Composing Programs has introduced us to a way to implement an 00P system in programming languages that don't have it. Together with the current lesson, the OOP system can do the following: • Make a class Bind the self object to a class method • Instantiate objects of a class • Run an --init_() routine during instantiation of an object • Make a class inherit from a base class • Get its superclass from a class method Now, we are interested in augmenting our system to be able to compare two objects. Recognizing that (at least initially) no two objects from the same class are created, we can assign an ID to each object instantiated. This hashcode is used by some languages, such as Java, to implement the equality operator between objects. In this challenge, we will be emulating this in Python. We will be assigning each object with a hash based on a pseudorandom universally unique identifier (UUID).
Composing Programs has introduced us to a way to implement an 00P system in programming languages that don't have it. Together with the current lesson, the OOP system can do the following: • Make a class Bind the self object to a class method • Instantiate objects of a class • Run an --init_() routine during instantiation of an object • Make a class inherit from a base class • Get its superclass from a class method Now, we are interested in augmenting our system to be able to compare two objects. Recognizing that (at least initially) no two objects from the same class are created, we can assign an ID to each object instantiated. This hashcode is used by some languages, such as Java, to implement the equality operator between objects. In this challenge, we will be emulating this in Python. We will be assigning each object with a hash based on a pseudorandom universally unique identifier (UUID).
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 19RQ
Related questions
Question
Python OOP (Check pictures for instructions)
Code Template below:
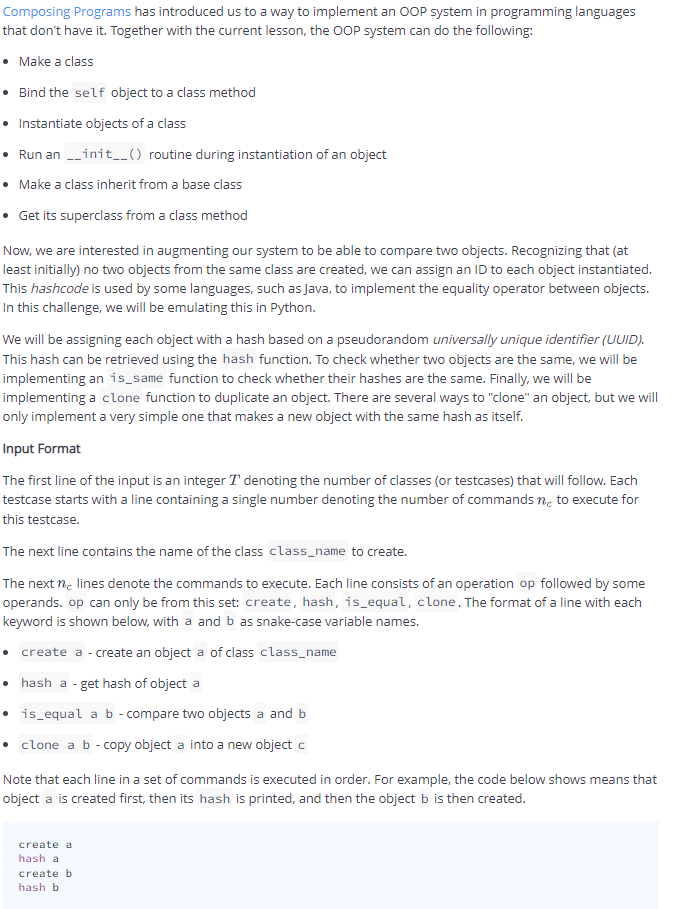
Transcribed Image Text:Composing Programs has introduced us to a way to implement an OOP system in programming languages
that don't have it. Together with the current lesson, the OOP system can do the following:
• Make a class
• Bind the self object to a class method
• Instantiate objects of a class
• Run an --init_() routine during instantiation of an object
• Make a class inherit from a base class
• Get its superclass from a class method
Now, we are interested in augmenting our system to be able to compare two objects. Recognizing that (at
least initially) no two objects from the same class are created, we can assign an ID to each object instantiated.
This hashcode is used by some languages, such as Java, to implement the equality operator between objects.
In this challenge, we will be emulating this in Python.
We will be assigning each object with a hash based on a pseudorandom universally unique identifier (UUID).
This hash can be retrieved using the hash function. To check whether two objects are the same, we will be
implementing an is_same function to check whether their hashes are the same. Finally, we will be
implementing a clone function to duplicate an object. There are several ways to "clone" an object, but we will
only implement a very simple one that makes a new object with the same hash as itself.
Input Format
The first line of the input is an integer T denoting the number of classes (or testcases) that will follow. Each
testcase starts with a line containing a single number denoting the number of commands ne to execute for
this testcase.
