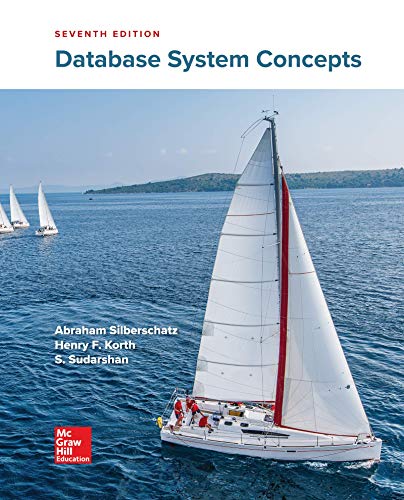
C++
Write a program that converts a number entered in Roman numerals to
decimal form. Program should consist of a class, say romanType. An
object of romanType should do the following:
a. Store the number as a Roman numeral.
b. Convert and store the number into decimal form.
c. Print the number as a Roman numeral or decimal number as requested by the user. (Write two separate functions—one to print the number as a
Roman numeral and the other to print the number as a decimal number.)
The decimal values of the Roman numerals are:
M 1000
D 500
C 100
L 50
X 10
V 5
I 1
Remember, a larger numeral preceding a smaller numeral means addition,
so LX is 60. A smaller numeral preceding a larger numeral means subtraction, so XL is 40. Any place in a decimal number, such as the 1s place, the
10s place, and so on, requires from zero to four Roman numerals.
(The program must include implementation files, .cpp and .h )

- Include header file <bits/stdc++.h> used for basic operations.
- Define function value with character type parameter x.
- Use if conditions to return the decimal number according to the alphabet in roman form.
- Define function conversion with string type parameter input.
- Define int variable res and initialize it to 0.
- Use for condition in range 0 to input length.
- Use if else condition to operate on the variable input according to greater and less than roman symbols taken together.
- Define function main.
- Define string variable inp.
- Take user input for the roman form and store in inp.
- Call function conversion and print the output.
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- python use Functionsarrow_forwardphythonarrow_forwardUsing C++ Programming language: Assume you want a function which expects as parameters an array of doubles and the size of the array. Write the function header that accepts these parameters but is defined in such a way that the array cannot be modified in the function. You can use your own variable names for the parameters.arrow_forward
- python only** define the following function: This function must return the task status for a particular task from a checklist. These examples for taskStatus demonstrate how it works. As with addTask, there's the possibility that the task specified is invalid (in this case, because it isn't in the checklist). In that case, taskStatus must print a specific message ("That task does not exist."), and must return None. Define taskStatus with 2 parameters Use def to define taskStatus with 2 parameters Use a return statement Within the definition of taskStatus with 2 parameters, use return _ in at least one place. Do not use any kind of loop Within the definition of taskStatus with 2 parameters, do not use any kind of loop.arrow_forwardpython only** define the following function: This function must set the value of a task in a checklist to True. It will accept two parameters: the checklist object to add to, and the name of the task to mark as completed. In addition to changing the task value, it must return the (now modified) checklist object that it was given. if the task name provided is not in the checklist, this function must print a specific message and must return None. Define completeTask with 2 parameters Use def to define completeTask with 2 parameters Use a return statement Within the definition of completeTask with 2 parameters, use return _ in at least one place. Do not use any kind of loop Within the definition of completeTask with 2 parameters, do not use any kind of loop.arrow_forwardIn C++, please and thank you!arrow_forward
