Create a flowchart for this Java Program import java.awt.BorderLayout; import java.awt.Color; import java.awt.Container; import java.awt.Dimension; import java.awt.GridLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.util.ArrayList; import javax.swing.*; public class Pincode extends JPanel implements ActionListener { JLabel display, displayExtra; JButton numButton; JButton clr; JButton etr; String displayContent = ""; String[] numPadContent = {"1", "2", "3", "4", "5", "6", "7", "8", "9", "CLEAR", "0", "ENTER"}; ArrayList buttonList; String PASSWORD = "123456"; JFrame f; Pincode(Container pane) { pane.setPreferredSize(new Dimension(320, 335)); display = new JLabel(displayContent); displayExtra = new JLabel(displayContent); display.setPreferredSize(new Dimension(320, 25)); display.setBorder(BorderFactory.createLoweredBevelBorder()); pane.add(display, BorderLayout.PAGE_START); buttonList = new ArrayList(12); JPanel numberPanel = new JPanel(); numberPanel.setLayout(new GridLayout(4, 3, 0, 0)); numberPanel.setPreferredSize(new Dimension(320, 260)); for (int i = 0; i < numPadContent.length; i++) { numButton = new JButton(numPadContent[i]); buttonList.add(numButton); } for (int n = 0; n < buttonList.size(); n++) { buttonList.get(n).addActionListener(this); numberPanel.add(buttonList.get(n)); } numberPanel.setBorder(BorderFactory.createMatteBorder(5, 5, 5, 5, Color.black)); pane.add(numberPanel, BorderLayout.LINE_END); clr = new JButton("Clear"); clr.setPreferredSize(new Dimension(160, 30)); clr.addActionListener(this); etr = new JButton("Enter"); etr.setPreferredSize(new Dimension(160, 30)); etr.addActionListener(this); pane.add(clr); pane.add(etr); } public void OptionPaneExample1(){ f=new JFrame(); JOptionPane.showMessageDialog(f,"You are authorized"); } public void OptionPaneExample2(){ f=new JFrame(); JOptionPane.showMessageDialog(f,"You are not authorized!!!"); } public void actionPerformed(ActionEvent e) { String textThere = display.getText(), textThereExtra = displayExtra.getText(); String additionalText = ""; for (int a = 0; a < buttonList.size(); a++) { if (e.getSource().equals(buttonList.get(a)) && a!=11 && a!=9) { additionalText = buttonList.get(a).getText(); } } int flag = 0; if (e.getSource().equals(buttonList.get(9))) { textThere = ""; textThereExtra = ""; flag = 1; } if(flag!=1) { display.setText(textThere.concat("*")); displayExtra.setText(textThereExtra.concat(additionalText)); } else display.setText(textThere); if (e.getSource().equals(buttonList.get(11))) { System.out.println(textThereExtra); System.out.println(textThere); if(textThereExtra.equals(PASSWORD)) { OptionPaneExample1(); } else { OptionPaneExample2(); } } } public static void main(String[] args) { JFrame frame = new JFrame(); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.getContentPane().add(new Pincode(frame)); frame.pack(); frame.setVisible(true); } }
Please don't use the online code to flowchart maker because it is not accurate.