The next line contains the name of the class class_name to create.
The next ne lines denote the commands to execute. Each line consists of an operation op followed by some
operands. op can only be from this set: create, hash, is_equal, clone. The format of a line with each
keyword is shown below, with a and b as snake-case variable names.
create a - create an object a of class class_name
hash a - get hash of object a
• is_equal a b - compare two objects a and b
• clone a b - copy object a into a new object c
Note that each line in a set of commands is executed in order. For example, the code below shows means that
object a is created first, then its hash is printed, and then the object b is then created.
create a
hash a
create b
hash b
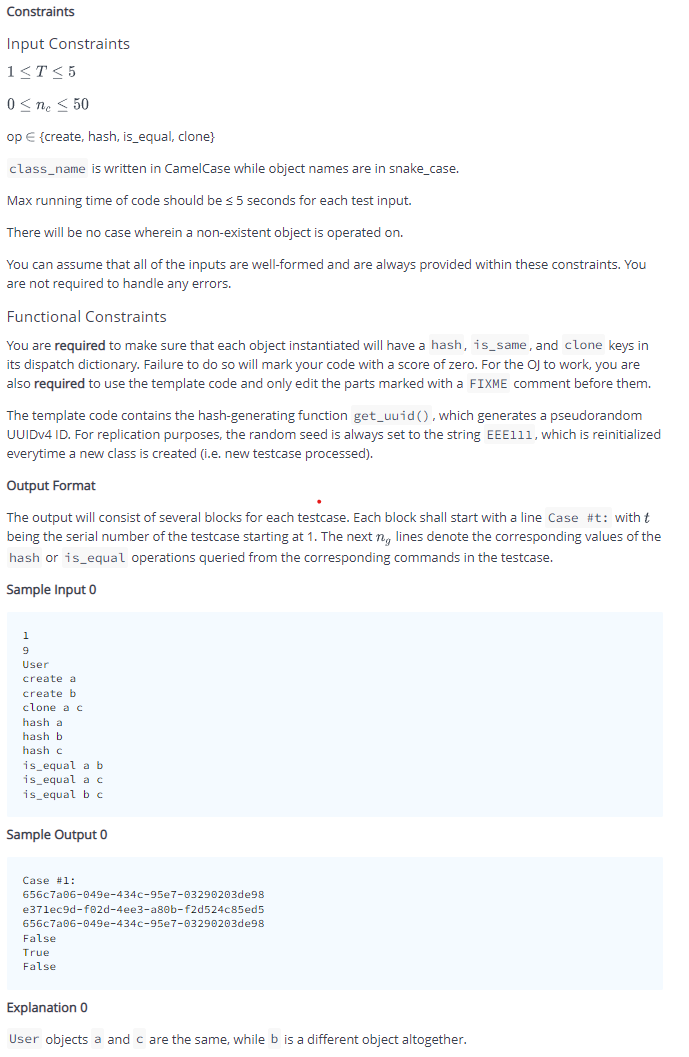
Transcribed Image Text:Constraints
Input Constraints
1<T< 5
0 < ne < 50
op E {create, hash, is_equal, clone}
class_name is written in CamelCase while object names are in snake_case.
Max running time of code should be <5 seconds for each test input.
There will be no case wherein a non-existent object is operated on.
You can assume that all of the inputs are well-formed and are always provided within these constraints. You
are not required to handle any errors.
Functional Constraints
You are required to make sure that each object instantiated will have a hash, is_same, and clone keys in
its dispatch dictionary. Failure to do so will mark your code with a score of zero. For the OJ to work, you are
also required to use the template code and only edit the parts marked with a FIXME comment before them.
The template code contains the hash-generating function get_uuid (), which generates a pseudorandom
UUIDV4 ID. For replication purposes, the random seed is always set to the string EEE111, which is reinitialized
everytime a new class is created (i.e. new testcase processed).
Output Format
The output will consist of several blocks for each testcase. Each block shall start with a line Case #t: with t
being the serial number of the testcase starting at 1. The next n, lines denote the corresponding values of the
hash or is_equal operations queried from the corresponding commands in the testcase.
Sample Input 0
User
create a
create b
clone a c
hash a
hash b
hash c
is_equal a b
is_equal a c
is_equal b c
Sample Output 0
Case #1:
656c7a06-049e-434c-95e7-03290203de98
e37lec9d-f02d-4ee3-a80b-f2d524c85ed5
656c7a06-049e-434c-95e7-03290203de98
False
True
False
Explanation 0
User objects a and c are the same, while b is a different object altogether.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
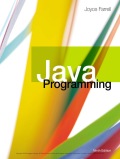
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
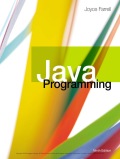
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT