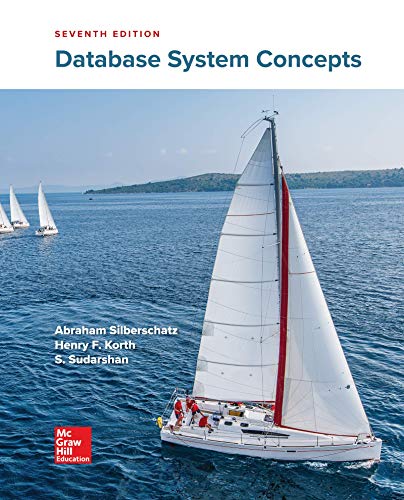
Concept explainers
convert or translate this Java Code to ( C
//import java util class
import java.util.*;
//import staement to import ThreadLocalRandom class
import java.util.concurrent.ThreadLocalRandom;
//main class
public class Main
{
//main method
public static void main(String[] args) {
//create a scanner class object
Scanner sc = new Scanner(System.in);
//declaring an integer variables
int userInput = 0, i;
// create an array with a size of 10 elements
int[] IntArray = new int[10];
//initiating random class
ThreadLocalRandom NumRandom = ThreadLocalRandom.current();
//using for loop to store the value in array created by random class
for(i = 0; i < 10; i++)
{
//assigning random values to array
IntArray[i] = NumRandom.nextInt(1, 31);
}
//Display output Message:
System.out.println("Please Enter your Choice");
/**while loop to continuos run the loop
***until user press 4.
***4 is to exit the program.*/
while(userInput != 4)
{
//propmt user to enter the choice
System.out.println("1: Enter 1 to see all array element.");
System.out.println("2: Enter 2 to see all elemnts of an array less than 25.");
System.out.println("3: Enter 3 to see all elemnts of an array greater than or equal to 25.");
System.out.println("4: Enter 4 to exit.");
//getting input choice of user
userInput = sc.nextInt();
//switch statement
switch(userInput)
{
//case 1 is used to display all the elements of the array.
case 1:
System.out.println("Random Generated Array's elements are: ");
for(i = 0; i < 10; i++)
{
//display the array elements
System.out.print(IntArray[i] + " ");
}
//for next line
System.out.println();
break;
//case 2 is used to display all the elements of an array less than 25.
case 2:
System.out.println("Display element of array less than 25");
for(i = 0; i < 10; i++)
{
if(IntArray[i] < 25)
//display the array elements
System.out.print(IntArray[i] + " ");
}
//for next line
System.out.println();
break;
//case 3 is used to display all the elements of an array greater than or equal to 25.
case 3:
System.out.println("Display element of array greater than or equal to 25");
for(i = 0; i < 10; i++)
{
if(IntArray[i] >= 25)
//display the array elements
System.out.print(IntArray[i] + " ");
}
//for next line
System.out.println();
break;
//end program
case 4:
break;
//when user not enter correct choice
default:
System.out.println("Please enter the correct choice.");
}
}
}
}

- The code is given in java programming language.
- The code given below is converted or translate to C programming.
#include <stdio.h>
#include<stdlib.h>
int main()
{
//int *IntArray= new int[10];
int arr[10],userInput=0;
for(int i=0;i<10;i++)
arr[i]=rand()%10;
printf("Please enter your choice\n");
while (userInput!=4){
printf("1: Enter 1 to see all the elements\n");
printf("2: Enter 2 to see all elemnts of an array less than 25.\n");
printf("3: Enter 3 to see all elemnts of an array greater than or equal to 25.\n");
printf("4: Enter 4 to exit.\n");
//getting input choice of user
scanf("%d",&userInput);
//switch statement
switch(userInput)
{
//case 1 is used to display all the elements of the array.
case 1:
printf("Random Generated Array's elements are: \n");
for(int i = 0; i < 10; i++)
{
//display the array elements
printf("%d",arr[i]);
printf(" ");
}
//for next line
printf("\n");
break;
//case 2 is used to display all the elements of an array less than 25.
case 2:
printf("Display element of array less than 25\n");
for(int i = 0; i < 10; i++)
{
if(arr[i] < 25)
//display the array elements
printf("%d",arr[i]);
printf(" ");
}
//for next line
printf("\n");
break;
//case 3 is used to display all the elements of an array greater than or equal to 25.
