Complete the program by creating a CheckoutEquipment function that takes in the number of keys and vests currently available at the office. If there are enough keys and vests, the function should reduce the number of keys and vests by 1 and return true. Otherwise, the number of keys and vests will stay the same, and the function will return false. Take note that the number of keys and vests will be defined in the main function and the CheckoutEquipment function should modify the values of those variables declared in main.
Complete the program by creating a CheckoutEquipment function that takes in the number of keys and vests currently available at the office. If there are enough keys and vests, the function should reduce the number of keys and vests by 1 and return true. Otherwise, the number of keys and vests will stay the same, and the function will return false. Take note that the number of keys and vests will be defined in the main function and the CheckoutEquipment function should modify the values of those variables declared in main.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter11: Introduction To Classes
Section11.4: A Case Study: Constructing A Date Class
Problem 7E
Related questions
Question
- The food pantry provides its volunteers with keys to unlock doors and a Food Pantry vest to let customers know they work there. There are a limited amount of keys and vests, so volunteers need to check them out of the office.
Complete the program by creating a CheckoutEquipment function that takes in the number of keys and vests currently available at the office. If there are enough keys and vests, the function should reduce the number of keys and vests by 1 and return true. Otherwise, the number of keys and vests will stay the same, and the function will return false. Take note that the number of keys and vests will be defined in the main function and the CheckoutEquipment function should modify the values of those variables declared in main.
#include <iostream>
int main() {
int keys = 10;
int vests = 10;
bool checked_out = CheckoutEquipment(keys, vests);
if (checked_out) {
std::cout << "Successfully checked out.\n";
} else {
std::cout << "Not enough keys and vests.\n";
}
}
Expert Solution

Algorithm
- Start
- function "CheckoutEquipment" takes two arguments, "keys" and "vests", both of which are passed by reference (using the "&" operator). The purpose of the function is to check out a set of keys and a vest from the food pantry office, and to return a boolean value indicating whether the check-out was successful or not.
- The function starts by checking if both the "keys" and "vests" variables are greater than 0. If they are, then the function reduces the values of "keys" and "vests" by 1, and returns "true" to indicate that the check-out was successful.
- If either "keys" or "vests" are less than or equal to 0, the function returns "false" to indicate that the check-out was not successful.
- In the main function, two variables "keys" and "vests" are declared and initialized to 10, and then passed as arguments to the "CheckoutEquipment" function.
- The result of the function call is stored in a variable called "checked_out".
- The program then uses an if-else statement to output a message indicating whether the check-out was successful or not.
- Stop
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
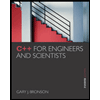
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
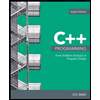
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
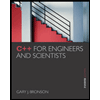
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
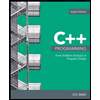
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning