Creat a method called givePenalty(String studentID, float fine, String info, Date date) It takes the parameters: studentID, the amount of the fine in $dollars, the information description of the penalty, and the date that it occurred. This method gives the Sudent with the given studentID to get a penalty for the given fine and given info on the given date and adds it to the school directory. This method HAS to return the Penalty object that is created. There should also be error checking so if the StudentID does not exist in the school directory it does nothing. 2)Create a method called pendingPenalty(Student x) which will return a boolean=true if the student has a penalty that has a pending payment. If the penalty is paid or there is no penalty then it returns boolean = false. 3)Create a method called catchStudent(String BackpackID) This method checks if the students backpackID is reported stolen (check the reportstolen function) and it checks if the student has any pending penalties (use the pending penalty method) If the students backpack is reported stolen OR he has a pending penalty return boolean = true. If neither are true return boolean=false. 4)Create a method called displayPenaltiesFor(String StudentID) This displays a list of all the penalties that the student with the given StudentID has. At the end it should have the total of the pending penalties. Follow the following format. Use string formatting to do this. $75.00 penalty on Mon Oct 04 12:11:37 IST 2011 [paid] $3.00 penalty on Tue Dec 14 6:03:04 IST 2011 [pending payment] $75.00 penalty on Fri Apr 1 18:01:22 IST 2012 [pending payment] Total pending payments = 2 5)create a method called goodStudents() this returns a Student[] of all the students who never had any penalties. The array should be the length of all the good students (meaning not allowed to have null values) 5)create a method called displayBadStudents() this method displays all the students who have at least 1 penalty. The format of each student displayed should be (student name, number of penalties, total amount of fines they have paid) The output should look like this: Alex Jones : 1 penalty, total fines paid = $70 Mike Rodney: 5 penalty, total fines paid = $100 Jim Jackson: 3 penalty, total fines paid = $20 Emma Ander: 2 penalty, total fines paid = $80 HERE IS THE TESTING file to test your code: import java.util.GregorianCalendar; public class SchoolDirectoryTesterProgram { public static void addPenalties(SchoolDirectory sd) { sd.givePenalty("h6547-74848-jui5", 75, "Cheating during test", new GregorianCalendar(2010, 6, 14, 7, 8).getTime()).pay(); sd.givePenalty("b9547-1244re8-ud5", 175, "Skipping class", new GregorianCalendar(2011, 2, 2, 14, 22).getTime()).pay(); } public static void main(String args[]) { SchoolDirectory sd = SchoolDirectory.example(); addPenalties(sd); System.out.println("Catch Students:"); for (int i=0; i
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
JAVA
THE FIRST PART HAS ALREADY BEEN DONE. Please go to the following link and download the file to get the code I have so far and the explanations of the code.
https://gofile.io/d/dGPaF4
Make sure to read the file to understand everything of the code I have so far. Copy paste all the classes into your IDE
Please help me add methods to this code. The student, backpack, penalty classes you do not need to add anything to them. All your methods will be added to SchoolDirectory class. You cannot use Arrays.list!!!!!
There is a test class pasted at bottom. Use that to test the code. make sure it compiles and runs.
1)Creat a method called givePenalty(String studentID, float fine, String info, Date date) It takes the parameters: studentID, the amount of the fine in $dollars, the information description of the penalty, and the date that it occurred. This method gives the Sudent with the given studentID to get a penalty for the given fine and given info on the given date and adds it to the school directory. This method HAS to return the Penalty object that is created. There should also be error checking so if the StudentID does not exist in the school directory it does nothing.
2)Create a method called pendingPenalty(Student x) which will return a boolean=true if the student has a penalty that has a pending payment. If the penalty is paid or there is no penalty then it returns boolean = false.
3)Create a method called catchStudent(String BackpackID) This method checks if the students backpackID is reported stolen (check the reportstolen function) and it checks if the student has any pending penalties (use the pending penalty method)
If the students backpack is reported stolen OR he has a pending penalty return
boolean = true. If neither are true return boolean=false.
