USING JAVA, specify, design, and implement a class called statistician. After a statistician is initialized, it can be given a sequence of double numbers. Each number in the sequence is given to the statistician by activating a member function called next_number. For example, we can declare a statistician called s, and then give it the sequence of numbers 1.1, -2.4, 0.8 as shown here: statistician s;
USING JAVA,
specify, design, and implement a class called statistician. After a statistician is initialized, it can be given a sequence of double numbers. Each number in the sequence is given to the statistician by activating a member function called next_number. For example, we can declare a statistician called s, and then give it the sequence of numbers 1.1, -2.4, 0.8 as shown here:
statistician s;
s.next_number(1.1);
s.next_number(-2.4);
s.next_number(0.8);
After a sequence has been given to a statistician, there are various member funcitons to obtain infomration about the sequence. Include member functions that will provide the length of the sequence, the last number of the sequence, the sum of all the numbers in the sequence, the arithmetic mean of the numbers(i.e the sum of the numbers divided by the length of the sequence), the smallest number inthe sequence, and the largest number in the sequence. Notice that the length and sum functions can be called at any time, even if there are no enumbers in the sequence. In this case of an "empty" sequence, both length and sum will be zero. But the other member functions all have a precondition requiring that the sequence is non-empty.
You should also provide a member funciton that erases the sequene ( so that the statistician can start afresh with a new sequenece).
Notes: Do not try to store the entire sequence(because you don't know how long this sequence will be). Instead, just store the necessary information about the sequence: What is the sequence lenghth? What is the sum of the numbers in the sequence? What are the last, smallest and largest numbers? Each of these pieces of information can be stored in a private member variable that is updated whenever next_number is activated.
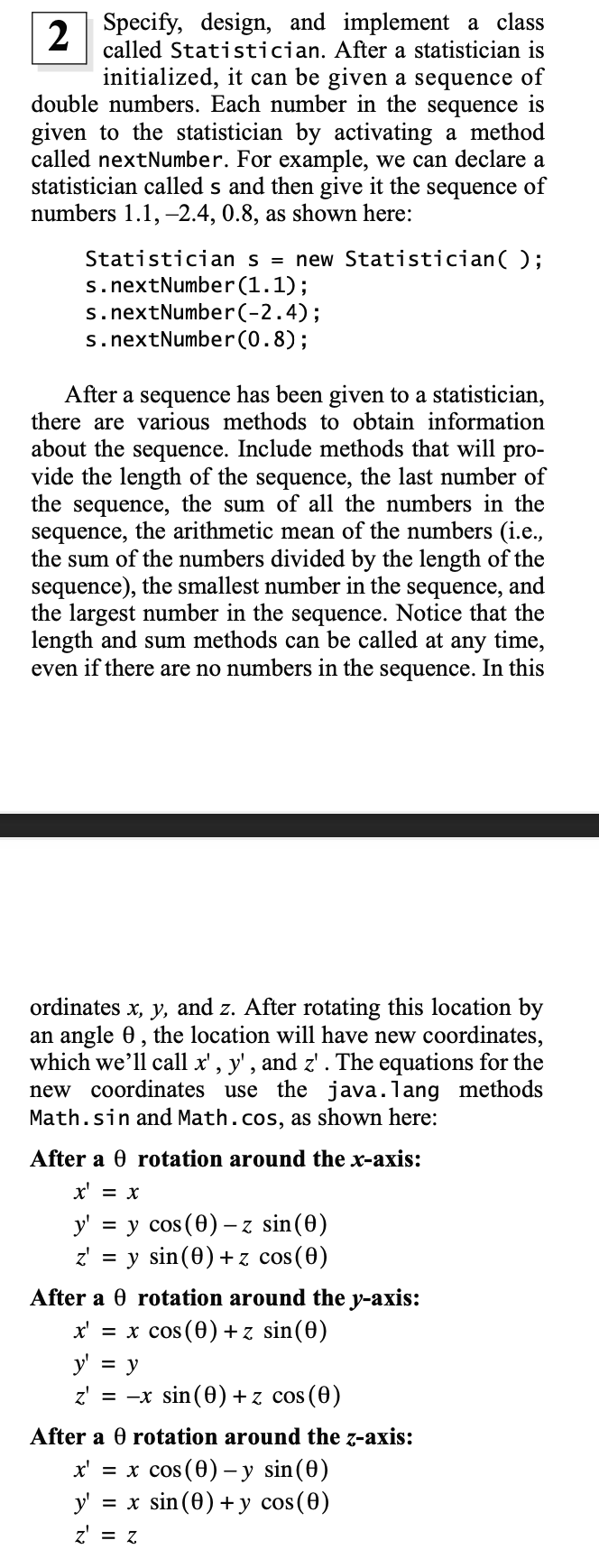

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

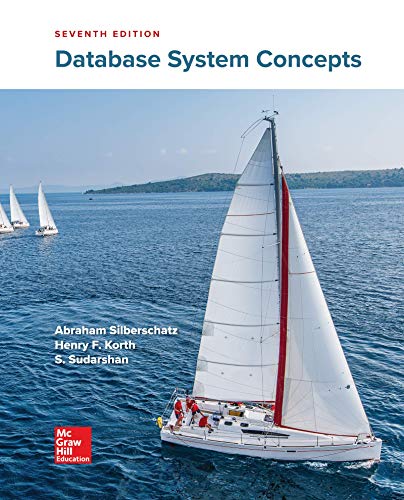
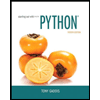
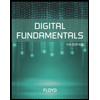
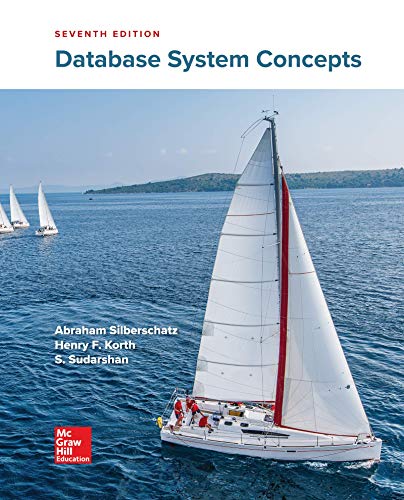
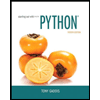
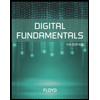
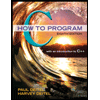
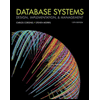
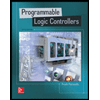