Problem Write a C++ program that will display multiple-choice trivia questions, accept the user's answers, and provide a full key and a score at the end. Question class Design and implement a class called Question. The Question class contains the following information: a stem (the text of the trivia question), an array of 4 multiple choice answers (the text of each possible answer) the letter of the correct answer (A, B, C, or D), called the key. This class will be used to represent trivia questions in a trivia game. The following operations should be available for Question objects (use the provided names). Construct a Question with no values (use empty strings for stem and answers and ‘X’ for the key). Construct a Question given its 3 components (). setStem: Set the stem question. getStem : Return the stem question. setAnswers : Set the 4 answers given an array. The answers will NOT include the letter (A, B, C, or D). getAnswer(i) : Return the single answer at index i. setKey: Set the key letter. getKey : Return the key letter. swapAnswers(a, b) Swaps two answers given two numbers a and b. Note that a and b are not indexes. a and b can be any integers. Use % to convert a and b into a value between 0 and 3, then swap the answers at these two positions. Don't forget to update the key appropriately. ask : Compose the stem and answers (but not the key), each on a separate line, as a string. Insert the letter A. before the first answer, B. before the second answer, and so on (you can use char(‘A’+i) to compute the letter for the question at index i). Put a period and space after each letter! Return the string. Save the class declaration in Question.h and save the member function definitions in Question.cpp (do not inline the member function definitions). Use the driver, QuestionTester.cpp, in the downloadable files below to test your class. You may modify this file to do more testing if you like. Don't change names, parameters or return values of these methods. Otherwise you won't be able to pass all tests. QuizDriver Design and implement a driver QuizDriver.cpp . This driver should create an array of 5 Question objects. It should then read the data provided in the file questions.txt, using it to create Question objects and storing them in the array. For each question it should shuffle the answers by swapping first with second and third with fourth answers. Then it should output each question (using the ask member function), numbering the questions 1 to 5. It should ask the user to enter the letter of their chosen answer. At the end of the quiz it should output the key to the quiz (labeled “Answers”), all on one line with spaces between the keys, and then output the number of correctly answered questio questions.txt What do you use to measure an angle? Compass Protractor Ruler T-Square B The Great Sphinx has the head of a human and the body of a what? Camel Eagle Lion Alligator C What is the flat rubber disc used in a game of ice hockey? Birdie Dart Tile Puck D What is the slowest wind speed a hurricane can have according to the Saffir-Simpson scale? 50 mph 74 mph 96 mph 110 mph B Which two planets have the most similar sized diameters? Mars and Mercury Venus and Earth Uranus and Neptune Jupiter and Saturn B
Problem
Write a C++ program that will display multiple-choice trivia questions, accept the user's answers, and provide a full key and a score at the end.
Question class
Design and implement a class called Question. The Question class contains the following information:
- a stem (the text of the trivia question),
- an array of 4 multiple choice answers (the text of each possible answer)
- the letter of the correct answer (A, B, C, or D), called the key.
This class will be used to represent trivia questions in a trivia game. The following operations should be available for Question objects (use the provided names).
- Construct a Question with no values (use empty strings for stem and answers and ‘X’ for the key).
- Construct a Question given its 3 components ().
- setStem: Set the stem question.
- getStem : Return the stem question.
- setAnswers : Set the 4 answers given an array. The answers will NOT include the letter (A, B, C, or D).
- getAnswer(i) : Return the single answer at index i.
- setKey: Set the key letter.
- getKey : Return the key letter.
- swapAnswers(a, b) Swaps two answers given two numbers a and b. Note that a and b are not indexes. a and b can be any integers. Use % to convert a and b into a value between 0 and 3, then swap the answers at these two positions. Don't forget to update the key appropriately.
- ask : Compose the stem and answers (but not the key), each on a separate line, as a string. Insert the letter A. before the first answer, B. before the second answer, and so on (you can use char(‘A’+i) to compute the letter for the question at index i). Put a period and space after each letter! Return the string.
Save the class declaration in Question.h and save the member function definitions in Question.cpp (do not inline the member function definitions). Use the driver, QuestionTester.cpp, in the downloadable files below to test your class. You may modify this file to do more testing if you like.
Don't change names, parameters or return values of these methods. Otherwise you won't be able to pass all tests.
QuizDriver
Design and implement a driver QuizDriver.cpp . This driver should create an array of 5 Question objects. It should then read the data provided in the file questions.txt, using it to create Question objects and storing them in the array. For each question it should shuffle the answers by swapping first with second and third with fourth answers. Then it should output each question (using the ask member function), numbering the questions 1 to 5. It should ask the user to enter the letter of their chosen answer. At the end of the quiz it should output the key to the quiz (labeled “Answers”), all on one line with spaces between the keys, and then output the number of correctly answered questio
questions.txt
What do you use to measure an angle?
Compass
Protractor
Ruler
T-Square
B
The Great Sphinx has the head of a human and the body of a what?
Camel
Eagle
Lion
Alligator
C
What is the flat rubber disc used in a game of ice hockey?
Birdie
Dart
Tile
Puck
D
What is the slowest wind speed a hurricane can have according to the Saffir-Simpson scale?
50 mph
74 mph
96 mph
110 mph
B
Which two planets have the most similar sized diameters?
Mars and Mercury
Venus and Earth
Uranus and Neptune
Jupiter and Saturn
B

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

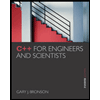
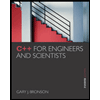