3. Go to the project05-01.js file in your code editor. Below the initial code at the top of the file declare the timeID variable but do not set an initial value. 4. Declare the questionList variable, storing in it the node list created by the querySelectorAll() method using “div#quiz input" as the CSS selector. 5. Add an onclick event handler to the startQuiz object, running an anonymous function that sets the class attribute of the overlay object to “showquiz" and repeats the countdown () function every 1 second (every 1000 milliseconds), storing the id of the timed command in the global timeID variable you declared in Step 3. 6. Create the countdown () function to update the quiz clock. Within the function create an if else statement that tests whether the value of the timeLeft variable is equal to 0. If it is equal to 0, do the following: a. Use the clearInterval() method to cancel the timed command with the variable timeID. b. Declare a variable named totalCorrect and set it equal to the value returned by the checkAnswers() function. c. If totalCorrect is equal to the length of the correctAnswers array then display an alert window congratulating the student on getting 100%, otherwise do the following: (i) Display an alert window indicating the number of incorrect answers out of the total number of questions on the quiz, (ii) change the value of the timeLeft variable to quizTime, (iii) set quizClock.value to the value of thetimeLeft variable, and (iv) change the class attribute of the overlay object to “hidequiz". 7. Otherwise, if the timeLeft variable is not equal 0, then: a. Decrease the value of timeLeft by 1. b. Set quickClock.value to the value of the timeLeft variable. 8. Save your changes to the file and then open project05-01.html in your web browser. 9. Run the quiz within the allotted time. Verify that the clock counts down the time remaining every second. At the end of the time limit, confirm that the quiz catches your mistakes and reports the number of correct answers out of the total number of questions. Verify that when you get all the answers correct, a congratulatory message is given. 1 "use strict"; 3 // Constants to set the time given for the quiz in seconds // and the correct answers to each quiz question const quizTime = 20; const correctAnswers = ["10", "4", "-6", "5", "-7"]; 4 7 // Elements in the quiz page let startQuiz = document.getElementById("startquiz"); let quizClock = document.getElementById("quizclock"); let overlay = document.getElementById("overlay"); 10 11 12 13 14 // Initialize the quiz time quizClock.value = quizTime; let timeleft = quizTime; 15 16 17 // Declare the ID for timed commands // and the node list for questions 18 19 20 let timeID; 21 let questionList = document.querySelectorAll("div#quiz input"); 22
