Class Rectangle: An instance of the class Rectangle represents a valid axis-aligned rectangle. It has several instance variables that one needs to use to describe a rectangle, as well as methods that operate on these variables. Rectangle instance variables: Class Rectangle will have the following instance variables: • x – a double that represents the x coordinate of the lower left vertex of the rectangle • y – a double that represents the y coordinate of the lower left vertex of the rectangle • width – a double that represents the width of the rectangle • height – a double that represents the height of the rectangle • color – a String that represents the color of the rectangle For the rectangle to be valid, the coordinates of all vertices must be between 0 and 1 inclusive. The color can be one of the following: “black”, “red”, “green”, “blue”. Rectangle constructor: The constructor should be: Rectangle (double x, double y, double height, double width, String color) Parameters are, in order, the x and y coordinates of the lower left corner, the height, the width, and the color of the rectangle. The constructor should check that these values represent a valid rectangle. If they do, then it should initialize the rectangle to these values. Otherwise, the date rectangle should be initialized to a rectangle of width 1, height 1, color “red” and lower left vertex at coordinates (0, 0). 2 Rectangle methods: Method Description double getX() Returns the x coordinate of this object. double getY() Returns the y coordinate of this object. double getWidth() Returns the width of this object. double getHeight() Returns the height of this object. String getColor() Returns the color of this object. void setX(double x) Sets the x coordinate of this object to x. If this results in an invalid rectangle, it leaves the x coordinate unchanged. void setY(double y) Sets the y coordinate of this object to y. If this results in an invalid rectangle, it leaves the y coordinate unchanged. void setWidth(double w) Sets the width of this object to w. If this results in an invalid rectangle, it leaves the width unchanged. void setHeight(double h) Sets the height of this object to h. If this results in an invalid rectangle, it leaves the height unchanged. void setColor (String c) Sets the color of this object to c. If c is invalid, it leaves the color coordinate unchanged. String toString() Returns a String representation of the rectangle containing the values of all instance variables. boolean equals(Rectangle rect) Returns true if rect represents the same rectangle as this object, and false otherwise. double computePerimeter() Computes and returns the perimeter of this rectangle double computeArea() Computes and returns the area of this rectangle boolean containsPoint(double x, double y) Returns true if this rectangle contains point (x, y) boolean containsRectangle(Rectangle rect) Returns true if this rectangle contains rectangle rect boolean intersects(Rectangle rect) Returns true if rectangle rect and this rectangle intersect. static boolean isValid(double x, double y, double w, double h) Static method. Returns true if the rectangle with lower left vertex at (x,y), width w and height h is valid, and false otherwise. void show() Extra Credit: Displays the rectangle on the screen. See details below. Make sure that the names of your methods match those listed above exactly, including capitalization. The number of parameters and their order must also match. You can change the parameter names if you want. All methods should be public.
Class Rectangle: An instance of the class Rectangle represents a valid axis-aligned rectangle. It has several instance variables that one needs to use to describe a rectangle, as well as methods that operate on these variables. Rectangle instance variables: Class Rectangle will have the following instance variables: • x – a double that represents the x coordinate of the lower left vertex of the rectangle • y – a double that represents the y coordinate of the lower left vertex of the rectangle • width – a double that represents the width of the rectangle • height – a double that represents the height of the rectangle • color – a String that represents the color of the rectangle For the rectangle to be valid, the coordinates of all vertices must be between 0 and 1 inclusive. The color can be one of the following: “black”, “red”, “green”, “blue”. Rectangle constructor: The constructor should be: Rectangle (double x, double y, double height, double width, String color) Parameters are, in order, the x and y coordinates of the lower left corner, the height, the width, and the color of the rectangle. The constructor should check that these values represent a valid rectangle. If they do, then it should initialize the rectangle to these values. Otherwise, the date rectangle should be initialized to a rectangle of width 1, height 1, color “red” and lower left vertex at coordinates (0, 0). 2 Rectangle methods: Method Description double getX() Returns the x coordinate of this object. double getY() Returns the y coordinate of this object. double getWidth() Returns the width of this object. double getHeight() Returns the height of this object. String getColor() Returns the color of this object. void setX(double x) Sets the x coordinate of this object to x. If this results in an invalid rectangle, it leaves the x coordinate unchanged. void setY(double y) Sets the y coordinate of this object to y. If this results in an invalid rectangle, it leaves the y coordinate unchanged. void setWidth(double w) Sets the width of this object to w. If this results in an invalid rectangle, it leaves the width unchanged. void setHeight(double h) Sets the height of this object to h. If this results in an invalid rectangle, it leaves the height unchanged. void setColor (String c) Sets the color of this object to c. If c is invalid, it leaves the color coordinate unchanged. String toString() Returns a String representation of the rectangle containing the values of all instance variables. boolean equals(Rectangle rect) Returns true if rect represents the same rectangle as this object, and false otherwise. double computePerimeter() Computes and returns the perimeter of this rectangle double computeArea() Computes and returns the area of this rectangle boolean containsPoint(double x, double y) Returns true if this rectangle contains point (x, y) boolean containsRectangle(Rectangle rect) Returns true if this rectangle contains rectangle rect boolean intersects(Rectangle rect) Returns true if rectangle rect and this rectangle intersect. static boolean isValid(double x, double y, double w, double h) Static method. Returns true if the rectangle with lower left vertex at (x,y), width w and height h is valid, and false otherwise. void show() Extra Credit: Displays the rectangle on the screen. See details below. Make sure that the names of your methods match those listed above exactly, including capitalization. The number of parameters and their order must also match. You can change the parameter names if you want. All methods should be public.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
For this project you will have to write two classes.
