Code done in C Write a piece of code in a function called password that creates a file with a random password (it can be a number). In another function called check_password, the function must ask the user to input the password to login and it should match the password in the file. If it doesn't, it should keep asking the user to keep inputting the right password. Finally, just by passing in the two functions into the main, that would be fine. Ex. int main() { ....password(....); ....check_password(....); }
Code done in C Write a piece of code in a function called password that creates a file with a random password (it can be a number). In another function called check_password, the function must ask the user to input the password to login and it should match the password in the file. If it doesn't, it should keep asking the user to keep inputting the right password. Finally, just by passing in the two functions into the main, that would be fine. Ex. int main() { ....password(....); ....check_password(....); }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Code done in C
Write a piece of code in a function called password that creates a file with a random password (it can be a number). In another function called check_password, the function must ask the user to input the password to login and it should match the password in the file. If it doesn't, it should keep asking the user to keep inputting the right password. Finally, just by passing in the two functions into the main, that would be fine.
Ex.
int main()
{
....password(....);
....check_password(....);
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
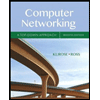
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
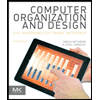
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
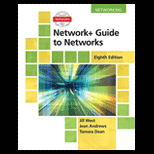
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
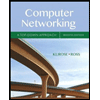
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
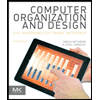
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
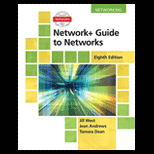
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
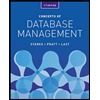
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
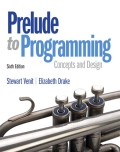
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
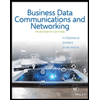
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY