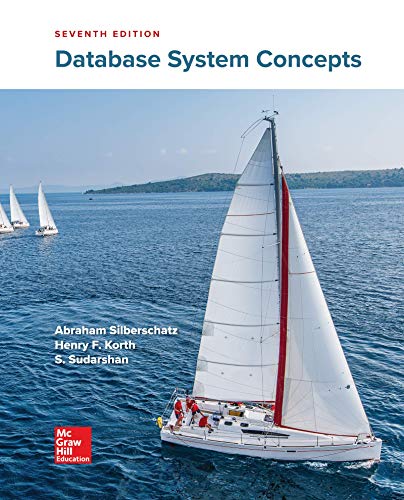
Concept explainers
Coding language: C++. (Try to avoid linked lists if possible please). Add comments. Thanks.
• Each functionality component must be implemented as a separate function, though the function does not need to be declared and defined separately
• No global variables are allowed
• No separate.hor.hpp are allowed
• You may not use any of the hash tables, or hashing functions provided by the STL or Boost library to implement your hash table
• Appropriate, informative messages must be provided for prompts and outputs
You must implement a hash table using the Double Hashing collision strategy and the mid-square base 10 hashing function with an R of 2. Your collision strategy must be implemented as a separate function, though it may be implemented inside your insert/search/delete functions, and should halt an insert / search/delete functions, and should halt an insert/search/delete after table size number of collisions. Your hash function must be implemented as a separate function. Your hash table must store positive integers and contain 20 buckets. No global declarations.
Functionality
- Your int main must have the menu allowing the user to choose between inserting, searching, deleting, and outputting the integers in your hash table. The user should be able to insert, search, delete, and output as many times as they wish. There should be an option to end the program. (Hint: enum/switch may be used).
- Insert a user specified integer
- If an insert is rejected due to the collision strategy halting, the function should output that the insert was rejected.
- Search for the first instance of a user specified integer
- If found, output that the integer exists upon finding the first instance of the integer.
- If the collision strategy halts, the function should output that the integer does not exist.
- No error checking required.
- Delete the first instance of a user specified integer
- If the desired integer is found, the function should tombstone the integer’s slot in the hash table, and report that the integer was deleted.
- If the collision strategy halts, the function should output that the integer does not exist.
- No error checking required
- Output all integers in the hash table starting at index 0 along with their index

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Question R .Full explain this question and text typing work only We should answer our question within 2 hours takes more time then we will reduce Rating Dont ignore this linearrow_forwardPythonarrow_forwardCreate an Ordered Doubly Linked List in C++. Remember that an ordered Linked List is one where inserts automatically place a new node so that all the nodes retain a certain order. This list should be templated where items should be in "ascending" order (i.e., if it is a list of numbers, it should be of order of least to greatest).arrow_forward
- Create Java Book objects using the following data and place them in a List. (Fields: Author, Title, Year, Price) Java Programming,Deitel,2016,68.75Python Programming,Gaddis,2018,78.56Data Analytics,Norman,2017,89.56Block Chain Development,Wilson,2019,87.45Web Development,Brown,2018,98.98 Create TWO List Comparators to sort the Book objects in ascending order: One by Book Title and the second by Book Year. Using each Comparator, SORT the List once by Title and again by Year. NOTE: You MUST use the Comparator to SORT. Cannot use any other built in sort methods. Output each SORTED List on Console in a format same as the data shown above (One Book per line, comma separated).arrow_forwardDiscuss the concept of dynamic sizing in arrays and linked lists. How does each data structure handle adding or removing elements while considering memory usage?arrow_forwardHow will this project be solved in Python? Thanks. There are example outputs in the picture.arrow_forward
- Write the lines of code to insert the key (book's ISBN) and value ("book") pair into "my_hash_table".arrow_forwarddef delete task (..., ...): I param: task_collection (list) holds all tasks; param: an integer ID indexes each task (stored as a dictionary) (str) - a string that is supposed to represent an integer ID that indexes the task in the list returns: 0 if the collection is empty. -1 - if the provided parameter is not a string or if it is not a string that contains a valid integer >=0 representing the task's position on the list. Otherwise, returns the item (dict) that was removed from the provided collection. 11 11 11arrow_forwardWhat is a hash table?a) A structure that maps values to keysb) A structure that maps keys to valuesc) A structure used for storaged) A structure used to implement stack and queuearrow_forward
- Just fix and organize the code below. Show screenshot code running! Write a menu-driven script that consolidates your code in 1 – 5. Define a function running each of them. However, you need to use a dictionary-based Jump Table to implement the menu Sample run: Assignment 5 Testing Script 1 Temperature Conversion and Mapping 2 Filtering Grades 3 Reducing Strings 4 Exit Just fix and organize the code below # our function temp_conversion def convert(): for i in range(len(cel)): cel[i] = (9 * cel[i] / 5 + 32) cel = [] number = int(input("How many elements you want to covert? : ")) for i in range(0, number): number = int(input("Enter the temperature in Celcius : ")) cel.append(number) print("The temperature in Celcius are", cel, "degrees") print("The temperature in Fahrenheit are", cel, "degrees") # our function filtering_grades def filtering_grades(): lst1 = [] lst2 = [] n = int(input("Enter number of elements : ")) for i in range(0, n):…arrow_forwardin C++ Write definition of search, isItemAtEqual, retrieve, remove, print, constructor, and destructor for class hashT based upon a method of your choice . Write a test program to test with at least 3 different hashing functions. No PLAGIARISM please . Thank you!arrow_forwardBuild an application that will use a hash table. In C++ using data structures.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
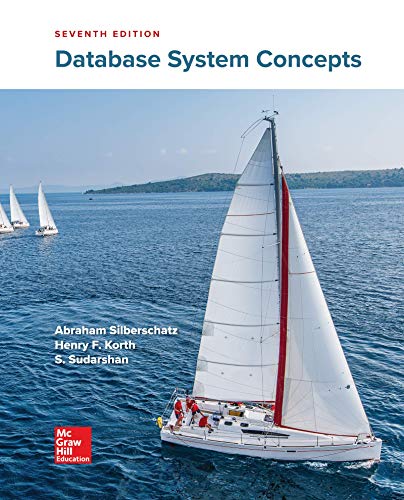
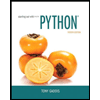
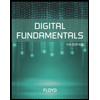
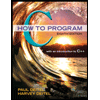
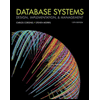
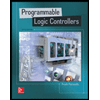