Class Rectangle is a class that represents an axis-aligned rectangle. Class
RectangleTester is the driver class, used to test the Rectangle class.
Class Rectangle:
An instance of the class Rectangle represents a valid axis-aligned rectangle. It has several
instance variables that one needs to use to describe a rectangle, as well as methods that
operate on these variables.
Rectangle instance variables:
Class Rectangle will have the following instance variables:
• x – a double that represents the x coordinate of the lower left vertex of the
rectangle
• y – a double that represents the y coordinate of the lower left vertex of the
rectangle
• width – a double that represents the width of the rectangle
• height – a double that represents the height of the rectangle
• color – a String that represents the color of the rectangle
For the rectangle to be valid, the coordinates of all vertices must be between 0 and 1
inclusive. The color can be one of the following: “black”, “red”, “green”, “blue”.
Rectangle constructor:
The constructor should be: Rectangle (double x, double y, double height, double width, String color)
Parameters are, in order, the x and y coordinates of the lower left corner, the height, the
width, and the color of the rectangle. The constructor should check that these values
represent a valid rectangle. If they do, then it should initialize the rectangle to these values.
Otherwise, the date rectangle should be initialized to a rectangle of width 1, height 1, color
“red” and lower left vertex at coordinates (0, 0).
Class Rectangle is a class that represents an axis-aligned rectangle. Class
RectangleTester is the driver class, used to test the Rectangle class.
Class Rectangle:
An instance of the class Rectangle represents a valid axis-aligned rectangle. It has several
instance variables that one needs to use to describe a rectangle, as well as methods that
operate on these variables.
Rectangle instance variables:
Class Rectangle will have the following instance variables:
• x – a double that represents the x coordinate of the lower left vertex of the
rectangle
• y – a double that represents the y coordinate of the lower left vertex of the
rectangle
• width – a double that represents the width of the rectangle
• height – a double that represents the height of the rectangle
• color – a String that represents the color of the rectangle
For the rectangle to be valid, the coordinates of all vertices must be between 0 and 1
inclusive. The color can be one of the following: “black”, “red”, “green”, “blue”.
Rectangle constructor:
The constructor should be: Rectangle (double x, double y, double height, double width, String color)
Parameters are, in order, the x and y coordinates of the lower left corner, the height, the
width, and the color of the rectangle. The constructor should check that these values
represent a valid rectangle. If they do, then it should initialize the rectangle to these values.
Otherwise, the date rectangle should be initialized to a rectangle of width 1, height 1, color
“red” and lower left vertex at coordinates (0, 0).
2
Rectangle methods:
Method Description double getX() Returns the x coordinate of this object. double getY() Returns the y coordinate of this object. double getWidth() Returns the width of this object. double getHeight() Returns the height of this object. String getColor() Returns the color of this object. void setX(double x) Sets the x coordinate of this object to x. If this
results in an invalid rectangle, it leaves the x
coordinate unchanged. void setY(double y) Sets the y coordinate of this object to y. If this
results in an invalid rectangle, it leaves the y
coordinate unchanged. void setWidth(double w) Sets the width of this object to w. If this results
in an invalid rectangle, it leaves the width
unchanged. void setHeight(double h) Sets the height of this object to h. If this results
in an invalid rectangle, it leaves the height
unchanged. void setColor (String c) Sets the color of this object to c. If c is invalid,
it leaves the color coordinate unchanged. String toString() Returns a String representation of the rectangle
containing the values of all instance variables. boolean equals(Rectangle rect) Returns true if rect represents the same
rectangle as this object, and false otherwise. double computePerimeter() Computes and returns the perimeter of this
rectangle double computeArea() Computes and returns the area of this rectangle boolean containsPoint(double x,
double y) Returns true if this rectangle contains
point (x, y) boolean
containsRectangle(Rectangle rect) Returns true if this rectangle contains rectangle
rect boolean intersects(Rectangle
rect) Returns true if rectangle rect and this rectangle
intersect. static boolean isValid(double x,
double y, double w, double h) Static method. Returns true if the rectangle with
lower left vertex at (x,y), width w and height h
is valid, and false otherwise. void show() Extra Credit: Displays the rectangle on the
screen. See details below.