case 3:
printf("Display element of array greater than or equal to 25\n");
for(int i = 0; i < 10; i++)
{
if(arr[i] >= 25)
//display the array elements
printf("%d",arr[i]);
printf(" ");
}
//for next line
printf("\n");
break;
//end program
case 4:
break;
//when user not enter correct choice
default:
printf("Please enter the correct choice.\n");
}
}
//printf("%d",rand()%32);
return 0;
}
Step by stepSolved in 3 steps with 1 images

- 1 import java.util.Scanner; 2 3 public class Parsestrings { 4 public static void main(string[] args) { /* Type your code here. */ 5 6 return; 7 8arrow_forwardCreate the UML Diagram for this java code import java.util.Scanner; interface Positive{ void Number();} class Square implements Positive{ public void Number() { Scanner in=new Scanner(System.in); System.out.println("Enter a number: "); int a = in.nextInt(); if(a>0) { System.out.println("Positive number"); } else { System.out.println("Negative number"); } System.out.println("\nThe square of " + a +" is " + a*a); System.out.println("\nThe cubic of "+ a + " is "+ a*a*a); }} class Sum implements Positive{ public void Number() { Scanner in = new Scanner(System.in); System.out.println("\nEnter the value for a: "); int a = in.nextInt(); System.out.println("Enter the value for b" ); int b= in.nextInt(); System.out.printf("The Difference of two numbers: %d\n", a-b); System.out.printf("The…arrow_forwardJAVA What can I change to make it work?arrow_forward
- import java.util.Scanner; public class ParkingFinder {/* Your code goes here */ public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int numVisits; int duration; numVisits = scnr.nextInt(); duration = scnr.nextInt(); System.out.println(findParkingPrice(numVisits, duration)); }}arrow_forward1 import java.util.Scanner; 2 3 public class LabProgram { m & in 6780 4 5 7 } public static void main(String[] args) { /* Type your code here. */ }arrow_forwardC++. I have to make a constructor for a product. please please help me understand this, I will really appreciate this so much! I have to use this code to help make it i believe:arrow_forward
- Given string inputStr on one line and integers idx1 and idx2 on a second line, output "Match found" if the character at index idx1 of inputStr is equal to the character at index idx2. Otherwise, output "Match not found". End with a newline. Ex: If the input is: eerie 4 1 then the output is: Match found Note: Assume the length of string inputStr is greater than or equal to both idx1 and idx2.arrow_forwardimport java.util.Scanner; public class RomanNumerals { public static void main(String[] args) { Scanner in = new Scanner("I C X D M L"); char romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 1") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 100") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 10") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 500") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 1000") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 50") ; } /** Gives the value…arrow_forwardimport java.util.Scanner; public class CircleAndSphereWhileLoop{ public static final double MAX_RADIUS = 500.0; public static void main(String[] args) { Scanner in = new Scanner(System.in); // Step 2: Read a double value as radius using prompt // "Enter the radius (between 0.0 and 500.0, exclusive): " // Step 3: While the input radius is not in the ragne (0.0, 500.0) // Display a message on one line (ssuming input value -1) // "The input number -1.00 is out of range." // Read a double value as radius using the same promt double circumference = 2 * Math.PI * radius; double area = Math.PI * radius * radius; double surfaceArea = 4 * Math.PI * Math.pow(radius, 2); double volume = (4 / 3.0) * Math.PI * Math.pow(radius, 3); // Step 4: Display the radius, circle circumference, circle area, // sphere surface area, and…arrow_forward
- Using Java.arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forwardimport java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print(" "); String s1 = sc.nextLine(); System.out.print(""); String s2 = sc.nextLine(); int minLen = Math.min(s1.length(), s2.length()); int matchCount = 0; for (int i = 0; i < minLen; i++) { if (s1.charAt(i) == s2.charAt(i)) { matchCount++; } } if (matchCount == 1) { System.out.println("1 character matches"); } else { System.out.println(matchCount + " characters match"); } sc.close(); }}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
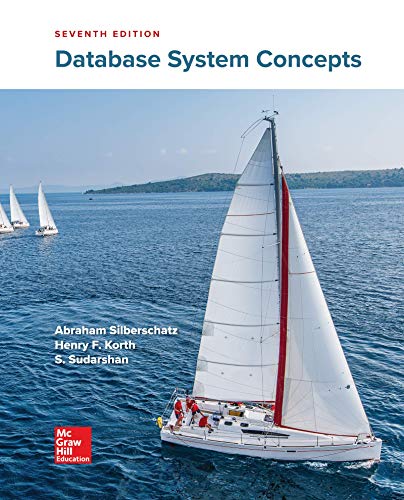
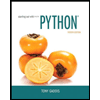
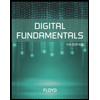
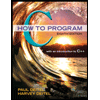
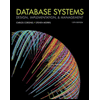
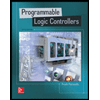