- Create a function called getHex() as follows: • This function should return a value of type char and have a parameter of type int. • The function should use either an iflelse or a switch to return the corresponding hex value of the number as a char value. o If the number is between 0 and 9, add '0' to the parameter. o If the number is between 10 and 15, a local char variable should be set equal to the correct letter ('A', 'B', 'C', 'D', 'E', or 'F').arrow_forwardYou are assigned to develop a C++ Program using Classes, Functions, Arrays and Pointers that requires users to enter their own passwords. Your software requires that user’s passwords meet the following criteria: The password should be at least sixteen (16) characters long. The password should contain at least: one uppercase letter one lowercase letter one digit one special character (no spaces) Write a program that asks for a password and then verifies that it meets the stated criteria. If it doesn’t, the program should display a message telling the user why. Whenever possible, use pointers. Manually destroy any memory used when finished using it.arrow_forwardPlease complete this program in C++ Please enusre that there is plenty of comments. PLENTY even if unessessary. Please include screenshots of actual code, screenshots of output, and code in the browser so I can copy and paste. Thank you.arrow_forward
- Add a function to get the CPI values from the user and validate that they are greater than 0. 1. Declare and implement a void function called getCPIValues that takes two float reference parameters for the old_cpi and new_cpi. 2. Move the code that reads in the old_cpi and new_cpi into this function. 3. Add a do-while loop that validates the input, making sure that the old_cpi and new_cpi are valid values. + if there is an input error, print "Error: CPI values must be greater than 0." and try to get data again. 4. Replace the code that was moved with a call to this new function. - Add an array to accumulate the computed inflation rates 1. Declare a constant called MAX_RATES and set it to 20. 2. Declare an array of double values having size MAX_RATES that will be used to accumulate the computed inflation rates. 3. Add code to main that inserts the computed inflation rate into the next position in the array. 4. Be careful to make sure the program does not overflow the array. - Add a…arrow_forwardWrite in C++ 1. Define a struct for a soccer player that stores their name, jersey number, and total points scored. 2.Using the struct in #1, write a function that takes an array of soccer players and its size as arguments and returns the average number of points scored by the players. 3.Using the struct in #1, write a function that takes an array of soccer players and its size as arguments and returns the index of the player who scored the most points. 4.Using the struct in #1, write a function that sorts an array of soccer players by name. 5.Using the struct in #1, write a function that takes an (unsorted) array of soccer players and its size and a number as arguments, and returns the name of the soccer player with that number. It should not do any extra unnecessary work. 6.Define a function to find a given target value in an array, but use pointer notation rather than array notation whenever possible. 7.Write a swap function, that swaps the values of two variables in main, but use…arrow_forwardWrite a C++ program, you will create a class called Books to hold the information about the books in a library. The information required for each book is as follows Book Title Author name Publisher Publish year Subject Book ID (identical 5 digits’ number) Implement the following getBookInfo () member function that receives a pointer to a book and fills it up with user data. User input validation is necessary. The user should be properly prompted for each field. void getBookInfo (Books *b) Implement the following printBookInfo () member function. void printBookInfo (Books *b) Your function should print the book in the following format: Book Title : Object Oriented Programming Using C++ Author : Chris M. Szalwinski Publisher : Amazon Year : 2016 Subject : Computer Science Book Id : 00034 Note: You need to add required constructors/destructors, member functions or data members/variables in your program to complete its execution. //Missing lines of codes to be completed by the student }…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
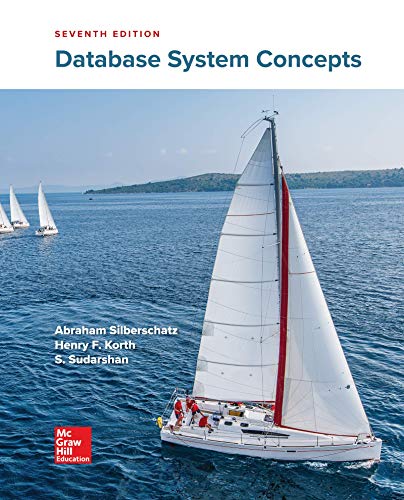
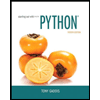
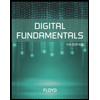
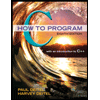
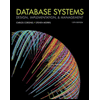
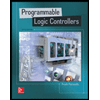