Complete the Course class by implementing the courseSize() method, which returns the total number of students in the course. Given classes: Class LabProgram contains the main method for testing the program. Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here.) Class Student represents a classroom student, which has three fields: first name, last name, and GPA. Ex. For the following students: Henry Bendel 3.6 Johnny Min 2.9 the output is: Course size: 2 public class LabProgram { public static void main (String [] args) { Course course = new Course(); // Example students for testing course.addStudent(new Student("Henry", "Bendel", 3.6)); course.addStudent(new Student("Johnny", "Min", 2.9)); System.out.println("Course size: " + course.courseSize()); } } public class Student { private String first; // first name private String last; // last name private double gpa; // grade point average // Student class constructor public Student(String first, String last, double gpa) { this.first = first; // first name this.last = last; // last name this.gpa = gpa; // grade point average } public double getGPA() { return gpa; } public String getLast() { return last; } } import java.util.ArrayList; public class Course { private ArrayList roster; //collection of Student objects public Course() { roster = new ArrayList(); } public int courseSize() { /* Your code goes here */ } public void addStudent(Student s) { roster.add(s); } }
Complete the Course class by implementing the courseSize() method, which returns the total number of students in the course.
Given classes:
- Class LabProgram contains the main method for testing the program.
- Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here.)
- Class Student represents a classroom student, which has three fields: first name, last name, and GPA.
Ex. For the following students:
Henry Bendel 3.6 Johnny Min 2.9
the output is:
Course size: 2
public class LabProgram {
public static void main (String [] args) {
Course course = new Course();
// Example students for testing
course.addStudent(new Student("Henry", "Bendel", 3.6));
course.addStudent(new Student("Johnny", "Min", 2.9));
System.out.println("Course size: " + course.courseSize());
}
}
public class Student {
private String first; // first name
private String last; // last name
private double gpa; // grade point average
// Student class constructor
public Student(String first, String last, double gpa) {
this.first = first; // first name
this.last = last; // last name
this.gpa = gpa; // grade point average
}
public double getGPA() {
return gpa;
}
public String getLast() {
return last;
}
}
import java.util.ArrayList;
public class Course {
private ArrayList<Student> roster; //collection of Student objects
public Course() {
roster = new ArrayList<Student>();
}
public int courseSize() {
/* Your code goes here */
}
public void addStudent(Student s) {
roster.add(s);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

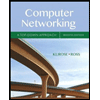
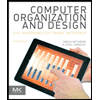
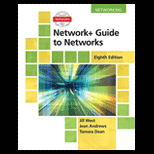
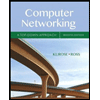
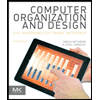
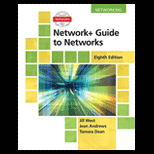
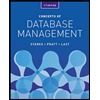
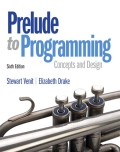
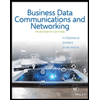