Download the Java source code of a lexical analyzer (Main.Java). A summary of the source code is provided below. 1. The lexical analyzer program identifies a) alphanumeric lexemes (variables) as IDENT token, b) numeric token (constant integers) as INT_LIT token, and c) all other lexemes, such as ('+','','1', (), are identified as "UNKNOWN" category. The program uses a look-up table to retrieve the token value for these symbols. Any symbols other than these will throw an error. 2. The program reads characters of a line of code from an external text file, identifies and prints the lexemes and token numbers. 3. You must type one-page report highlighting your work and any additional features you may have added (for bonus points). Upload this report on DROPBOX. NO HANDWRITTEN items will be accepted. 4. Write a line of code in a text file as follows and provide the screenshot of lexical analyzer output in your report. You need to write no more than one-page report. (15) your_last_name] = ([last two digits of your T-number]+[your first name]) - yourFavoriteCity85yourFavoriteState] Example: smith = (65+ohn) - norfolk85ny 5. Modify the code in such a way that if it gets a character that is not in the lookup table, it prints an error message "Invalid lexeme, character not found." Test this scenario placing an example character in the text file such as '$' or '%' or '#'. Provide screenshots of your modified part of the code and the output. Hint: please add a case for the '=' sign. (5) 6. For bonus points, investigate ways to add new features to your program as follows. You may obtain up to 100% additional points as bonus. Provide screenshots of the modified part of the code along with the output. a. What if someone declares a variable starting with numerals (e.g., 8varX). Throw an error message suggesting that this is an illegal variable declaration. Can you detect a semicolon as the end of line (EOF)? b. c. Can you detect reserved lexemes and their tokens such as IF, WHILE, FOR, INT?
source code:
import java.util.*;
import java.io.*;
public class Main {
static File text = new File("/Users/.../Desktop/sourceCode.txt");
static FileInputStream keyboard;
static int charClass;
static char lexeme[] = new char[100]; //stores char of lexemes
static char nextChar;
static int lexLen;// length of lexeme
static int token;
static int nextToken;
// Token numbers
static final int LETTER = 0;
static final int DIGIT = 1;
static final int UNKNOWN = 99;
static final int INT_LIT = 10;
static final int IDENT = 11;
static final int ASSIGN_OP = 20;
static final int ADD_OP = 21;
static final int SUB_OP = 22;
static final int MULT_OP = 23;
static final int DIV_OP = 24;
static final int LEFT_PAREN = 25;
static final int RIGHT_PAREN = 26;
public static void main(String[] args) {
try{
keyboard = new FileInputStream(text);
getChar();
do {
lex();
}while (keyboard.available() > 0);
charClass = -1; // reset the character class
lex();
} catch(Exception e) {
System.out.println("Can not open file");
}
}
// Lookup table for non-alphanumeric characters
public static int lookup(char ch) {
switch (ch) {
case '(' :
addChar();
nextToken = LEFT_PAREN;
break;
case ')' :
addChar();
nextToken = RIGHT_PAREN;
break;
case '+' :
addChar();
nextToken = ADD_OP;
break;
case '-' :
addChar();
nextToken = SUB_OP;
break;
case '*' :
addChar();
nextToken = MULT_OP;
break;
case '/' :
addChar();
nextToken = DIV_OP;
break;
default:
addChar();
nextToken = -1;
break;
}
return nextToken;
}
public static void addChar() {
if (lexLen <= 98) { // a max length of 98
lexeme [lexLen++] = nextChar; // storing the char in lexeme array
lexeme [lexLen] = 0;//append lexeme with a zero
} else {
System.out.println("Error - lexeme is too long \n");
}
}
public static void getChar() throws IOException{
if ((nextChar = (char) keyboard.read()) != -1) {
if (Character.isLetter(nextChar))
charClass = LETTER;
else if (Character.isDigit(nextChar))
charClass = DIGIT;
else charClass = UNKNOWN;
}
else
charClass = -1;
}
public static void getNonBlank() throws IOException{
while (Character.isSpace(nextChar)) { //skip the white spaces
getChar();
}
}
public static int lex() throws IOException{
lexLen = 0;
getNonBlank();
switch (charClass) {
case LETTER:
addChar(); // add the char to lexeme array
getChar(); // get the next char
// Keep scanning for all characters in a variable/identifier
// until you get a non-alphanumeric character
while (charClass == LETTER || charClass == DIGIT) {
addChar();
getChar();
}
nextToken = IDENT; //spit the final token of the stored lexeme array
break;
case DIGIT:
addChar();
getChar();
while (charClass == DIGIT) {
addChar();
getChar();
}
nextToken = INT_LIT;//spit the final token of the stored lexeme array
break;
case UNKNOWN:
lookup(nextChar); //look up in the lookup table
getChar();
break;
case -1:
nextToken = -1;
lexeme[0] = 'E';
lexeme[1] = 'O';
lexeme[2] = 'F';
lexeme[3] = 0;
break;
}
System.out.printf("Next token is: %d, Next lexeme is ", nextToken);
for (char n: lexeme) {
if(n == 0) {
break;
}
System.out.print(n);
}
System.out.println();
return nextToken;
}
}
![Download the Java source code of a lexical analyzer (Main.Java). A summary of the source code
is provided below.
