Create a function called surprise_function () which takes in two integers and adds them together. You will then create the following overloaded versions, which should do different things based on the data type passed in: Data Type Operation Integer Addition Float Division Double Modulus Char Concatenation (Return as String) Boolean Result of AND You should prompt the user for what data type they want to enter, then ask for two values of that data type. Then, call surprise_function (), pass in the values, store the result in an appropriate variable, then print the variable. Note: You must make overloaded functions for this assignment – they must all be called surprise_function (). You can not create unique, non-overloaded functions like surprise_function_booleans(). Dynamic Arrays for C++ . There are several popular compilers, but there are a few key differences between what they will and won’t allow you to do in C++. In some compilers, the following code is perfectly fine: int i = 12; int array[i]; Other compilers will throw an error because you are not using a constant to define the size of the array. This limitation obviously poses a challenge to completing certain assignments. If your compiler does not allow you to use variables to define arrays, use the following workarounds: For 1D Arrays int x = 12; int * array = new int[x]; For 2D Arrays int x = 12; int y = 24; int ** array = new int * [x]; for(int i = 0; i < x; i++){ array[i] = new int[y]; } Once you have done so, you can use the arrays as normal (e.g. array[0][0] = 34 will assign the array at index 0, 0 to the value of 34).
Create a function called surprise_function () which takes in two integers and adds them together. You will then create the following overloaded versions, which should do different things based on the data type passed in:
Data Type | Operation |
Integer | Addition |
Float | Division |
Double | Modulus |
Char | Concatenation (Return as String) |
Boolean | Result of AND |
You should prompt the user for what data type they want to enter, then ask for two values of that data type. Then, call surprise_function (), pass in the values, store the result in an appropriate variable, then print the variable.
Note: You must make overloaded functions for this assignment – they must all be called surprise_function (). You can not create unique, non-overloaded functions like surprise_function_booleans().
Dynamic Arrays for C++ . There are several popular compilers, but there are a few key differences between what they will and won’t allow you to do in C++.
In some compilers, the following code is perfectly fine:
int i = 12;
int array[i];
Other compilers will throw an error because you are not using a constant to define the size of the array. This limitation obviously poses a challenge to completing certain assignments. If your compiler does not allow you to use variables to define arrays, use the following workarounds:
For 1D Arrays
int x = 12;
int * array = new int[x];
For 2D Arrays
int x = 12;
int y = 24;
int ** array = new int * [x];
for(int i = 0; i < x; i++){
array[i] = new int[y];
}
Once you have done so, you can use the arrays as normal (e.g. array[0][0] = 34 will assign the array at index 0, 0 to the value of 34).

Step by step
Solved in 3 steps with 1 images

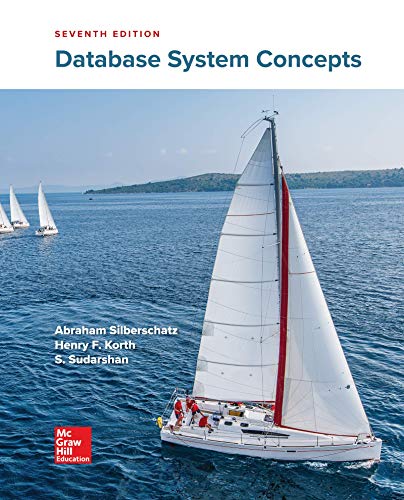
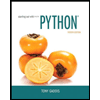
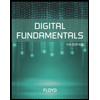
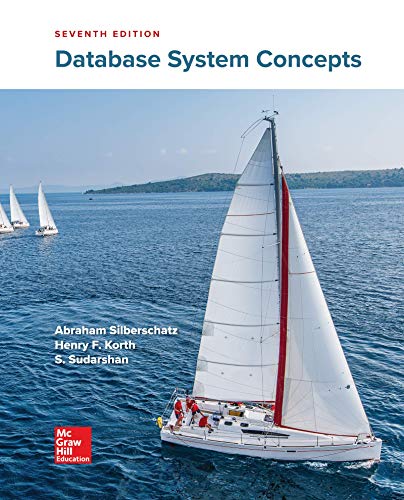
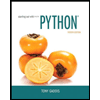
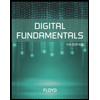
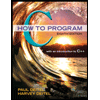
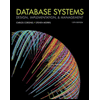
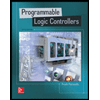