Create a new NetBeans project, called Task2. For this task you are required to write a Java 8 program that incorporates a series of Java classes that represent the core logic of an application and provides a NetBeans 8 console user interface. The required Java application relates to a proposed software system to manage some aspects of a motorboat club, including hiring motorboats and training session bookings (lessons) for any given week. Below is an initial description of the system domain. Five use cases (structured as step-by-step natural language statements) are listed in Appendix B. System domain There are two types of members: novices and motorboat licence holders (MBLH). The club employs several instructors (who are not members), and each novice is assigned to a single instructor. All members and instructors are identified by their name. An instructor can provide individual lessons to either their assigned novices or to MBLHS (who are not assigned to instructors). The club owns 10 motorboats, and each has a unique name to identify it. Lessons, which are one hour long and start on the hour (between the hours of 09:00 and 18:00), can be booked for all seven days of the week. Lessons are each taken by a single member and provided by one instructor in one of the club motorboats. MBLHS may book motorboats for hire during the day (i.e., from 09:00 to 18:00) for one hour (on the hour) without involving an instructor. A member may be scheduled to take no more than three lessons for any given week. A MBLH may have bookings for no more than two motorboat hires per week. Motorboats, instructors, and members can each be involved in only one lesson or hire at any one time. Your task is to design a Java 8 program for the motorboat management system described above, which provides a console-based (text 1/0) user interface that facilitates the five use cases listed in Appendix B. An initial analysis of the domain has already been completed, and a class model has been designed as shown in
Create a new NetBeans project, called Task2. For this task you are required to write a Java 8 program that incorporates a series of Java classes that represent the core logic of an application and provides a NetBeans 8 console user interface. The required Java application relates to a proposed software system to manage some aspects of a motorboat club, including hiring motorboats and training session bookings (lessons) for any given week. Below is an initial description of the system domain. Five use cases (structured as step-by-step natural language statements) are listed in Appendix B. System domain There are two types of members: novices and motorboat licence holders (MBLH). The club employs several instructors (who are not members), and each novice is assigned to a single instructor. All members and instructors are identified by their name. An instructor can provide individual lessons to either their assigned novices or to MBLHS (who are not assigned to instructors). The club owns 10 motorboats, and each has a unique name to identify it. Lessons, which are one hour long and start on the hour (between the hours of 09:00 and 18:00), can be booked for all seven days of the week. Lessons are each taken by a single member and provided by one instructor in one of the club motorboats. MBLHS may book motorboats for hire during the day (i.e., from 09:00 to 18:00) for one hour (on the hour) without involving an instructor. A member may be scheduled to take no more than three lessons for any given week. A MBLH may have bookings for no more than two motorboat hires per week. Motorboats, instructors, and members can each be involved in only one lesson or hire at any one time. Your task is to design a Java 8 program for the motorboat management system described above, which provides a console-based (text 1/0) user interface that facilitates the five use cases listed in Appendix B. An initial analysis of the domain has already been completed, and a class model has been designed as shown in
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter11: Inheritance And Composition
Section: Chapter Questions
Problem 5PE: Using classes, design an online address book to keep track of the names, addresses, phone numbers,...
Related questions
Question
I need some help please and thanks
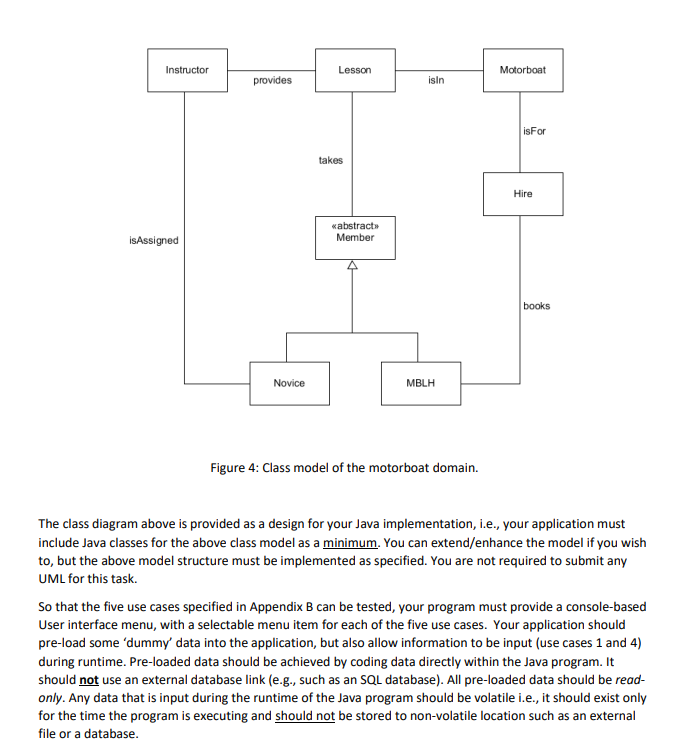
Transcribed Image Text:Instructor
Lesson
Motorboat
provides
isin
isFor
takes
Hire
«abstract»
iSAssigned
Member
books
Novice
MBLH
Figure 4: Class model of the motorboat domain.