1. The lexical analyzer program identifies
a) alphanumeric lexemes (variables) as IDENT token,
b) numeric token (constant integers) as INT_LIT token, and
c) all other lexemes, such as ('+','','1', (), are identified as "UNKNOWN"
category. The program uses a look-up table to retrieve the token value for these
symbols. Any symbols other than these will throw an error.
2.
The program reads characters of a line of code from an external text file, identifies and
prints the lexemes and token numbers.
3. You must type one-page report highlighting your work and any additional features you
may have added (for bonus points). Upload this report on DROPBOX. NO HANDWRITTEN
items will be accepted.
4. Write a line of code in a text file as follows and provide the screenshot of lexical
analyzer output in your report. You need to write no more than one-page report. (15)
your_last_name] = ([last two digits of your T-number]+[your first name]) -
yourFavoriteCity85yourFavoriteState]
Example: smith = (65+ohn) - norfolk85ny
5. Modify the code in such a way that if it gets a character that is not in the lookup table, it
prints an error message "Invalid lexeme, character not found." Test this scenario placing
an example character in the text file such as '$' or '%' or '#'. Provide screenshots of your
modified part of the code and the output. Hint: please add a case for the '=' sign.
(5)
6. For bonus points, investigate ways to add new features to your program as follows. You
may obtain up to 100% additional points as bonus. Provide screenshots of the modified
part of the code along with the output.
a. What if someone declares a variable starting with numerals (e.g., 8varX). Throw
an error message suggesting that this is an illegal variable declaration.
Can you detect a semicolon as the end of line (EOF)?
b.
c. Can you detect reserved lexemes and their tokens such as IF, WHILE, FOR, INT?](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6c9872f4-07a5-438e-9711-8d7455ed4876%2F93a6ebc3-9c8b-47b7-a838-c854a8ab5ab3%2F1nsvjkk_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

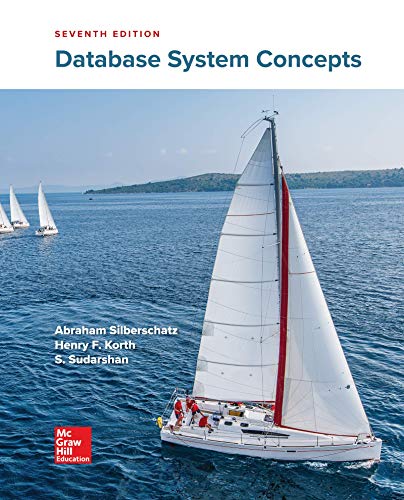
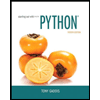
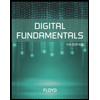
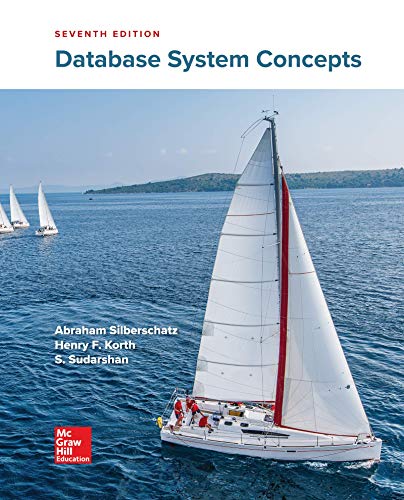
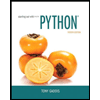
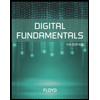
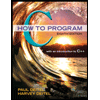
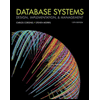
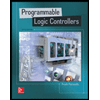