Consider a network of streets laid out in a rectangular grid, In a northeast path from one point in the grid to another, one may walk only to the north (up) and to the east (right). Write a program that must use a recursive function to count the number of northeast paths from one point to another in a rectangular grid. Your program should prompt the user to input the numbers of points to the north and to east respectively, and then output the total number of paths. Notes: 1. Here is a file (timer.h download and timer.cpp download) which should be included in your program to measure time in Window or Unix (includes Mac OS and Linux) systems (use start() for the beginning of the algorithm, stop() for the ending, and show() for printing). 2. The computing times of this algorithm is very high, and the number of paths may be overflow, don't try input numbers even over 16. 3. Name your recursive function prototype as calcPath(int north, int east) to ease grading.
Consider a network of streets laid out in a rectangular grid,
In a northeast path from one point in the grid to another, one may walk only to the north (up) and to the east (right).
Write a
Notes:
1. Here is a file (timer.h download and timer.cpp download) which should be included in your program to measure time in Window or Unix (includes Mac OS and Linux) systems (use start() for the beginning of the
2. The computing times of this algorithm is very high, and the number of paths may be overflow, don't try input numbers even over 16.
3. Name your recursive function prototype as calcPath(int north, int east) to ease grading.
4. Paste your output
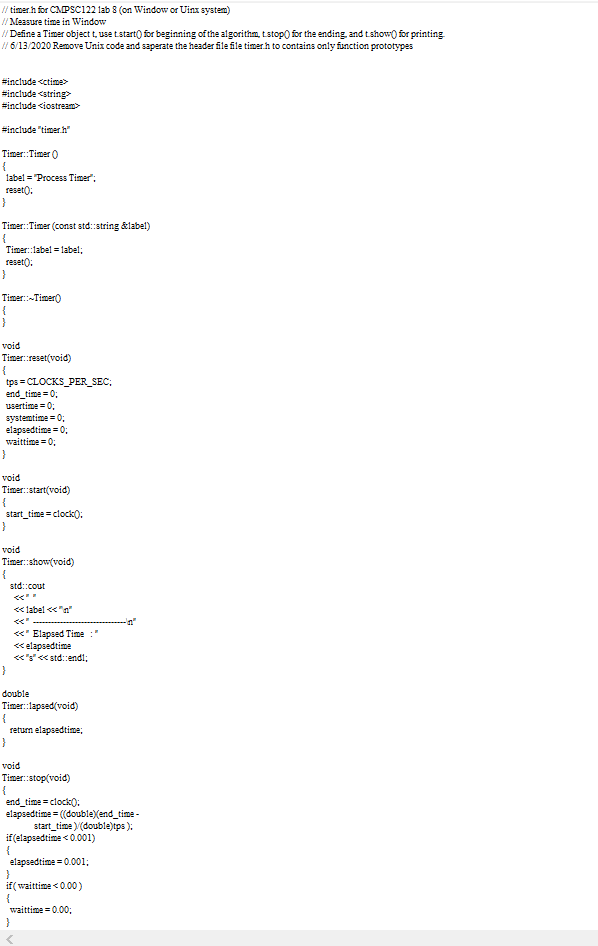
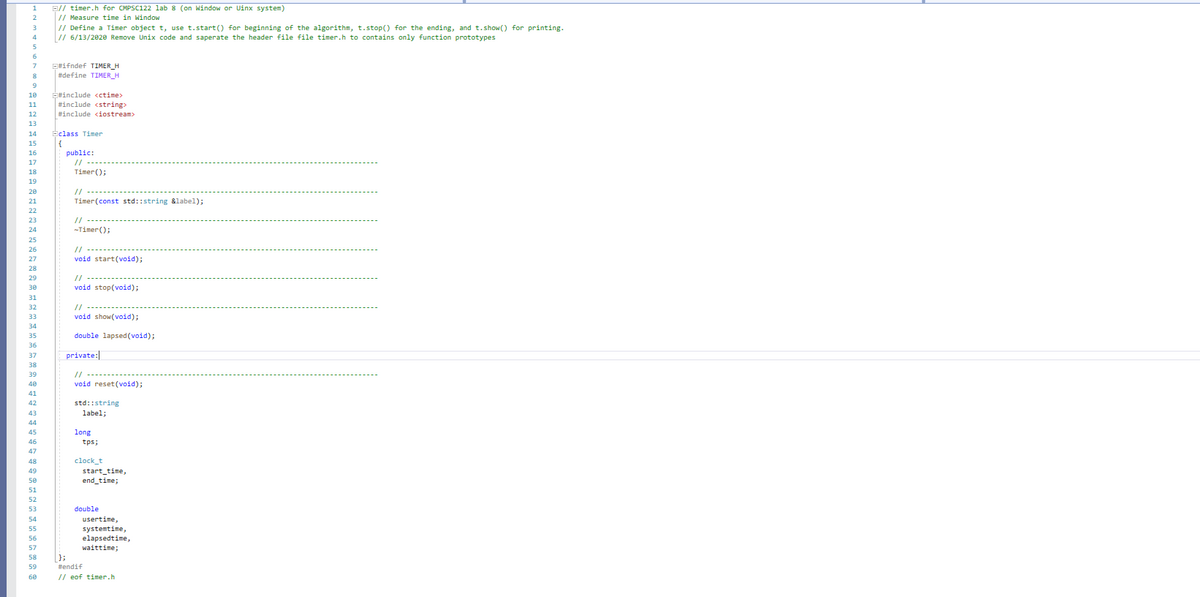

Trending now
This is a popular solution!
Step by step
Solved in 3 steps
