Coding Assignment 3 1. The format and content of the output is not a suggestion –it is the specification given to you to follow so please follow it exactly. Points will be lost for not following the specification.Formatting the output to look exactly the same is part of exercises in this assignment.2. Please note that part of the rubric is how you coded the program in addition to outputting valid responses.Be sure to review the rubric before submitting your code.3.Make sure your name and student id are in a comment in the first line of your program.4.Name your program Code3_studentid.cand name the zip file Code3_studentid.zipfor submissionto Canvas.This assignment is about simulating a bingo game. If you are unfamiliar with how bingo is played, please see this YouTube link describing the game and how it is played.You will be expected to code your program’s behavior according to this version of BINGO.https://youtu.be/grzRsplu5acThis Wikipedia article describes the rules of the game and the layout of the cards. We will be using a 5x5 bingo card.https://en.wikipedia.org/wiki/Bingo_(American_version)PseudocodeCreate a 2D array that will be your bingo card.The bingo card will ONLY be 5x5.Make sure your random numbers are truly random between program runs.Createa function to fill the bingo card with random numbers in the proper ranges.Pass your 2D bingo array to this function to be populated.Be sure to mark the free spot.The ‘B’ columncontains numbers between 1 and 15The ‘I’ columncontains numbers between 16 and 30The ‘N’ columncontains numbers between 31 and 45The ‘G’ columncontains numbers between 46 and 60The ‘O’ columncontains numbers between 61 and 75Just a reminder -BINGO numbers MUST be unique. You cannot have the same number in multiple cells of your BINGO card. The rules of BINGO state that every number is unique.Obtaining random numbers using rand()does NOTguarantee that each number is unique –YOU must check that each number is unique. If a number has already been used, then get another number.Create a function to print the bingo cardto the screen.You must match the formatting shown in the sample output.The bingo array must be passed to this function. Create a function to pick a number that has not already been chosen.You will need to use a 1D array to keep track of which numbers between 1 and 75 have been used so that you don’t pick numbers that have already been called. If you randomly pick a number that was already called, then pick another. Continue this processuntil you find a number that has not been previously called.Print the number to thescreen along with its corresponding letter.This function should return that value.Create a function to determine if a called number exists in the player’s bingo card. Pass the bingo array and the number to the function. Loop over the array (nested for loops) and, if the number is found in the bingo array, then change the value to 0 to“mark” it. If the number is found, then this function should return true; otherwise, return false.Create a function to check for a completed row. A completed row is a row in the bingo array that has 0 for every value (we “marked” our bingo numbers by changing the existing valueto zero to indicate that the called number matches one inour bingo card).This function shouldcheck every row in the bingo array andreturn whether or not it found a completed row.Create a function to check for a completed column. A completed column is a column in the bingo array that has 0 for every value (we “marked” our bingo numbers by changing the existing value to zero to indicate that the called number matches one in our bingo card). This function should check every column in the bingo array and return whether or not it found a completed column.Add prototypes for thefunctions at the top of the program.main()Call function to fill bingo cardCall function to print bingo card to screenWhilethe player has not won and while there are still numbers to chose from (there are 75 total)Call a function to picka number that has not been chosen already–this function returns the called number.Show the player the number (including the B, I, N, G or O) and ask if the player has that number on their bingo card.If the player answers anything other than something that begins with ‘Y’, then reprint the bingo card and increment the count of numbers drawn so far.If the player answers something that begins with ‘Y’, thenCall a function to determine if the number drawn IS a number from the bingo card.If the function returns false, then print the message about cheating.If the function returns true,then call a functionto check for a completed row and a function to check for acompleted column.If a completed row and/or column is found, then the player has won anda message should print and the game should end.10% bonus –add another function to check for acompleteddiagonal.
