CONVERT THIS PYTHON CODE TO JAVA class Vehicle(): def __init__(self, color, wheels): self.color = color self.wheels = wheels def __str__(self): return "color {}, {} wheels".format( self.color, self.wheels ) class Car(Vehicle): def __init__(self, color, wheels, speed, displacement): Vehicle.__init__(self, color, wheels) self.speed = speed self.displacement = displacement def __str__(self): return Vehicle.__str__(self) + ", {} km/h, {} cc".format( self.speed, self.displacement) class Truck(Car): def __init__(self, color, wheels, speed, displacement, load): Car.__init__(self,color, wheels, speed, displacement) self.load = load def __str__(self): return Car.__str__(self) + ", {} load kg".format(self.load) class Bike(Vehicle): def __init__(self, color, wheels, type): Vehicle.__init__(self,color, wheels) self.type = type def __str__(self): return Vehicle.__str__(self) + ", {}".format(self.type) class Motorcycle(Bike): def __init__(self, color, wheels, type, speed, displacement): Bike.__init__(self,color, wheels, type) self.speed = speed self.displacement = displacement def __str__(self): return Bike.__str__(self) + ", {} km/h, {} cc".format( self.speed, self.displacement) def catalog(vehicles, wheels=None): # First pass, show count if wheels != None: counter = 0 for v in vehicles: if v.wheels == wheels: counter += 1 print("\n{} vehicles with {} wheels have been found:".format( counter, wheels)) # Second pass, show vehicles for v in vehicles: if wheels == None: print(v) else: if v.wheels == wheels: print(v)
CONVERT THIS PYTHON CODE TO JAVA
class Vehicle():
def __init__(self, color, wheels):
self.color = color
self.wheels = wheels
def __str__(self):
return "color {}, {} wheels".format( self.color, self.wheels )
class Car(Vehicle):
def __init__(self, color, wheels, speed, displacement):
Vehicle.__init__(self, color, wheels)
self.speed = speed
self.displacement = displacement
def __str__(self):
return Vehicle.__str__(self) + ", {} km/h, {} cc".format(
self.speed, self.displacement)
class Truck(Car):
def __init__(self, color, wheels, speed, displacement, load):
Car.__init__(self,color, wheels, speed, displacement)
self.load = load
def __str__(self):
return Car.__str__(self) + ", {} load kg".format(self.load)
class Bike(Vehicle):
def __init__(self, color, wheels, type):
Vehicle.__init__(self,color, wheels)
self.type = type
def __str__(self):
return Vehicle.__str__(self) + ", {}".format(self.type)
class Motorcycle(Bike):
def __init__(self, color, wheels, type, speed, displacement):
Bike.__init__(self,color, wheels, type)
self.speed = speed
self.displacement = displacement
def __str__(self):
return Bike.__str__(self) + ", {} km/h, {} cc".format(
self.speed, self.displacement)
def catalog(vehicles, wheels=None):
# First pass, show count
if wheels != None:
counter = 0
for v in vehicles:
if v.wheels == wheels:
counter += 1
print("\n{} vehicles with {} wheels have been found:".format(
counter, wheels))
# Second pass, show vehicles
for v in vehicles:
if wheels == None:
print(v)
else:
if v.wheels == wheels:
print(v)
list = [
Car("blue", 0, 150, 1200),
Van("white", 4, 100, 1300, 1500),
Bicycle("green", 2, "urban"),
Motorcycle("black", 2, "sport", 180, 900)
]
catalog(list)
catalog(list, 0)
catalog(list, 2)
catalog(list, 4)
output
blue color, 0 wheels, 150 km/h, 1200 cc
white color, 4 wheels, 100 km/h, 1300 cc, 1500 kg load
green color, 2 wheels, urban
black color, 2 wheels, sports, 180 km/h, 900 cc
Found 1 vehicles with 0 wheels:
blue color, 0 wheels, 150 km/h, 1200 cc
2 vehicles with 2 wheels have been found:
green color, 2 wheels, urban
black color, 2 wheels, sports, 180 km/h, 900 cc
Found 1 vehicles with 4 wheels:
white color, 4 wheels, 100 km/h, 1300 cc, 1500 kg load

Step by step
Solved in 2 steps

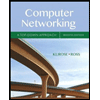
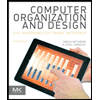
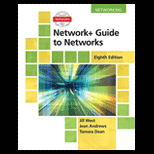
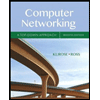
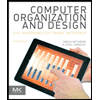
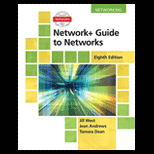
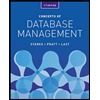
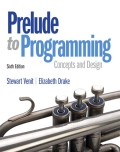
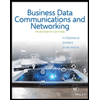