3. Go to the project05-01.js file in your code editor. Below the initial code at the top of the file declare the timeID variable but do not set an initial value. 4. Declare the questionList variable, storing in it the node list created by the querySelectorAll() method using “div#quiz input" as the CSS selector. 5. Add an onclick event handler to the startQuiz object, running an anonymous function that sets the class attribute of the overlay object to “showquiz" and repeats the countdown () function every 1 second (every 1000 milliseconds), storing the id of the timed command in the global timeID variable you declared in Step 3. 6. Create the countdown () function to update the quiz clock. Within the function create an if else statement that tests whether the value of the timeLeft variable is equal to 0. If it is equal to 0, do the following: a. Use the clearInterval() method to cancel the timed command with the variable timeID. b. Declare a variable named totalCorrect and set it equal to the value returned by the checkAnswers() function. c. If totalCorrect is equal to the length of the correctAnswers array then display an alert window congratulating the student on getting 100%, otherwise do the following: (i) Display an alert window indicating the number of incorrect answers out of the total number of questions on the quiz, (ii) change the value of the timeLeft variable to quizTime, (iii) set quizClock.value to the value of thetimeLeft variable, and (iv) change the class attribute of the overlay object to “hidequiz". 7. Otherwise, if the timeLeft variable is not equal 0, then: a. Decrease the value of timeLeft by 1. b. Set quickClock.value to the value of the timeLeft variable. 8. Save your changes to the file and then open project05-01.html in your web browser. 9. Run the quiz within the allotted time. Verify that the clock counts down the time remaining every second. At the end of the time limit, confirm that the quiz catches your mistakes and reports the number of correct answers out of the total number of questions. Verify that when you get all the answers correct, a congratulatory message is given. 1 "use strict"; 3 // Constants to set the time given for the quiz in seconds // and the correct answers to each quiz question const quizTime = 20; const correctAnswers = ["10", "4", "-6", "5", "-7"]; 4 7 // Elements in the quiz page let startQuiz = document.getElementById("startquiz"); let quizClock = document.getElementById("quizclock"); let overlay = document.getElementById("overlay"); 10 11 12 13 14 // Initialize the quiz time quizClock.value = quizTime; let timeleft = quizTime; 15 16 17 // Declare the ID for timed commands // and the node list for questions 18 19 20 let timeID; 21 let questionList = document.querySelectorAll("div#quiz input"); 22
New Perspectives on HTML5, CSS3, and JavaScript
6th Edition
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Patrick M. Carey
Chapter7: Designing A Web Form: Creating A Survey Form
Section: Chapter Questions
Problem 15CP2
Related questions
Question
100%
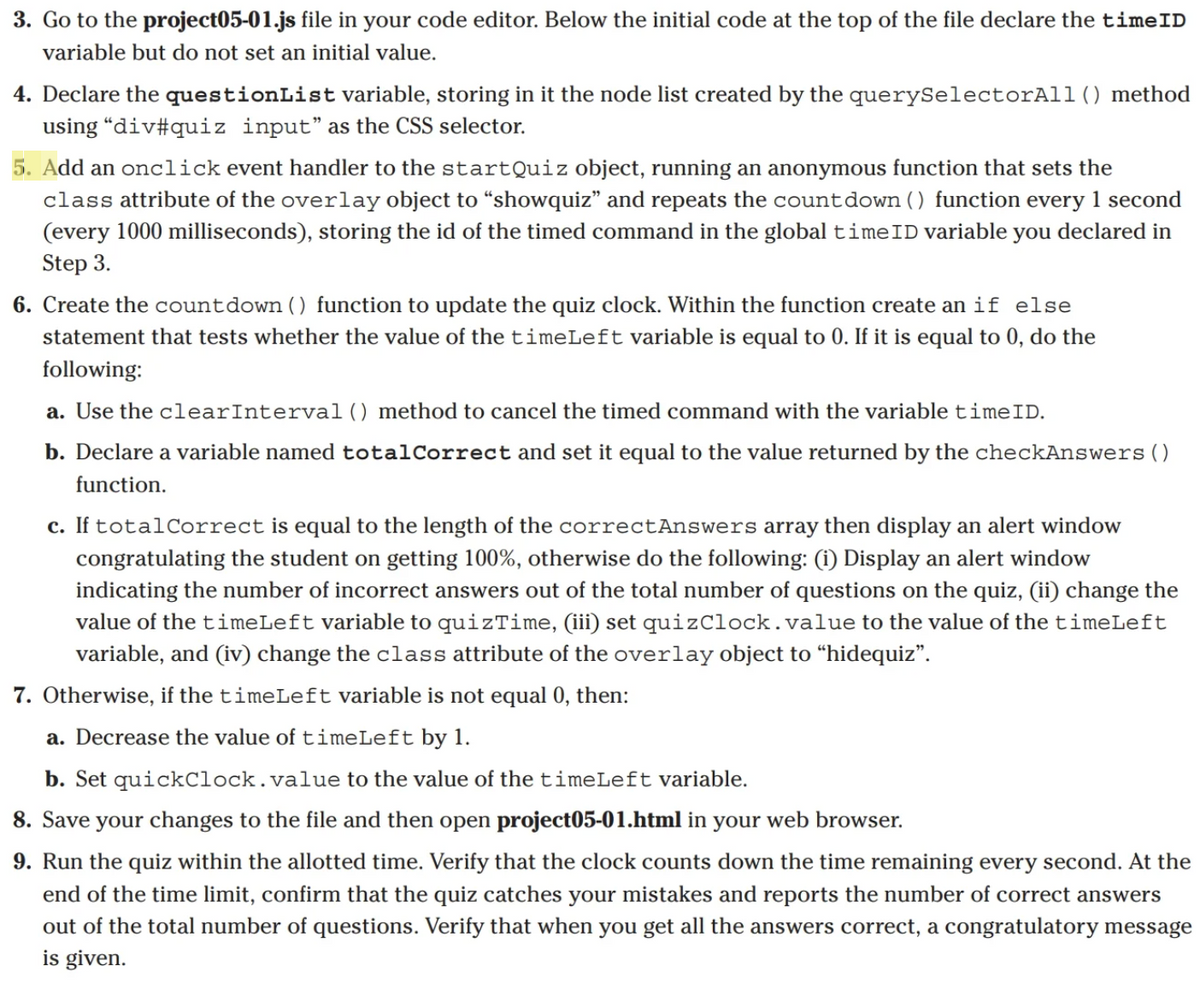
Transcribed Image Text:3. Go to the project05-01.js file in your code editor. Below the initial code at the top of the file declare the timeID
variable but do not set an initial value.
4. Declare the questionList variable, storing in it the node list created by the querySelectorAll() method
using “div#quiz input" as the CSS selector.