Coding Assignment 3 1. The format and content of the output is not a suggestion –it is the specification given to you to follow so please follow it exactly. Points will be lost for not following the specification.Formatting the output to look exactly the same is part of exercises in this assignment.2. Please note that part of the rubric is how you coded the program in addition to outputting valid responses.Be sure to review the rubric before submitting your code.3.Make sure your name and student id are in a comment in the first line of your program.4.Name your program Code3_studentid.cand name the zip file Code3_studentid.zipfor submissionto Canvas.This assignment is about simulating a bingo game. If you are unfamiliar with how bingo is played, please see this YouTube link describing the game and how it is played.You will be expected to code your program’s behavior according to this version of BINGO.https://youtu.be/grzRsplu5acThis Wikipedia article describes the rules of the game and the layout of the cards. We will be using a 5x5 bingo card.https://en.wikipedia.org/wiki/Bingo_(American_version)PseudocodeCreate a 2D array that will be your bingo card.The bingo card will ONLY be 5x5.Make sure your random numbers are truly random between program runs.Createa function to fill the bingo card with random numbers in the proper ranges.Pass your 2D bingo array to this function to be populated.Be sure to mark the free spot.The ‘B’ columncontains numbers between 1 and 15The ‘I’ columncontains numbers between 16 and 30The ‘N’ columncontains numbers between 31 and 45The ‘G’ columncontains numbers between 46 and 60The ‘O’ columncontains numbers between 61 and 75Just a reminder -BINGO numbers MUST be unique. You cannot have the same number in multiple cells of your BINGO card. The rules of BINGO state that every number is unique.Obtaining random numbers using rand()does NOTguarantee that each number is unique –YOU must check that each number is unique. If a number has already been used, then get another number.Create a function to print the bingo cardto the screen.You must match the formatting shown in the sample output.The bingo array must be passed to this function. Create a function to pick a number that has not already been chosen.You will need to use a 1D array to keep track of which numbers between 1 and 75 have been used so that you don’t pick numbers that have already been called. If you randomly pick a number that was already called, then pick another. Continue this processuntil you find a number that has not been previously called.Print the number to thescreen along with its corresponding letter.This function should return that value.Create a function to determine if a called number exists in the player’s bingo card. Pass the bingo array and the number to the function. Loop over the array (nested for loops) and, if the number is found in the bingo array, then change the value to 0 to“mark” it. If the number is found, then this function should return true; otherwise, return false.Create a function to check for a completed row. A completed row is a row in the bingo array that has 0 for every value (we “marked” our bingo numbers by changing the existing valueto zero to indicate that the called number matches one inour bingo card).This function shouldcheck every row in the bingo array andreturn whether or not it found a completed row.Create a function to check for a completed column. A completed column is a column in the bingo array that has 0 for every value (we “marked” our bingo numbers by changing the existing value to zero to indicate that the called number matches one in our bingo card). This function should check every column in the bingo array and return whether or not it found a completed column.Add prototypes for thefunctions at the top of the program.main()Call function to fill bingo cardCall function to print bingo card to screenWhilethe player has not won and while there are still numbers to chose from (there are 75 total)Call a function to picka number that has not been chosen already–this function returns the called number.Show the player the number (including the B, I, N, G or O) and ask if the player has that number on their bingo card.If the player answers anything other than something that begins with ‘Y’, then reprint the bingo card and increment the count of numbers drawn so far.If the player answers something that begins with ‘Y’, thenCall a function to determine if the number drawn IS a number from the bingo card.If the function returns false, then print the message about cheating.If the function returns true,then call a functionto check for a completed row and a function to check for acompleted column.If a completed row and/or column is found, then the player has won anda message should print and the game should end.10% bonus –add another function to check for acompleteddiagonal.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Coding Assignment 3 1. The format and content of the output is not a suggestion –it is the specification given to you to follow so please follow it exactly. Points will be lost for not following the specification.Formatting the output to look exactly the same is part of exercises in this assignment.2. Please note that part of the rubric is how you coded the program in addition to outputting valid responses.Be sure to review the rubric before submitting your code.3.Make sure your name and student id are in a comment in the first line of your program.4.Name your program Code3_studentid.cand name the zip file Code3_studentid.zipfor submissionto Canvas.This assignment is about simulating a bingo game. If you are unfamiliar with how bingo is played, please see this YouTube link describing the game and how it is played.You will be expected to code your program’s behavior according to this version of BINGO.https://youtu.be/grzRsplu5acThis Wikipedia article describes the rules of the game and the layout of the cards. We will be using a 5x5 bingo card.https://en.wikipedia.org/wiki/Bingo_(American_version)PseudocodeCreate a 2D array that will be your bingo card.The bingo card will ONLY be 5x5.Make sure your random numbers are truly random between program runs.Createa function to fill the bingo card with random numbers in the proper ranges.Pass your 2D bingo array to this function to be populated.Be sure to mark the free spot.The ‘B’ columncontains numbers between 1 and 15The ‘I’ columncontains numbers between 16 and 30The ‘N’ columncontains numbers between 31 and 45The ‘G’ columncontains numbers between 46 and 60The ‘O’ columncontains numbers between 61 and 75Just a reminder -BINGO numbers MUST be unique. You cannot have the same number in multiple cells of your BINGO card. The rules of BINGO state that every number is unique.Obtaining random numbers using rand()does NOTguarantee that each number is unique –YOU must check that each number is unique. If a number has already been used, then get another number.Create a function to print the bingo cardto the screen.You must match the formatting shown in the sample output.The bingo array must be passed to this function.
