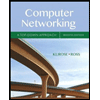
#include <bits/stdc++.h>
#include <iostream>
#include <fstream>
#include <string>
#include <cctype>
using namespace std;
// Function to print available currencies for exchange
void printCurrencies() {
cout << "Available currencies for exchange: " << endl;
cout << "SAR --> Saudi Arabia Riyal" << endl;
cout << "KWD --> Kuwaiti Dinar" << endl;
cout << "QAR --> Qatar Riyal" << endl;
cout << "AED --> United Arab Emirates Dirham" << endl;
cout << "BHD --> Bahraini Dinar" << endl;
cout << "OMR --> Omani Rial" << endl;
}
// Function to convert currency
double convertCurrency(string fromCurrency, string toCurrency, double amount) {
ifstream exchangeRateFile("ExchangeRate.txt"); // Open file for reading
string line;
double desiredRate=0;
while (getline(exchangeRateFile, line)) { // Read file line by line
int i=0;
string from, rate, to;
while (line[i] != ' ') {
i++;
}
i++;
while (line[i] != ' ') {
from += line[i];
i++;
}
i++;
while (line[i] != ' ') {
rate += line[i];
i++;
}
i++;
while (i < line.length()) {
to += line[i];
i++;
}
if(from==fromCurrency && to.substr(0,to.length()-1)==toCurrency){
desiredRate=stod(rate);
break;
}
}
exchangeRateFile.close(); // Close file
return amount * desiredRate; // Return converted amount
}
string toupper(string currency){
string s="";
for (char c : currency) {
s += toupper(c);
}
return s;
}
int main() {
printCurrencies(); // Print available currencies
string fromCurrency, toCurrency;
double amount;
cout << "Enter the currency you want to convert from: ";
cin >>fromCurrency;
fromCurrency = toupper(fromCurrency);
while(fromCurrency != "SAR" && fromCurrency != "KWD" && fromCurrency != "QAR" && fromCurrency != "AED" && fromCurrency != "BHD" && fromCurrency != "OMR"){
cout << "Invalid input, Please Enter again: ";
cin >>fromCurrency;
fromCurrency = toupper(fromCurrency);
}
cout<< "Enter the currency you want to convert to: ";
cin >>toCurrency;
toCurrency = toupper(toCurrency);
while(toCurrency != "SAR" && toCurrency != "KWD" && toCurrency != "QAR" && toCurrency != "AED" && toCurrency != "BHD" && toCurrency != "OMR"){
cout<< "Invalid input, Please Enter again: ";
cin >>toCurrency;
toCurrency = toupper(toCurrency);
}
cout<<"Enter the amount you want to convert: ";
cin >> amount;
cout << amount << " " << fromCurrency << " = " << convertCurrency(fromCurrency, toCurrency, amount) << " " << toCurrency << endl;
return 0;
}
to get the below result:
Sample run1:
The currencies we have exchange for are:
SAR--> Saudi Arabia Riyal
KWD --> Kuwaiti Dinar
QAR --> Qatar Riyal
AED--> United Arab Emirates Dirham
BHD --> Bahraini Dinar
OMR --> Omani Rial
What is the currency you have? SaR
What is the currency you want to exchange it for?
aed
How much money you want to exchange?
707
707 SAR is 692.86 AED
Sample run2:
The currencies we have exchange for are:
SAR --> Saudi Arabia Riyal
KWD --> Kuwaiti Dinar
QAR --> Qatar Riyal
AED --> United Arab Emirates Dirham
BHD --> Bahraini Dinar
OMR--> Omani Rial
What is the currency you have? Kuwaiti
Incorrect abbreviation please provide it again
Sad
Incorrect abbreviation please provide it again
SAR
What is the currency you want to exchange it for?
BHR
Incorrect abbreviation please provide it again
BHD
How much money you want to exchange?
50
50 SAR is 5 BHD
Please note that the .
- “ExchangeRate.txt” file contain:
1.00 SAR 0.08 KWD
1.00 SAR 0.97 QAR
1.00 SAR 0.98 AED
1.00 SAR 0.10 BHD
1.00 SAR 0.10 OMR
1.00 QAR 0.08 KWD
1.00 QAR 1.01 AED
1.00 QAR 0.10 BHD
1.00 QAR 0.11 OMR
1.00 KWD 12.00 AED
1.00 KWD 1.23 BHD
1.00 KWD 1.26 OMR
1.00 AED 0.10 BHD
1.00 AED 0.10 OMR
1.00 BHD 1.02 OMR

Step by stepSolved in 4 steps with 3 images

- C++languagearrow_forward// SumAndProduct.cpp - This program computes sums and products // Input: Interactive// Output: Computed sum and product #include <iostream>#include <string>void sums(int);void products(int);using namespace std; int main() { int number; cout << "Enter a positive integer or 0 to quit: "; cin >> number; while(number != 0) { // Call sums function here // Call products function here cout << "Enter a positive integer or 0 to quit: "; cin >> number; } return 0;} // End of main function// Write sums function here// Write products function herearrow_forwardC++ shapes.h: #include <iostream>#include <cmath>struct Point2d{double x;double y;void print(){// example: (2.5,3.64)std::cout << "(" << x << "," << y << ")" << std::endl;}double length() const{return std::sqrt(x * x + y * y);}// const Point2D & ==> function can't modify 'other'// the second const ==> function can't modify x, yPoint2d add(const Point2d &other) const{Point2d result;result.x = x + other.x;result.y = y + other.y;return result;}};// example of an interfacestruct Shape{virtual bool contains(Point2d p) = 0;};struct Circle : Shape{Point2d center;double radius;bool contains(Point2d p){Point2d diff;diff.x = p.x - center.x;diff.y = p.y - center.y;double distToCenter = diff.length();return distToCenter <= radius;}};// TODO: replace this with your Rectangle implementationstruct Rectangle : Shape{// TODO: which member variables to model a rectangle?bool contains(Point2d p){return false; // TODO }};//…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
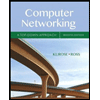
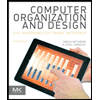
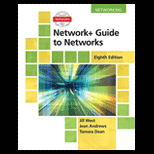
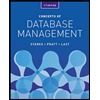
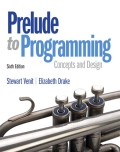
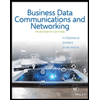