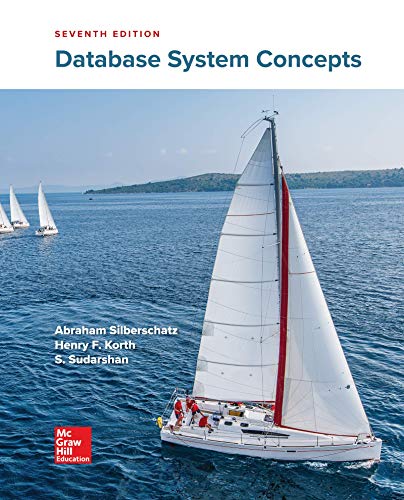
1. Introduction
CCCS 121
Object Oriented Programming language Group Course Project
An object-based application is an application that involves objects created out of classes. These objects interact with each other to fulfill certain functionalities.
The focus of this project is to develop an application written in java which involves the use of object-oriented programming. This project is a group project. As a student you will be given a chance to come up with an idea for your project. However, the project must satisfy the requirements given in this document.
This document shall be used as a set of guidelines. You are allowed to make necessary additions and/or changes to the requirements with prior approval from your instructor.
2. Requirements
Propose and implement an application, that contributes to solving a real-life problem. Your application should include at least the following:
2.1 Classes
3 to 4 classes each one of them should have:
A. B. C.
A number of private and public attributes. The private attributes (variables) will need corresponding set/get methods.
2 or more constructors, one of then should take all the class’s data members (attributes) as arguments.
Some functionality methods that can demonstrate the relationship between different classes. For example, assume that we have a system for a hotel, where a costumer can book a room. Each costumer has an ID (private) and a name. Each room has a number and price. The relationship between the Customer class and the Room class is books as illustrated in the following class diagram:
كلية علوم وهندسة الحاسب
قسم علوم الحاسب والذكاء الاصطناعي
College of Computer Science & Engineering
Department of Computer Science &
This relationship should be implemented in the Customer side as a method that takes a Room object as an argument, so when a customer books a room, the room object will be stored for example in an ArrayList or a file in case if the customer is allowed to book more than one room.
D. Each class should have a printDetails method that will print out to the user the details of an object .
E. One Main (Test) class to create objects out of the classes in your application, and to demonstrate the interaction between these objects.
2.2 Objects
A. IntheMain(Test)classcreateatleast3objectsoutofeachclassusingdifferent constructors.
B. Aftercreatingtheobjects,showhowcouldyoureadorchangethevaluesofthe private attributes.
C. Demonstrate the interaction (relationships) between these created objects. For example, assuming that you have created a customer object (c1) and a room object (r1), you can use the method books to book r1 for c1 as follows: c1.books(r1);
D. Make a demo that shows the details to the user for each created object.
2.3 Files and Arrays
A. When you create an object in the Test class, read the values of the object variables from the user (e.g., the value of an ID).
B. After creating the objects store them temporally in an array (ArraList preferable). Then store them permanently in a file.
C. When printing out the detail for an object read them from that file.
كلية علوم وهندسة الحاسب
قسم علوم الحاسب والذكاء الاصطناعي
College of Computer Science & Engineering
Department of Computer Science & Artificial Intelligence
2.4 Input Validation
A. Applyinputvalidationwhereneeded,forexample,intheexamplegivenabove,the price of a room should not be a negative value, or you can’t have two customers with the same ID.
2.5 Inheritance (optional)
Apply the inheritance in your application where possible.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Portfolio Instructions: You are working for a financial advisor who creates portfolios of financial securities for his clients. A portfolio is a conglomeration of various financial assets, such as stocks and bonds, that together create a balanced collection of investments. When the financial advisor makes a purchase of securities on behalf of a client, a single transaction can include multiple shares of stock or multiple bonds. It is your job to create an object-oriented application that will allow the financial advisor to maintain the portfolios for his/her clients. You will need to create several classes to maintain this information: Security, Stock, Bond, Portfolio, and Date. The characteristics of stocks and bonds in a portfolio are shown below: Stocks: Bonds: Purchase date (Date) Purchase date (Date) Purchase price (double)…arrow_forwardObject-Oriented Programming OvalDraw Plus (Java code) Summary: Create a graphical application with Java that that draws an oval Prerequisites: Java, VS Code, and Terminal Create a graphical Java application that runs on Microsoft Windows and MacOS that draws an oval centered in the main application window when the programming starts. The oval should automatically resize and reposition itself when the window is resized. In this activity you will start with our OvalDraw application in our sample code, review the code, and then make incremental improvements to the application. Be sure to make the resulting application uniquely your own by adding standard comments at the top application (i.e. your name, class, etc.), changing the names of variables, and adding small features. Finally, be sure to save your work as you will often be asked to submit it as part of an assignment. Be sure to review the example OvalDraw project in the Java section of our example code repository. Requirement 1:…arrow_forwardObject oriented programming is where you focus more of the data and the properties that make that data up, along with the actions you can preform by that data, rather than focusing on doing some procedural thing. Group of answer choices True Falsearrow_forward
- Object adapters and class adapters each provide a unique function. These concepts are also significant due to the significance that you attach to them.arrow_forwardJAVA programming languagearrow_forwardDescribe what a "class" is in terms of object-oriented design and programming. You are welcome to use an example, but you must also explain the concept.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
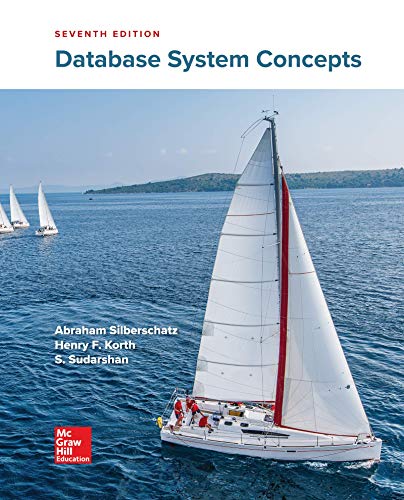
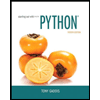
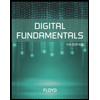
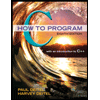
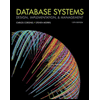
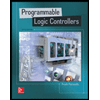