C++(OOP) Write a program that implements the following situation. A marketing organization ABC Ltd helps to promote the sales of other brands and products. ABC Ltd has permanent employees and also hires employees from outside as well for different occasions on contract basis. A permanent employee gets monthly salary whereas contractual employee gets paid on daily wage rate along with basic salary. You need to create a class Employee with following data members. • String name • Int age • Int contact • Int CNIC • String education You will inherit the Employee class into Permanent_EMP and Contractual_EMP classes. In Permanent_EMP you are required to create following data members • Int basic_salary • Int allowances In addition, you are required to write down following class functions • A constructor that takes values of salary & allowances at the time of object declaration. • A method that calculates and returns the salary with following formula o Salary = basic_salary + allowances. In Contractual_EMP class you are required to create following data members • Int basic_salary • Int commission_percentage In addition, you are required to write down following class functions • A constructor that takes values of basic_salary & commission_percentage at the time of object declaration. • A method that calculates and returns the salary with following formula o Salary = basic_salary + (basic_salary* commission_percentage/100) In main you are required to create one object of Permanent_EMP and one object of Contractual_EMP and implement the above given concept by using dynamic polymorphism.
C++(OOP)
Write a program that implements the following situation.
A marketing organization ABC Ltd helps to promote the sales of other brands and products. ABC
Ltd has permanent employees and also hires employees from outside as well for different occasions
on contract basis. A permanent employee gets monthly salary whereas contractual employee gets
paid on daily wage rate along with basic salary.
You need to create a class Employee with following data members.
• String name
• Int age
• Int contact
• Int CNIC
• String education
You will inherit the Employee class into Permanent_EMP and Contractual_EMP classes.
In Permanent_EMP you are required to create following data members
• Int basic_salary
• Int allowances
In addition, you are required to write down following class functions
• A constructor that takes values of salary & allowances at the time of object declaration.
• A method that calculates and returns the salary with following formula
o Salary = basic_salary + allowances.
In Contractual_EMP class you are required to create following data members
• Int basic_salary
• Int commission_percentage
In addition, you are required to write down following class functions
• A constructor that takes values of basic_salary & commission_percentage at the time of
object declaration.
• A method that calculates and returns the salary with following formula
o Salary = basic_salary + (basic_salary* commission_percentage/100)
In main you are required to create one object of Permanent_EMP and one object of
Contractual_EMP and implement the above given concept by using dynamic polymorphism.

Step by step
Solved in 4 steps with 4 images

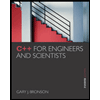
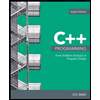
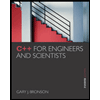
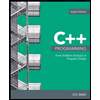