Create a flowchart for this Java
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Container;
import java.awt.Dimension;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import javax.swing.*;
public class Pincode extends JPanel implements ActionListener {
JLabel display, displayExtra;
JButton numButton;
JButton clr;
JButton etr;
String displayContent = "";
String[] numPadContent = {"1", "2", "3", "4", "5", "6", "7", "8", "9", "CLEAR", "0", "ENTER"};
ArrayList<JButton> buttonList;
String PASSWORD = "123456";
JFrame f;
Pincode(Container pane) {
pane.setPreferredSize(new Dimension(320, 335));
display = new JLabel(displayContent);
displayExtra = new JLabel(displayContent);
display.setPreferredSize(new Dimension(320, 25));
display.setBorder(BorderFactory.createLoweredBevelBorder());
pane.add(display, BorderLayout.PAGE_START);
buttonList = new ArrayList<JButton>(12);
JPanel numberPanel = new JPanel();
numberPanel.setLayout(new GridLayout(4, 3, 0, 0));
numberPanel.setPreferredSize(new Dimension(320, 260));
for (int i = 0; i < numPadContent.length; i++) {
numButton = new JButton(numPadContent[i]);
buttonList.add(numButton);
}
for (int n = 0; n < buttonList.size(); n++) {
buttonList.get(n).addActionListener(this);
numberPanel.add(buttonList.get(n));
}
numberPanel.setBorder(BorderFactory.createMatteBorder(5, 5, 5, 5, Color.black));
pane.add(numberPanel, BorderLayout.LINE_END);
clr = new JButton("Clear");
clr.setPreferredSize(new Dimension(160, 30));
clr.addActionListener(this);
etr = new JButton("Enter");
etr.setPreferredSize(new Dimension(160, 30));
etr.addActionListener(this);
pane.add(clr);
pane.add(etr);
}
public void OptionPaneExample1(){
f=new JFrame();
JOptionPane.showMessageDialog(f,"You are authorized");
}
public void OptionPaneExample2(){
f=new JFrame();
JOptionPane.showMessageDialog(f,"You are not authorized!!!");
}
public void actionPerformed(ActionEvent e) {
String textThere = display.getText(), textThereExtra = displayExtra.getText();
String additionalText = "";
for (int a = 0; a < buttonList.size(); a++) {
if (e.getSource().equals(buttonList.get(a)) && a!=11 && a!=9) {
additionalText = buttonList.get(a).getText();
}
}
int flag = 0;
if (e.getSource().equals(buttonList.get(9))) {
textThere = "";
textThereExtra = "";
flag = 1;
}
if(flag!=1) {
display.setText(textThere.concat("*"));
displayExtra.setText(textThereExtra.concat(additionalText));
}
else
display.setText(textThere);
if (e.getSource().equals(buttonList.get(11))) {
System.out.println(textThereExtra);
System.out.println(textThere);
if(textThereExtra.equals(PASSWORD)) {
OptionPaneExample1();
}
else {
OptionPaneExample2();
}
}
}
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().add(new Pincode(frame));
frame.pack();
frame.setVisible(true);
}
}

Step by step
Solved in 3 steps with 1 images

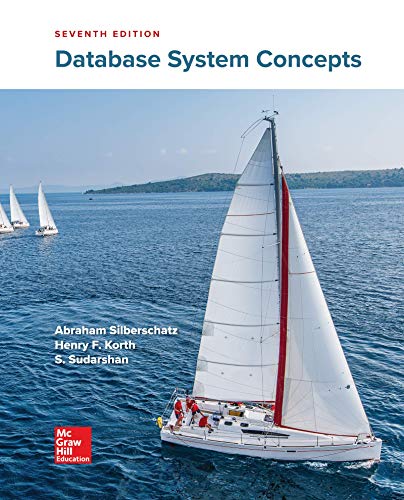
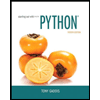
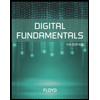
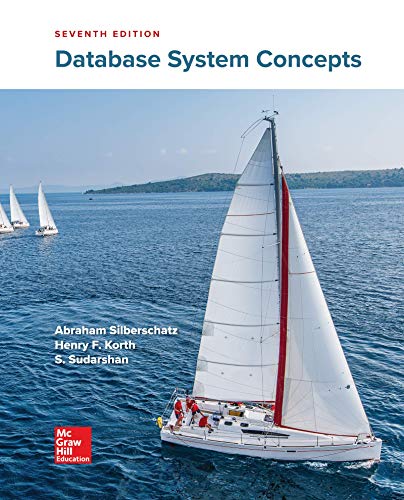
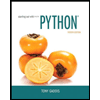
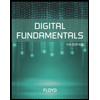
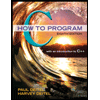
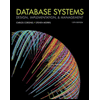
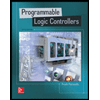