lin)
# This method will check whether the array is palindrome or not
def palindromeCheck(self): #Hard
# To Do
pass # Remove this line
print("= ======Test 4===
lin_arr3 = [10, 20, 30, 20, 10, None, None]
c3 = CircularArray (lin_arr3, 3, 5)
c3.printForward() # This should print: 10, 20, 30, 20, 10
c3.palindromeCheck()
# This should print: This array is a palindrome
print("==========Test 5=======
lin_arr4 = [10, 20, 30, 20, None, None, None]
c4 = CircularArray (lin_arr4, 3, 4)
c4.printForward() # This should print: 10, 20, 30, 20
c4.palindromeCheck() # This should print: This array is NOT a palindrome
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
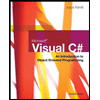
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
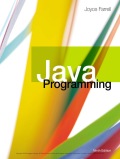
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
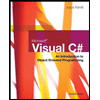
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
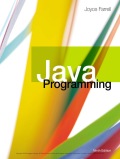
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT