consumers for residential housing. Your task is to create an application to be used by the loan officers of the company when presenting loan options to its customers. The application will be a mortgage calculator that determines a monthly payment for loans. The company offers 10-, 15-, and 30-year fixed loans. The interest rates offered to customers are based on the customer’s credit score. Credit scores are classified into the following categories: Table 1: Credit Score Categories Rating Range Excellent 720-850 Good 690-719 Fair 630-689 Bad 300-629 The program should initially prompt the user (the loan officer) for the principle of the loan (i.e. the amount that is being borrowed). It should then ask him or her to enter the customer’s credit score. Based on the customer’s credit score, the program will randomly generate an interest rate based on the following ranges: Table 2: Interest Rate Assignments Rating Interest Rate Excellent 2.75% - 4.00% Good 4.01% - 6.50% Fair 6.51% - 8.75% Bad 8.76% - 10.50% The final input should be the number of years that the loan will be outstanding. Because the company only offers three different terms ( 10-, 15-, and 30-year loans), the program should ensure that no other terms are entered. Formulas Payment Calculator [Adapted from Wittwer, J.W., "Amortization Calculation," From Vertex42.com, Nov 11, 2008.] The formula to calculate the monthly payment for a fixed interest rate loan is as follows: where A = payment Amount per period P = initial Principal (loan amount) r = interest rate per period n = total number of payments or periods Example: What would the monthly payment be on a 15-year, $100,000 loan with a 4.50% annual interest rate? P = $100,000 r = 4.50% per year / 12 months = 0.375% (or 0.00375) per period n = 15 years * 12 months = 180 total periods A = 100,000 * (.00375 * (1 + .00375)180)/((1+.00375)180 – 1) Using these numbers in the formula above yields a monthly payment of $764.99. Start your program by prompting the user to enter a principle amount. The data type of this number should be a double. The amount must be positive and it must also be a numeric value. For example, when prompted to enter a number, if the user enters “abc,” the program will not be able to process the loan appropriately. Likewise, if the user enters -100000, the program will again produce erroneous results. Prompt the user to enter the customer’s credit score. Again, appropriate error checking is essential here. In addition to ensuring that values are numeric and positive, you must also ensure that the credit score entered does not exceed 850. If a non-numeric, negative, or score that exceeds 850 is entered, prompt the user to re-enter the score. If the credit score is below 300, display an error message to the user stating that the loan cannot be offered and exit the program. Make certain that the error message is displayed for a long enough duration for the user to read it before the program closes. Prompt the user to enter the term of the loan. Valid terms are 10, 15, and 30 years. Any other numbers should be rejected, and the user should be prompted to re-enter an appropriate value. Once the inputs have been entered and validated, your program must generate an interest rate to be used in the loan calculation. Create an enum to represent the possible credit ratings: EXCELLENT, GOOD, FAIR, and BAD. Assign the appropriate enum to the customer based on the ranges listed in Table 1 above. I recommend using if-statements for this. Using a SWITCH statement with the enum values as cases, assign an interest rate based on the customer’s credit rating. Within each case, use a random number generator to create an interest rate for each category based on the ranges listed in Table 2 above. Because the random number generator that you have been taught generates only integer values, you will need to generate a number in the hundreds and divide that number by 100 to get a result within the valid range for interest rates. Be careful with integer division here. Remember that an int divided by an int equals an int (i.e. int/int=int). To arrive at a double, you must make either the numerator or denominator a double to generate an interest rate with a decimal portion. To perform the calculation for a monthly payment, create a function called CalcPayment that receives the principle, interest rate, and number of years as parameters. The function should return the monthly payment that is calculated. Your program should check for invalid data such as non-numeric and non-positive entries for principle, credit score, and term. Use proper indentation and style, meaningful identifiers, and appropriate comments.
consumers for residential housing. Your task is to create an application to be used by the loan officers of the company when presenting loan options to its customers. The application will be a mortgage calculator that determines a monthly payment for loans.
The company offers 10-, 15-, and 30-year fixed loans. The interest rates offered to customers are based on the customer’s credit score. Credit scores are classified into the following categories:
Table 1: Credit Score Categories
Rating Range
Excellent 720-850
Good 690-719
Fair 630-689
Bad 300-629
The program should initially prompt the user (the loan officer) for the principle of the loan (i.e. the amount that is being borrowed). It should then ask him or her to enter the customer’s credit score. Based on the customer’s credit score, the program will randomly generate an interest rate based on the following ranges:
Table 2: Interest Rate Assignments
Rating Interest Rate
Excellent 2.75% - 4.00%
Good 4.01% - 6.50%
Fair 6.51% - 8.75%
Bad 8.76% - 10.50%
The final input should be the number of years that the loan will be outstanding. Because the company only offers three different terms ( 10-, 15-, and 30-year loans), the program should ensure that no other terms are entered.
Formulas
Payment Calculator
[Adapted from Wittwer, J.W., "Amortization Calculation," From Vertex42.com, Nov 11, 2008.]
The formula to calculate the monthly payment for a fixed interest rate loan is as follows:
where
A = payment Amount per period
P = initial Principal (loan amount)
r = interest rate per period
n = total number of payments or periods
Example: What would the monthly payment be on a 15-year, $100,000 loan with a 4.50% annual interest rate?
P = $100,000
r = 4.50% per year / 12 months = 0.375% (or 0.00375) per period
n = 15 years * 12 months = 180 total periods
A = 100,000 * (.00375 * (1 + .00375)180)/((1+.00375)180 – 1)
Using these numbers in the formula above yields a monthly payment of $764.99.
- Start your program by prompting the user to enter a principle amount. The data type of this number should be a double. The amount must be positive and it must also be a numeric value. For example, when prompted to enter a number, if the user enters “abc,” the program will not be able to process the loan appropriately. Likewise, if the user enters -100000, the program will again produce erroneous results.
- Prompt the user to enter the customer’s credit score. Again, appropriate error checking is essential here. In addition to ensuring that values are numeric and positive, you must also ensure that the credit score entered does not exceed 850. If a non-numeric, negative, or score that exceeds 850 is entered, prompt the user to re-enter the score.
If the credit score is below 300, display an error message to the user stating that the loan cannot be offered and exit the program. Make certain that the error message is displayed for a long enough duration for the user to read it before the program closes.
- Prompt the user to enter the term of the loan. Valid terms are 10, 15, and 30 years. Any other numbers should be rejected, and the user should be prompted to re-enter an appropriate value.
- Once the inputs have been entered and validated, your program must generate an interest rate to be used in the loan calculation.
- Create an enum to represent the possible credit ratings: EXCELLENT, GOOD, FAIR, and BAD.
- Assign the appropriate enum to the customer based on the ranges listed in Table 1 above. I recommend using if-statements for this.
- Using a SWITCH statement with the enum values as cases, assign an interest rate based on the customer’s credit rating.
- Within each case, use a random number generator to create an interest rate for each category based on the ranges listed in Table 2 above. Because the random number generator that you have been taught generates only integer values, you will need to generate a number in the hundreds and divide that number by 100 to get a result within the valid range for interest rates. Be careful with integer division here. Remember that an int divided by an int equals an int (i.e. int/int=int). To arrive at a double, you must make either the numerator or denominator a double to generate an interest rate with a decimal portion.
- To perform the calculation for a monthly payment, create a function called CalcPayment that receives the principle, interest rate, and number of years as parameters. The function should return the monthly payment that is calculated.
Your program should check for invalid data such as non-numeric and non-positive entries for principle, credit score, and term. Use proper indentation and style, meaningful identifiers, and appropriate comments.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 6 images

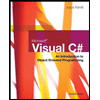
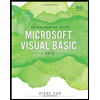
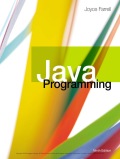
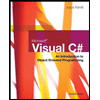
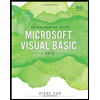
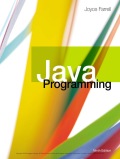