Make sure that the names of your methods match those listed above exactly, including
capitalization. The number of parameters and their order must also match. You can
change the parameter names if you want. All methods should be public.
Rectangle methods:
Method Description double getX() Returns the x coordinate of this object. double getY() Returns the y coordinate of this object. double getWidth() Returns the width of this object. double getHeight() Returns the height of this object. String getColor() Returns the color of this object. void setX(double x) Sets the x coordinate of this object to x. If this
results in an invalid rectangle, it leaves the x
coordinate unchanged. void setY(double y) Sets the y coordinate of this object to y. If this
results in an invalid rectangle, it leaves the y
coordinate unchanged. void setWidth(double w) Sets the width of this object to w. If this results
in an invalid rectangle, it leaves the width
unchanged. void setHeight(double h) Sets the height of this object to h. If this results
in an invalid rectangle, it leaves the height
unchanged. void setColor (String c) Sets the color of this object to c. If c is invalid,
it leaves the color coordinate unchanged. String toString() Returns a String representation of the rectangle
containing the values of all instance variables. boolean equals(Rectangle rect) Returns true if rect represents the same
rectangle as this object, and false otherwise. double computePerimeter() Computes and returns the perimeter of this
rectangle double computeArea() Computes and returns the area of this rectangle boolean containsPoint(double x,
double y) Returns true if this rectangle contains
point (x, y) boolean
containsRectangle(Rectangle rect) Returns true if this rectangle contains rectangle
rect boolean intersects(Rectangle
rect) Returns true if rectangle rect and this rectangle
intersect. static boolean isValid(double x,
double y, double w, double h) Static method. Returns true if the rectangle with
lower left vertex at (x,y), width w and height h
is valid, and false otherwise. void show() Extra Credit: Displays the rectangle on the
screen. See details below.
Make sure that the names of your methods match those listed above exactly, including
capitalization. The number of parameters and their order must also match. You can
change the parameter names if you want. All methods should be public.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
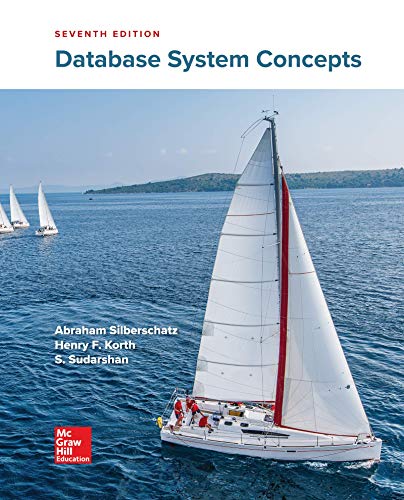
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
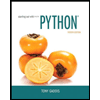
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
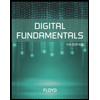
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
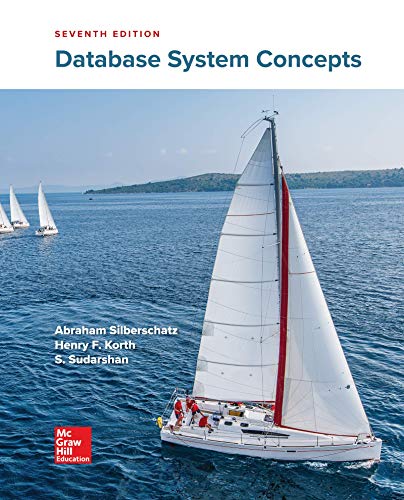
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
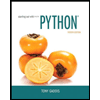
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
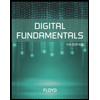
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
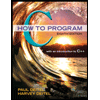
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
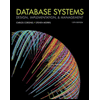
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
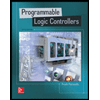
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education