fix the code to able to end the program when press "q". PLease Thank you so much
fix the code to able to end the program when press "q". PLease Thank you so much
#include <iostream>
#include <cmath>
class Currency {
protected:
int whole;
int fraction;
virtual std::string get_name() = 0;
public:
Currency() {
whole = 0;
fraction = 0;
}
Currency(double value) {
if (value < 0)
throw "Invalid value";
whole = int(value);
fraction = std::round(100 * (value - whole));
}
Currency(const Currency& curr) {
whole = curr.whole;
fraction = curr.fraction;
}
int get_whole() { return whole; }
int get_fraction() { return fraction; }
void set_whole(int w) {
if (w >= 0)
whole = w;
}
void set_fraction(int f) {
if (f >= 0 && f < 100)
fraction = f;
}
void add(const Currency* curr) {
whole += curr->whole;
fraction += curr->fraction;
if (fraction > 100) {
whole++;
fraction %= 100;
}
}
void subtract(const Currency* curr) {
if (!isGreater(*curr))
throw "Invalid Subtraction";
whole -= curr->whole;
if (fraction < curr->fraction) {
fraction = fraction + 100 - curr->fraction;
whole--;
}
else {
fraction -= curr->fraction;
}
}
bool isEqual(const Currency& curr) {
return curr.whole == whole && curr.fraction == fraction;
}
bool isGreater(const Currency& curr) {
if (whole < curr.whole)
return false;
if (whole == curr.whole && fraction < curr.fraction)
return false;
return true;
}
void print() {
std::cout << whole << "." << fraction << " " << get_name() << std::endl;
}
};
class Krone : public Currency {
protected:
std::string name = "Krone";
std::string get_name() {
return name;
}
public:
Krone() : Currency() { }
Krone(double value) : Currency(value) { }
Krone(Krone& curr) : Currency(curr) { }
};
class Soum : public Currency {
protected:
std::string name = "Soum";
std::string get_name() {
return name;
}
public:
Soum() : Currency() { }
Soum(double value) : Currency(value) { }
Soum(Krone& curr) : Currency(curr) { }
};
int main() {
Currency* currencies[2] = { new Soum(), new Krone() };
while (true) {
currencies[0]->print();
currencies[1]->print();
char oprr;
char oprd;
double value;
char curr[10];
std::cin >> oprr >> oprd >> value >> curr;
std::string currency(curr);
if (oprr == 'q') {
return 0;
}
try {
switch (oprr) {
case 'a':
if (oprd == 's' && currency == "Soum")
currencies[0]->add(new Soum(value));
else if (oprd == 'k' && currency == "Krone")
currencies[1]->add(new Krone(value));
else
throw "Invalid Addition";
break;
case 's':
if (oprd == 's' && currency == "Soum")
currencies[0]->subtract(new Soum(value));
else if (oprd == 'k' && currency == "Krone")
currencies[1]->subtract(new Krone(value));
else
throw "Invalid Subtraction";
break;
default:
throw "Invalid Operator";
}
}
catch (const char e[]) {
std::cout << e << std::endl;
}
}
}
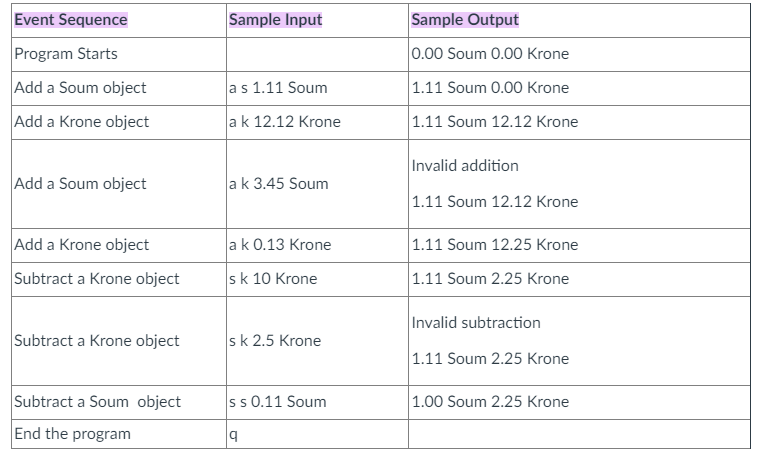

Step by step
Solved in 3 steps with 1 images

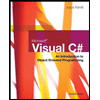
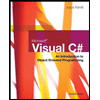