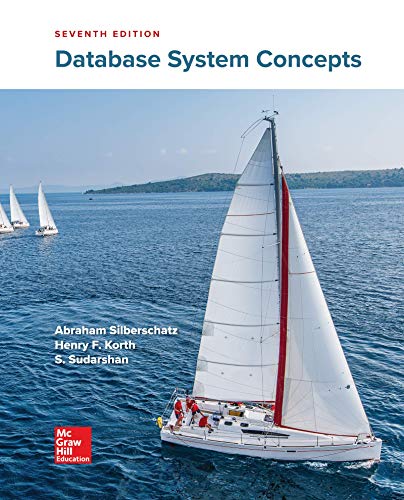
Concept explainers
public class ArrayCommonOperations
{
public static void main(String [] args)
{
//*** Task #1: Define and instantiate an array of integer numbers, with 10 elements
//*** Task #2: Fill in the array with integer numbers from 1 to 100
//*** Task #3: define another array, named temp, and copy the initial array in it.
//* This allows to preserve the original array
//*** Task #4: Define the variables you need to calculate the following values,
//* and initialize them with appropriate values.
//*** Task #5: Print the original array
//*** Task #6: Calculate the sum of all values
//*** Task #7: Count the number of even values
//*** Task #8: Calculate the minimum value in the array
//*** Task #9: Calculate the maximum value in the array
//*** Task #10: Replace the elements that are divisible by 3, with their value plus 2
//*** Task #11: Display the new array after replacement
//*** Task #12: Display the calculated values.
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- C PROGRAMMING LANGUAGEarrow_forward6. Show the printout of the following code: public class ArraysBasic { public static void main(String[] args) { int A[] = {1, 2, 3}; } System.out.println("array A: " + A[0] +""+A[1]+""+ A[2]); System.out.println("array B: " + A[0] + " " + A[1] + " " + A[2]); zeroArray(A); A = new int[5]; System.out.println("array C: " + A[0] + " " + A[1] + " " + A[3] + " " + A[4]); } } public static void zeroArray(int[] list) { for (int j = 0; j < list.length; ++j) { list[j] = 99;arrow_forward4. Remove all occurrences of a particular element from an array Consider an array named source. Write a method/function named removeAll( source, size, element) that removes all the occurrences of the given element in the source array. You must execute the method by passing an array, its size and the element to be removed. After calling the method, print the array to show whether all the occurrences of the element have been removed properly. nmm" Use Python Example: source=[10,2,30,2,50,2,2,60,0,0] removeAll(source,8,2) After calling removeAll(source,8,2), all the occurrences of 2 must be removed. Printing the array afterwards should give the output as: [ 10,30,50,60,0,0,0,0,0,0]arrow_forward
- Language: Javaarrow_forwardpublic int[] selectionSort(int[] array) {for (int i = 0; i < array.length - 1; i++) {int min = array[i];int minIndex = i;for (int j = i + 1; j < array.length; j++) {if (min > array[j]) {min = array[j];minIndex = j;}}if (minIndex == i) {array[minIndex] = array[i];array[i] = min;}}return array;}arrow_forwardProgramming language: Processing from Java Question attached as photo Topic: Use of Patial- Full Arraysarrow_forward
- Two dimension array in C:Create a two dimension array of integers that is 5 rows x 10 columns.Populate each element in the first 2 rows (using for loops) with the value 5.Populate each element of the last three rows (using for loops) with the value 7.Write code (using for loops) to sum all the elements of the first three columns and output thesum to the screen. without using #definearrow_forward5arrow_forwardMethod Details: public static java.lang.String getArrayString(int[] array, char separator) Return a string where each array entry (except the last one) is followed by the specified separator. An empty string will be return if the array has no elements. Parameters: array - separator - Returns: stringThrows:java.lang.IllegalArgumentException - When a null array parameter is providedarrow_forward
- CODE IN JAVA design a program that grades arithmetic quizzes as follows: (Use the below startup code for Quizzes.java and provide code as indicated by the comments) 1. Ask the user how many questions are in the quiz. 2. Use a for loop to load the array. Ask the user to enter the key (that is, the correct answers). There should be one answer for each question in the quiz, and each answer should be an integer. They can be entered on a single line, e.g., 34, 7, 13, 100, 8 might be the key for a 5-question quiz. You will need to store the key in an array called "key". 3. Ask the user to enter the student's answers for the quiz to be graded. There needs to be one answer for each question. Note that each answer can simply be compared to the key as it is entered. If the answer is correct, add 1 to a correct answer counter. need this in a separate for loop from the loop used to load the array. 4. When the user has entered all of the answers to be graded, print the number correct…arrow_forwardJava - When using an array to do a binary search, what additional requirement is placed on the array?arrow_forward/*** creates an array of 1000 integers where each element matches its index* * @return the array that is created*/public static int[] makeNumberArray() {arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
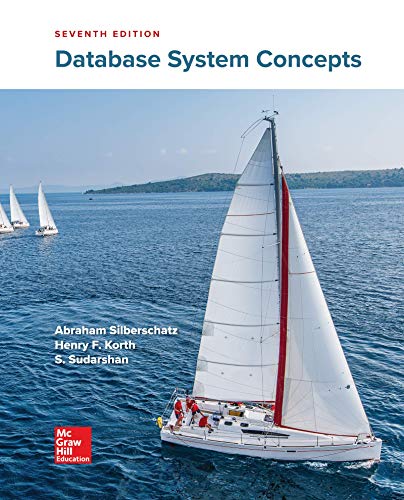
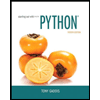
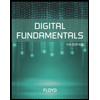
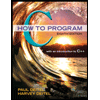
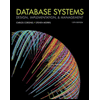
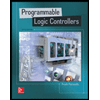