4)Create a method called displayPenaltiesFor(String StudentID) This displays a list of all the penalties that the student with the given StudentID has. At the end it should have the total of the pending penalties. Follow the following format. Use string formatting to do this.
$75.00 penalty on Mon Oct 04 12:11:37 IST 2011 [paid]
$3.00 penalty on Tue Dec 14 6:03:04 IST 2011 [pending payment]
$75.00 penalty on Fri Apr 1 18:01:22 IST 2012 [pending payment]
Total pending payments = 2
5)create a method called goodStudents() this returns a Student[] of all the students who never had any penalties. The array should be the length of all the good students (meaning not allowed to have null values)
5)create a method called displayBadStudents() this method displays all the students who have at least 1 penalty.
The format of each student displayed should be (student name, number of penalties, total amount of fines they have paid)
The output should look like this:
Alex Jones : 1 penalty, total fines paid = $70
Mike Rodney: 5 penalty, total fines paid = $100
Jim Jackson: 3 penalty, total fines paid = $20
Emma Ander: 2 penalty, total fines paid = $80
HERE IS THE TESTING file to test your code:
import java.util.GregorianCalendar;
public class SchoolDirectoryTesterProgram {
public static void addPenalties(SchoolDirectory sd) {
sd.givePenalty("h6547-74848-jui5", 75, "Cheating during test",
new GregorianCalendar(2010, 6, 14, 7, 8).getTime()).pay();
sd.givePenalty("b9547-1244re8-ud5", 175, "Skipping class",
new GregorianCalendar(2011, 2, 2, 14, 22).getTime()).pay();
}
public static void main(String args[]) {
SchoolDirectory sd = SchoolDirectory.example();
addPenalties(sd);
System.out.println("Catch Students:");
for (int i=0; i<sd.number_of_backpacks; i++)
if (sd.catchStudent(sd.backpacks[i].backpackID))
System.out.println(sd.backpacks[i]);
System.out.println("\nGood studnets:");
for (Student x: sd.goodStudents())
System.out.println(x);
System.out.println("\nBad students:");
sd.displayBadStudents();
System.out.println("\nStatus of Alex Jones:");
sd.displayPenaltiesFor("h6547-74848-jui");
}
}
Make sure the testing file compiles.
Thanks in advance for the help!!

Step by step
Solved in 2 steps

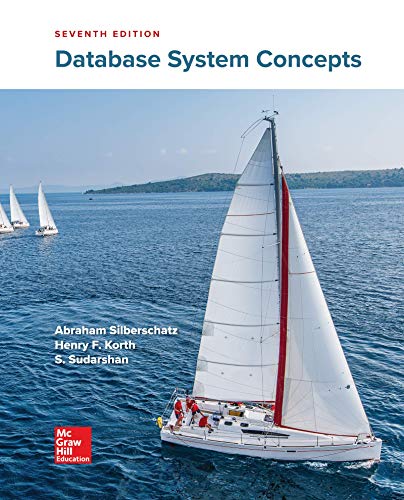
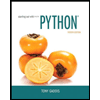
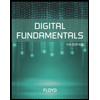
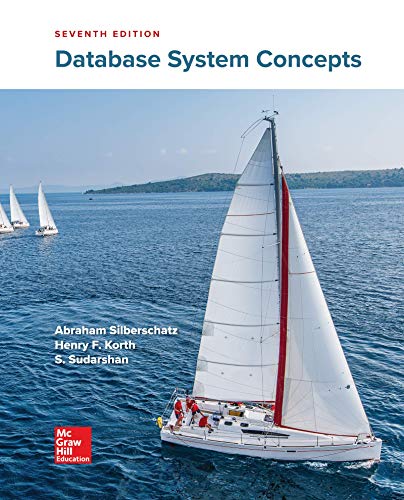
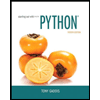
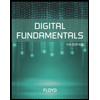
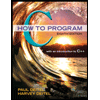
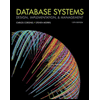
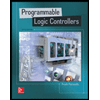