Create a function to pick a number that has not already been chosen.You will need to use a 1D array to keep track of which numbers between 1 and 75 have been used so that you don’t pick numbers that have already been called. If you randomly pick a number that was already called, then pick another. Continue this processuntil you find a number that has not been previously called.Print the number to thescreen along with its corresponding letter.This function should return that value.Create a function to determine if a called number exists in the player’s bingo card. Pass the bingo array and the number to the function. Loop over the array (nested for loops) and, if the number is found in the bingo array, then change the value to 0 to“mark” it. If the number is found, then this function should return true; otherwise, return false.Create a function to check for a completed row. A completed row is a row in the bingo array that has 0 for every value (we “marked” our bingo numbers by changing the existing valueto zero to indicate that the called number matches one inour bingo card).This function shouldcheck every row in the bingo array andreturn whether or not it found a completed row.Create a function to check for a completed column. A completed column is a column in the bingo array that has 0 for every value (we “marked” our bingo numbers by changing the existing value to zero to indicate that the called number matches one in our bingo card). This function should check every column in the bingo array and return whether or not it found a completed column.Add prototypes for thefunctions at the top of the program.main()Call function to fill bingo cardCall function to print bingo card to screenWhilethe player has not won and while there are still numbers to chose from (there are 75 total)Call a function to picka number that has not been chosen already–this function returns the called number.Show the player the number (including the B, I, N, G or O) and ask if the player has that number on their bingo card.If the player answers anything other than something that begins with ‘Y’, then reprint the bingo card and increment the count of numbers drawn so far.If the player answers something that begins with ‘Y’, thenCall a function to determine if the number drawn IS a number from the bingo card.If the function returns false, then print the message about cheating.If the function returns true,then call a functionto check for a completed row and a function to check for acompleted column.If a completed row and/or column is found, then the player has won anda message should print and the game should end.10% bonus –add another function to check for acompleteddiagonal.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
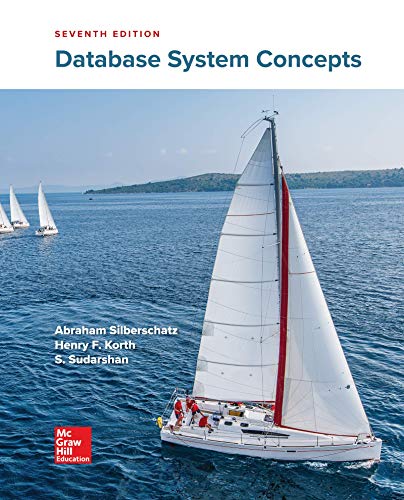
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
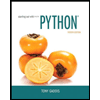
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
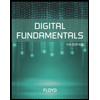
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
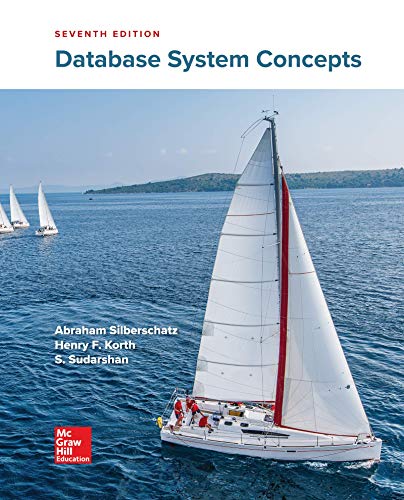
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
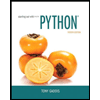
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
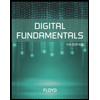
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
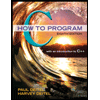
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
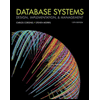
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
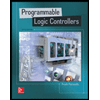
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education