(Converting a Number from Binary to Decimal) The language of a computer, called machine language, is a sequence of 0s and 1s. When you press the key A on the keyboard, 01000001 is stored in the computer. Also, the collating sequence of A in the ASCII character set is 65. In fact, the binary representation of A is 01000001 and the decimal representation of A is 65. The numbering system we use is called the decimal system, or base 10 system. The numbering system that the computer uses is called the binary system, or base 2 system. The purpose of this exercise is to write a function to convert a number from base 2 to base 10. To convert a number from base 2 to base 10, we first find the weight of each bit in the binary number. The weight of each bit in the binary number is assigned from right to left. The weight of the rightmost bit is 0. The weight of the bit immediately to the left of the rightmost bit is 1, the weight of the bit immediately to the left of it is 2, and so on. Consider the binary number 1001101. The weight of each bit is as follows: weight 6 5 4 3 2 1 0 1 0 0 1 1 0 1 We use the weight of each bit to find the equivalent decimal number. For each bit, we multiply the bit by 2 to the power of its weight, and then we add all of the numbers. For the binary number 1001101, the equivalent decimal number is 1 X 2^6 + 0 X 2^5 + 0 X 2^4 + 1 X 2^3 + 1 X 2^2 + 0 X 2^1 + 1 X 2^0 = 64 + 0 + 0 + 8 + 4 + 0 + 1 = 77 To write a program that converts a binary number into the equivalent decimal number, we note two things: (1) The weight of each bit in the binary number must be known, and (2) the weight is assigned from right to left. Because we do not know in advance how many bits are in the binary number, we must process the bits from right to left. After processing a bit, we can add 1 to its weight, giving the weight of the bit immediately to its left. Also, each bit must be extracted from the binary number and multiplied by 2 to the power of its weight. To extract a bit, you can use the mod operator. Write a program that uses a stack to convert a binary number into an equivalent decimal number and test your function for the following values:
(Converting a Number from Binary to Decimal) The language of a computer, called machine language, is a sequence of 0s and 1s.
When you press the key A on the keyboard, 01000001 is stored in the computer.
Also, the collating sequence of A in the ASCII character set is 65.
In fact, the binary representation of A is 01000001 and the decimal representation of A is 65.
The numbering system we use is called the decimal system, or base 10 system.
The numbering system that the computer uses is called the binary system, or base 2 system. The purpose of this exercise is to write a function to convert a number from base 2 to base 10.
To convert a number from base 2 to base 10, we first find the weight of each bit in the binary number.
The weight of each bit in the binary number is assigned from right to left.
The weight of the rightmost bit is 0.
The weight of the bit immediately to the left of the rightmost bit is 1, the weight of the bit immediately to the left of it is 2, and so on.
Consider the binary number 1001101.
The weight of each bit is as follows:
weight 6 5 4 3 2 1 0
1 0 0 1 1 0 1
We use the weight of each bit to find the equivalent decimal number.
For each bit, we multiply the bit by 2 to the power of its weight, and then we add all of the numbers.
For the binary number 1001101, the equivalent decimal number is
1 X 2^6 + 0 X 2^5 + 0 X 2^4 + 1 X 2^3 + 1 X 2^2 + 0 X 2^1 + 1 X 2^0
= 64 + 0 + 0 + 8 + 4 + 0 + 1 = 77
To write a program that converts a binary number into the equivalent decimal number, we note two things:
(1) The weight of each bit in the binary number must be known, and
(2) the weight is assigned from right to left.
Because we do not know in advance how many bits are in the binary number, we must process the bits from right to left.
After processing a bit, we can add 1 to its weight, giving the weight of the bit immediately to its left.
Also, each bit must be extracted from the binary number and multiplied by 2 to the power of its weight.
To extract a bit, you can use the mod operator.
Write a program that uses a stack to convert a binary number into an equivalent decimal number and test your function for the following values:
11000101, 10101010, 11111111, 10000000, 1111100000.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

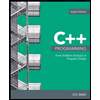
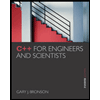
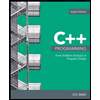
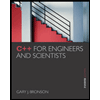