The class diagram above is provided as a design for your Java implementation, i.e., your application must
include Java classes for the above class model as a minimum. You can extend/enhance the model if you wish
to, but the above model structure must be implemented as specified. You are not required to submit any
UML for this task.
So that the five use cases specified in Appendix B can be tested, your program must provide a console-based
User interface menu, with a selectable menu item for each of the five use cases. Your application should
pre-load some 'dummy' data into the application, but also allow information to be input (use cases 1 and 4)
during runtime. Pre-loaded data should be achieved by coding data directly within the Java program. It
should not use an external database link (e.g., such as an SQL database). All pre-loaded data should be read-
only. Any data that is input during the runtime of the Java program should be volatile i.e., it should exist only
for the time the program is executing and should not be stored to non-volatile location such as an external
file or a database.
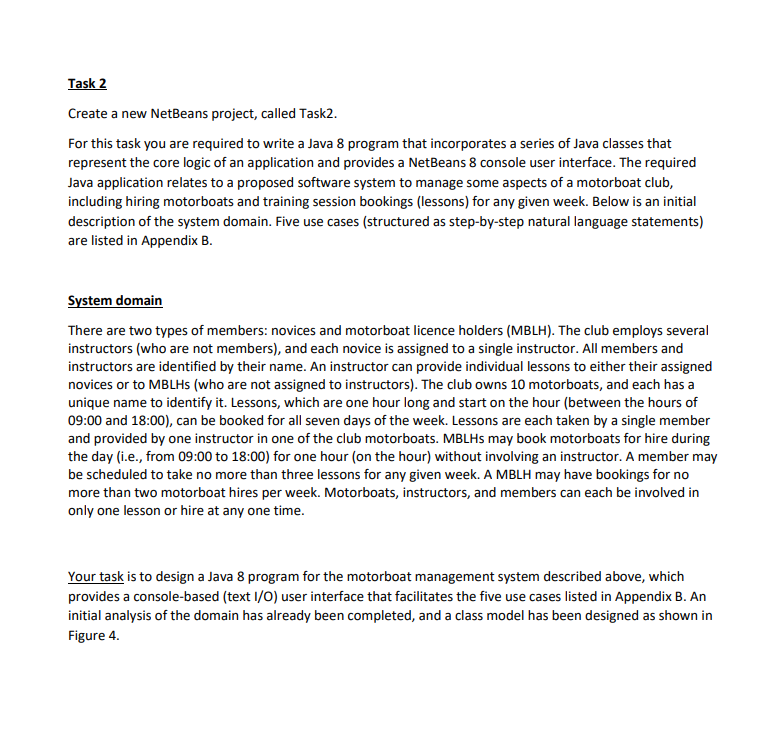
Transcribed Image Text:Task 2
Create a new NetBeans project, called Task2.
For this task you are required to write a Java 8 program that incorporates a series of Java classes that
represent the core logic of an application and provides a NetBeans 8 console user interface. The required
Java application relates to a proposed software system to manage some aspects of a motorboat club,
including hiring motorboats and training session bookings (lessons) for any given week. Below is an initial
description of the system domain. Five use cases (structured as step-by-step natural language statements)
are listed in Appendix B.
System domain
There are two types of members: novices and motorboat licence holders (MBLH). The club employs several
instructors (who are not members), and each novice is assigned to a single instructor. All members and
instructors are identified by their name. An instructor can provide individual lessons to either their assigned
novices or to MBLHS (who are not assigned to instructors). The club owns 10 motorboats, and each has a
unique name to identify it. Lessons, which are one hour long and start on the hour (between the hours of
09:00 and 18:00), can be booked for all seven days of the week. Lessons are each taken by a single member
and provided by one instructor in one of the club motorboats. MBLHS may book motorboats for hire during
the day (i.e., from 09:00 to 18:00) for one hour (on the hour) without involving an instructor. A member may
be scheduled to take no more than three lessons for any given week. A MBLH may have bookings for no
more than two motorboat hires per week. Motorboats, instructors, and members can each be involved in
only one lesson or hire at any one time.
Your task is to design a Java 8 program for the motorboat management system described above, which
provides a console-based (text I/0) user interface that facilitates the five use cases listed in Appendix B. An
initial analysis of the domain has already been completed, and a class model has been designed as shown in
Figure 4.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
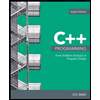
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
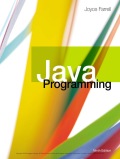
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
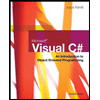
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
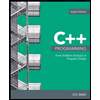
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
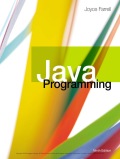
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
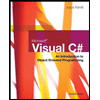
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,