Ro-Sham-Bo. Believe it or not, the classic game of Rock-PaperScissors has many other names. One of them is Ro-Sham-Bo. For this assignment, “Ro” will represent “Rock”, “Sham” will represent Paper, and “Bo” will represent Scissors. You will create a RoshamboPlayer class. It will have three attributes: PlayerName: String RoLimit: int ShamBoLimit: int It will also have an overloaded constructor that sets those three values. It will have two functions: playRound that takes in a string and returns a boolean value, and getName that takes in nothing and returns the PlayerName string. In your driver class for this assignment, you will create two RoshamboPlayer objects with the following values: p1: RoLimit == 30, ShamBoLimit == 60 p2: RoLimit = 40, ShamBoLimit = 85 You may name them whatever you like. You will prompt the user to choose one of these two to play against. Then you will create a loop that prompt the user to either play a round of Roshambo, or quit the game. If the user chooses to play, prompt them to attack with either “Ro”, “Sham”, or “Bo”. If the input is invalid, keep prompting them until they enter valid input. Once they’ve made a choice, pass the input to the RoshamboPlayer object’s playRound function. This function should randomly generate the RoshamboPlayer’s attack of “Ro”, “Sham”, or “Bo”. This is done by generating a random number between 0 and 100, then comparing it with the RoLimit and ShamboLimit values for the object. If the random value is between 0 and RoLimit, the object’s attack is “Ro”. If the random value is between RoLimit and ShamboLimit, the object’s attack is “Sham”. If the random value is greater than ShamboLimit, the object’s attack is “Bo”. Compare the object’s attack with the player’s attack. The playRound function should return TRUE if the player won the match, and FALSE if the object won or there was a draw (which is considered a win for the object). Use that information to inform the player if they won or lost. Repeat the process until the player quits.
Ro-Sham-Bo. Believe it or not, the classic game of Rock-PaperScissors has many other names. One of them is Ro-Sham-Bo. For this assignment, “Ro” will represent “Rock”, “Sham” will represent Paper, and “Bo” will represent Scissors.
You will create a RoshamboPlayer class. It will have three attributes:
- PlayerName: String
- RoLimit: int
- ShamBoLimit: int
It will also have an overloaded constructor that sets those three values. It will have two functions: playRound that takes in a string and returns a boolean value, and getName that takes in nothing and returns the PlayerName string.
In your driver class for this assignment, you will create two RoshamboPlayer objects with the following values:
- p1: RoLimit == 30, ShamBoLimit == 60
- p2: RoLimit = 40, ShamBoLimit = 85
You may name them whatever you like. You will prompt the user to choose one of these two to play against. Then you will create a loop that prompt the user to either play a round of Roshambo, or quit the game. If the user chooses to play, prompt them to attack with either “Ro”, “Sham”, or “Bo”. If the input is invalid, keep prompting them until they enter valid input.
Once they’ve made a choice, pass the input to the RoshamboPlayer object’s playRound function. This function should randomly generate the RoshamboPlayer’s attack of “Ro”, “Sham”, or “Bo”. This is done by generating a random number between 0 and 100, then comparing it with the RoLimit and ShamboLimit values for the object. If the random value is between 0 and RoLimit, the object’s attack is “Ro”. If the random value is between RoLimit and ShamboLimit, the object’s attack is “Sham”. If the random value is greater than ShamboLimit, the object’s attack is “Bo”. Compare the object’s attack with the player’s attack.
The playRound function should return TRUE if the player won the match, and FALSE if the object won or there was a draw (which is considered a win for the object). Use that information to inform the player if they won or lost. Repeat the process until the player quits.
Please see the sample output below.
![Sample Output:
[Ro-Sham-Bo Player]
Who do you want to face?
1) R. Dorothy
2) Johnny 5
Opponent: 1
Your opponent is R. Dorothy!
1) Play a round?
2) Quit?
Choice: 1
Enter an attack: Rock
Invalid attack!
Enter an attack: Sham
R. Dorothy chose Bo! You lose...
1) Play a round?
2) Quit?
Choice: 1
Enter an attack: Bo
R. Dorothy chose Sham! You win!
1) Play a round?
2) Quit?
Choice: 1
Enter an attack: Ro
R. Dorothy chose Ro! You lose...
1) Play a round?
2) Quit?
Choice: 2
Game Over](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F00eafb53-b95e-4692-845a-9178aef4b05e%2Ffc10ce60-6597-4bc5-93d7-0c22216d374f%2Fbrod89h_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 5 images

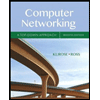
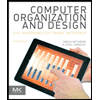
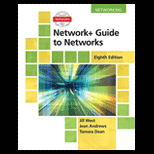
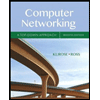
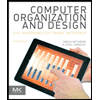
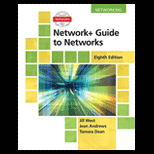
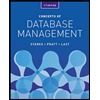
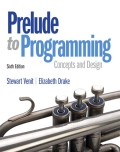
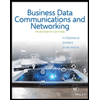