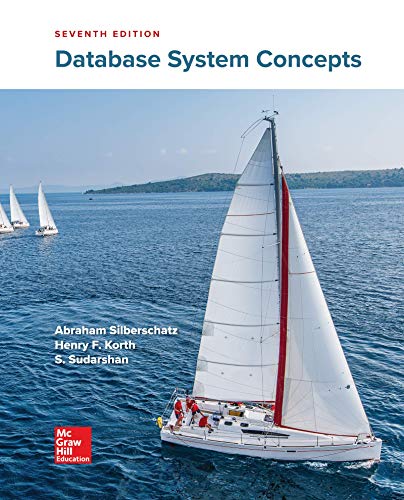
classHighArrayApp
{
public static void main(String[] args)
{
intmaxSize=100;// array size
HighArrayarr;// reference to array
arr=newHighArray(maxSize);// create the array
arr.insert(77);// insert 10 items
arr.insert(99);
arr.insert(44);
arr.insert(55);
arr.insert(22);
arr.insert(88);
arr.insert(11);
arr.insert(00);
arr.insert(66);
arr.insert(33);
arr.display();// display items
intsearchKey=35;// search for item
if(arr.find(searchKey))
System.out.println("Found " + searchKey);
else
System.out.println("Can’t find " + searchKey);
arr.delete(00);// delete 3 items
arr.delete(55);
arr.delete(99);
arr.display();// display items again
}// end main()
}// end class HighArrayApp
// highArray.java
// demonstrates array class with high-level interface
// to run this program: C>java HighArrayApp
////////////////////////////////////////////////////////////////
class HighArray
{
private long[] a; // ref to array a
private int nElems; // number of data items
//-----------------------------------------------------------
public HighArray(int max) // constructor
{
a = new long[max]; // create the array
nElems = 0; // no items yet
}
//-----------------------------------------------------------
public boolean find(long searchKey)
{ // find specified value
int j;
for(j=0; j<nElems; j++) // for each element,
if(a[j] == searchKey) // found item
break; // exit loop before end
if(j==nElems)// gone to end?
returnfalse;// yes, can’t find it
else
return true; // no, found it
} // end find()
//-----------------------------------------------------------
public void insert(long value) // put element into array
{
a[nElems]=value;// insert it
nElems++;// increment size
}
//-----------------------------------------------------------
public boolean delete(long value)
{
intj;
for(j=0;j<nElems;j++)// look for it
if( value == a[j] )
break;
if(j==nElems)// can’t find it
returnfalse;
else// found it
{
for(int k=j; k<nElems; k++) // move higher ones down
a[k]=a[k+1];
nElems--; // decrement size
returntrue;
}
} // end delete()
//-----------------------------------------------------------
public void display() // displays array contents
{
for(int j=0; j<nElems; j++) // for each element,
System.out.print(a[j] + " "); // display it
System.out.println("");
}
//-----------------------------------------------------------
} // end class HighArray
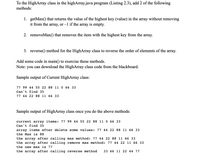

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- JAVA PROGRAM Chapter 7. PC #16. 2D Array Operations Write a program that creates a two-dimensional array initialized with test data. Use any primitive data type that you wish. The program should have the following methods: • getTotal. This method should accept a two-dimensional array as its argument and return the total of all the values in the array. • getAverage. This method should accept a two-dimensional array as its argument and return the average of all the values in the array. • getRowTotal. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The method should return the total of the values in the specified row. • getColumnTotal. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The method should return the…arrow_forwardwhat is the correct syntax in displaying the "8" in the given array? int nums[]={,3,5,8,9,11}; a.)nums[3] b.)nums[4] c.)nums(3) d.)nums(4)arrow_forward1. Implement a class IntArr using dynamic memory. a. data members: capacity: maximum number of elements in the array size: current number of elements in the array array: a pointer to a dynamic array of integers b. constructors: default constructor: capacity and size are 0, array pointer is nullptr user constructor: create a dynamic array of the specified size c. overloaded operators: subscript operator: return an element or exits if illegal index d. "the big three": copy constructor: construct an IntArr object using deep copy assignment overload operator: deep copy from one object to another destructor: destroys an object without creating a memory leak e. grow function: "grow" the array to twice its capacity f. push_back function: add a new integer to the end of the array g. pop_back function: remove the last element in the array h. getSize function: return the current size of the array (not capacity) i. Use the provided main function (next page) and output example Notes a. grow…arrow_forward
