To demonstrate working with ArrayLists, we will be working with four source files: a Dessert class, two classes that extend Dessert called IceCream and Cake, and a TestDessert class. The TestDessert class contains a main() method that declares an ArrayList to hold Dessert objects. This ArrayList is referenced by the variable named "list". Examine TestDessert's main() method and notice the four comments. You are to implement code that accomplishes the tasks described in each step. For step 1, you are to populate the ArrayList with 10 IceCream and Cake objects. These objects should be inserted into the ArrayList at random. This means each run of the program should produce an ArrayList with different proportions of IceCream and Cake objects. After this operation, the ArrayList should contain 10 total objects (IceCream and Cake objects). Display the ArrayList after the operation. For step 2, if the first and last dessert in the ArrayList are different (one is IceCream and the other is Cake), make the last dessert the same as the first dessert. Display the ArrayList after the operation. For step 3, at every other index (0, 2, 4, etc.), if the dessert is not a Cake object, make it a Cake object. Display the ArrayList after the operation. For step 4, remove all the cake objects from the ArrayList. Display the ArrayList after the operation. Your TestDessert class with the required steps must compile. Therefore, you will also need to copy the Dessert, IceCream, and Cake files to your computer so TestDessert can work with them. Write the operation for each step under its comment and submit the file as a response public class Dessert { } public class IceCream extends Dessert { public String toString() { return "Ice Cream"; } } public class Cake extends Dessert { public String toString() { return "Cake"; } } import java.util.*; public class TestDessert { public static void main(String[] args) { ArrayList list = new ArrayList(); // Step 1: populate list with a total of 10 IceCream // and Cake objects at random indexes // Step 2: If the first and last desserts are different, // make the last dessert the same as the first dessert // Step 3: At every other index, if the dessert is not // cake, make it cake // Step 4: Remove all cakes }
To demonstrate working with ArrayLists, we will be working with four source files: a Dessert class, two classes that extend Dessert called IceCream and Cake, and a TestDessert class. The TestDessert class contains a main() method that declares an ArrayList to hold Dessert objects. This ArrayList is referenced by the variable named "list".
Examine TestDessert's main() method and notice the four comments. You are to implement code that accomplishes the tasks described in each step.
For step 1, you are to populate the ArrayList with 10 IceCream and Cake objects. These objects should be inserted into the ArrayList at random. This means each run of the program should produce an ArrayList with different proportions of IceCream and Cake objects. After this operation, the ArrayList should contain 10 total objects (IceCream and Cake objects). Display the ArrayList after the operation.
For step 2, if the first and last dessert in the ArrayList are different (one is IceCream and the other is Cake), make the last dessert the same as the first dessert. Display the ArrayList after the operation.
For step 3, at every other index (0, 2, 4, etc.), if the dessert is not a Cake object, make it a Cake object. Display the ArrayList after the operation.
For step 4, remove all the cake objects from the ArrayList. Display the ArrayList after the operation.
Your TestDessert class with the required steps must compile. Therefore, you will also need to copy the Dessert, IceCream, and Cake files to your computer so TestDessert can work with them.
Write the operation for each step under its comment and submit the file as a response
public class Dessert { }
public class IceCream extends Dessert {
public String toString() {
return "Ice Cream"; } }
public class Cake extends Dessert {
public String toString() {
return "Cake"; } }
import java.util.*;
public class TestDessert {
public static void main(String[] args) {
ArrayList<Dessert> list = new ArrayList<Dessert>();
// Step 1: populate list with a total of 10 IceCream
// and Cake objects at random indexes
// Step 2: If the first and last desserts are different,
// make the last dessert the same as the first dessert
// Step 3: At every other index, if the dessert is not
// cake, make it cake
// Step 4: Remove all cakes
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

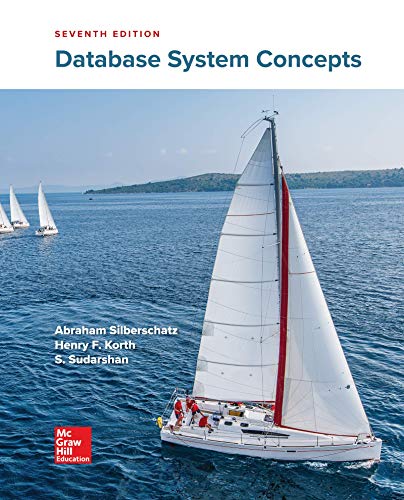
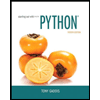
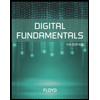
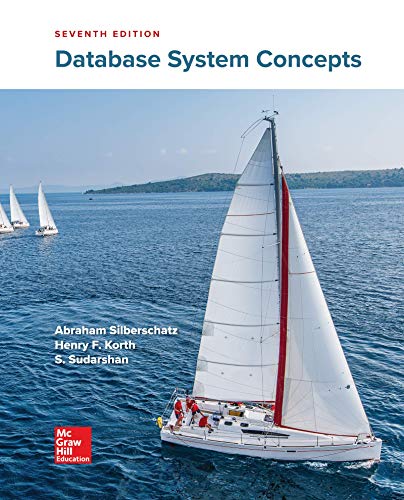
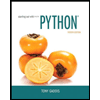
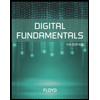
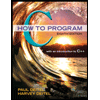
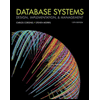
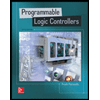