5. Add an onclick event handler to the startQuiz object, running an anonymous function that sets the
class attribute of the overlay object to “showquiz" and repeats the countdown () function every 1 second
(every 1000 milliseconds), storing the id of the timed command in the global timeID variable you declared in
Step 3.
6. Create the countdown () function to update the quiz clock. Within the function create an if else
statement that tests whether the value of the timeLeft variable is equal to 0. If it is equal to 0, do the
following:
a. Use the clearInterval() method to cancel the timed command with the variable timeID.
b. Declare a variable named totalCorrect and set it equal to the value returned by the checkAnswers()
function.
c. If totalCorrect is equal to the length of the correctAnswers array then display an alert window
congratulating the student on getting 100%, otherwise do the following: (i) Display an alert window
indicating the number of incorrect answers out of the total number of questions on the quiz, (ii) change the
value of the timeLeft variable to quizTime, (iii) set quizClock.value to the value of thetimeLeft
variable, and (iv) change the class attribute of the overlay object to “hidequiz".
7. Otherwise, if the timeLeft variable is not equal 0, then:
a. Decrease the value of timeLeft by 1.
b. Set quickClock.value to the value of the timeLeft variable.
8. Save your changes to the file and then open project05-01.html in your web browser.
9. Run the quiz within the allotted time. Verify that the clock counts down the time remaining every second. At the
end of the time limit, confirm that the quiz catches your mistakes and reports the number of correct answers
out of the total number of questions. Verify that when you get all the answers correct, a congratulatory message
is given.
![1
"use strict";
3
// Constants to set the time given for the quiz in seconds
// and the correct answers to each quiz question
const quizTime = 20;
const correctAnswers = ["10", "4", "-6", "5", "-7"];
4
7
// Elements in the quiz page
let startQuiz = document.getElementById("startquiz");
let quizClock = document.getElementById("quizclock");
let overlay = document.getElementById("overlay");
10
11
12
13
14
// Initialize the quiz time
quizClock.value = quizTime;
let timeleft = quizTime;
15
16
17
// Declare the ID for timed commands
// and the node list for questions
18
19
20
let timeID;
21
let questionList = document.querySelectorAll("div#quiz input");
22](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8f399ba7-b009-4644-8bcd-a13b6a8c4ed8%2F37d17bc1-96bb-408c-b0d2-a108109b3c3f%2F8h5a3g_processed.png&w=3840&q=75)
Transcribed Image Text:1
"use strict";
3
// Constants to set the time given for the quiz in seconds
// and the correct answers to each quiz question
const quizTime = 20;
const correctAnswers = ["10", "4", "-6", "5", "-7"];
4
7
// Elements in the quiz page
let startQuiz = document.getElementById("startquiz");
let quizClock = document.getElementById("quizclock");
let overlay = document.getElementById("overlay");
10
11
12
13
14
// Initialize the quiz time
quizClock.value = quizTime;
let timeleft = quizTime;
15
16
17
// Declare the ID for timed commands
// and the node list for questions
18
19
20
let timeID;
21
let questionList = document.querySelectorAll("div#quiz input");
22
Expert Solution

Step 1
project05-01_txt.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<title>Hands-on Project 5-1</title>
<link rel="stylesheet" href="styles.css" />
<script src="project05-01_txt.js" defer></script>
</head>
<body>
<header>
<h1>
Hands-on Project 5-1
</h1>
</header>
<article>
<h2>Algebra I Practice Quiz</h2>
<div id="container">
<div id="instructions">
<p>Click the <strong>Start Quiz</strong> button below
to start the quiz and begin the countdown. Upon completion, incorrect answers
will be marked in red. Retake the quiz as many times as you wish.
</p>
<input id="startquiz" value="Start Quiz" />
<input id="quizclock" />
</div>
<div id="quiz">
<table>
<tr>
<td>1) 5 + 2<em>x</em> = 25</td>
<td><em>x</em> = <input id="question1" /></td>
</tr>
<tr>
<td>2) 30 – 5<em>x</em> = 10</td>
<td><em>x</em> = <input id="question2" /></td>
</tr>
<tr>
<td>3) 6 + 3<em>x</em> = –12</td>
<td><em>x</em> = <input id="question3" /></td>
</tr>
<tr>
<td>4) 44 – 12<em>x</em> = –16</td>
<td><em>x</em> = <input id="question4" /></td>
</tr>
<tr>
<td>5) 48 + 6<em>x</em> = 6</td>
<td><em>x</em> = <input id="question5" /></td>
</tr>
</table>
</div>
<div id="overlay" class="hidequiz"></div>
</div>
</article>
</body>
</html>
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
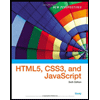
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
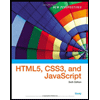
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L