- Javaarrow_forwardpublic class arrayOutput { public static void main (String [] args) { final int NUM_ELEMENTS = 3; int[] userVals = new int [NUM_ELEMENTS]; int i; int sumVal; userVals [0] = 3; userVals [1] = 5; userVals [2] = 8; Type the program's output sumVal = 0; for (i = 0; i < userVals.length; ++i) { sumVal= sumVal + userVals [i]; System.out.println (sumVal); } ? ? ??arrow_forwardCan you fix the code please on the first picture shows the error output. // Corrected code #define _CRT_SECURE_NO_WARNINGS #include "LibraryManagement.h" #include "Books.h" #include "DigitalMedia.h" #include "LibraryConfig.h" #include #include #include #include // Include the necessary header for boolean data type // Comparison function for qsort to sort Digital Media by ID int compareDigitalMedia(const void* a, const void* b) { return ((struct DigitalMedia*)a)->id - ((struct DigitalMedia*)b)->id; } // initializing library struct Library initializeLibrary() { struct Library lib; lib.bookCount = 0; lib.ebookCount = 0; lib.digitalMediaCount = 0; // Initialize book array for (int i = 0; i < MAX_BOOK_COUNT; i++) { lib.books[i].commonAttributes.id = -1; // Set an invalid ID to mark empty slot } // Initialize ebook array for (int i = 0; i < MAX_EBOOK_COUNT; i++) { lib.ebooks[i].commonAttributes.id = -1; }…arrow_forward
- Enhanced selection sort algorithm SelectionSortDemo.java package chapter7; /** This program demonstrates the selectionSort method in the ArrayTools class. */ public class SelectionSortDemo { public static void main(String[] arg) { int[] values = {5, 7, 2, 8, 9, 1}; // Display the unsorted array. System.out.println("The unsorted values are:"); for (int i = 0; i < values.length; i++) System.out.print(values[i] + " "); System.out.println(); // Sort the array. selectionSort(values); // Display the sorted array. System.out.println("The sorted values are:"); for (int i = 0; i < values.length; i++) System.out.print(values[i] + " "); System.out.println(); } /** The selectionSort method performs a selection sort on an int array. The array is sorted in ascending order. @param array The array to sort. */ public static void selectionSort(int[] array) { int startScan, index, minIndex, minValue; for (startScan = 0; startScan < (array.length-1); startScan++) { minIndex = startScan; minValue…arrow_forwardvoid changeAll(int x[]) { x[0] = x[0] + 5; return; The first element in an array would be increased by 5 The array would be the same because it was passed by value x would be increased by 5 All the elements in the passed array would be incremented by 5arrow_forwardquery the user for the size of an array of integers build an array of integers and fill it with random integer values. The values will range between 0 and 100 display the array clone the array display the cloned array sort the cloned array display the original array and the sorted cloned array create another array that is double the size of the cloned array and copy the data from the cloned array into the new array. Then fill the remaining cells in the new array with random values. These will also range between 0 and 100.Note: int[] newArray = new int[clone.lenght*2] Write program in jvaarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
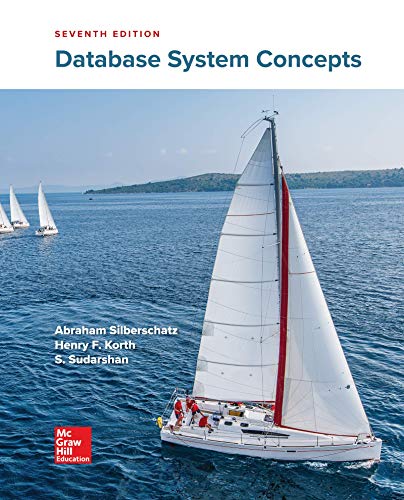
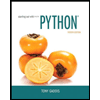
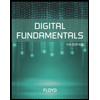
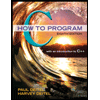
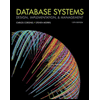
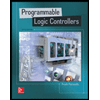