Create a Right Triangle class that has two sides. Name your class rightTraingle. Code getter and setters for the base and the height. (Remember class variables are private.) The class should include a two-argument constructor that allows the program to set the base and height. The constructor should verify that all the dimensions are greater than 0. before assigning the values to the private data members. If a side is not greater than zero, set the value to -1. The class also should include two value-returning methods. One value-returning method should calculate the area of a triangle, and the other should calculate the perimeter of a triangle. If either side is -1, these functions return a -1. The formula for calculating the area of a triangle is 1/2 *b*h, where b is the base and h is the height. The formula for calculating the perimeter of a triangle is b+h+sqrt (b*b +h*h). Be sure to include a default constructor that initializes the variables of the base, height to -1. To test create a program(main function) that uses the two-argument constructor to set the triangle's base and height and then displays the triangle's area and perimeter amounts. Then use the no- argument constructor to do the same. You can use the main function below to implement these tests. Save and then run the program. Test the program using the main function shown below. Also shown is the expected output. int main() { iinstatiate the rightTriangle object rightTriangle testīriangle(3, 4); cout « "The perimeter is - « test Triangle.getPerimeter() <« endl; cout « "The area is " « testTriangle.getArea () « endl; rightTriangle anotherTriangle; cout « "The perimeter is « another Triangle.getperimeter() « endl; cout « "The area is " « anotherTriangle.getarea () << endl; return e;
Create a Right Triangle class that has two sides. Name your class rightTraingle. Code getter and setters for the base and the height. (Remember class variables are private.) The class should include a two-argument constructor that allows the program to set the base and height. The constructor should verify that all the dimensions are greater than 0. before assigning the values to the private data members. If a side is not greater than zero, set the value to -1. The class also should include two value-returning methods. One value-returning method should calculate the area of a triangle, and the other should calculate the perimeter of a triangle. If either side is -1, these functions return a -1. The formula for calculating the area of a triangle is 1/2 *b*h, where b is the base and h is the height. The formula for calculating the perimeter of a triangle is b+h+sqrt (b*b +h*h). Be sure to include a default constructor that initializes the variables of the base, height to -1. To test create a program(main function) that uses the two-argument constructor to set the triangle's base and height and then displays the triangle's area and perimeter amounts. Then use the no- argument constructor to do the same. You can use the main function below to implement these tests. Save and then run the program. Test the program using the main function shown below. Also shown is the expected output. int main() { iinstatiate the rightTriangle object rightTriangle testīriangle(3, 4); cout « "The perimeter is - « test Triangle.getPerimeter() <« endl; cout « "The area is " « testTriangle.getArea () « endl; rightTriangle anotherTriangle; cout « "The perimeter is « another Triangle.getperimeter() « endl; cout « "The area is " « anotherTriangle.getarea () << endl; return e;
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter11: Inheritance And Composition
Section: Chapter Questions
Problem 9PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
C++ code help needed. C++ only please.
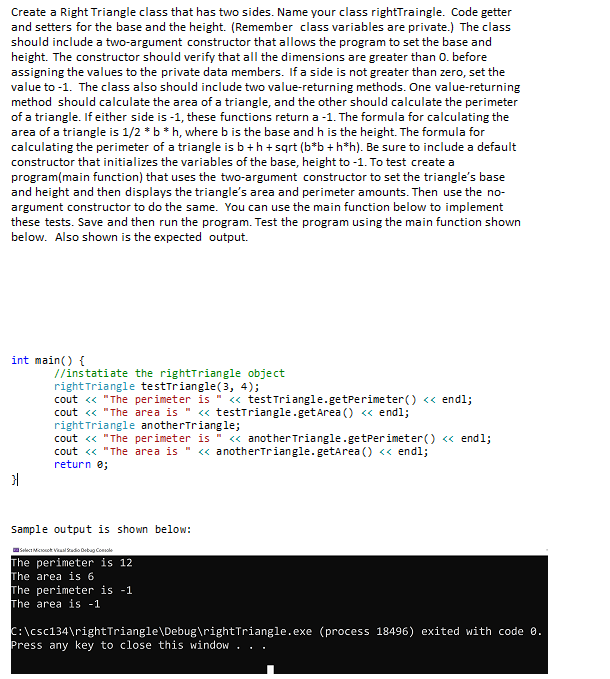
Transcribed Image Text:Create a Right Triangle class that has two sides. Name your class rightTraingle. Code getter
and setters for the base and the height. (Remember class variables are private.) The class
should include a two-argument constructor that allows the program to set the base and
height. The constructor should verify that all the dimensions are greater than 0. before
assigning the values to the private data members. If a side is not greater than zero, set the
value to -1. The class also should include two value-returning methods. One value-returning
method should calculate the area of a triangle, and the other should calculate the perimeter
of a triangle. If either side is -1, these functions return a -1. The formula for calculating the
area of a triangle is 1/2 *b * h, where b is the base and h is the height. The formula for
calculating the perimeter of a triangle is b +h + sqrt (b*b + h*h). Be sure to include a default
constructor that initializes the variables of the base, height to -1. To test create a
program(main function) that uses the two-argument constructor to set the triangle's base
and height and then displays the triangle's area and perimeter amounts. Then use the no-
argument constructor to do the same. You can use the main function below to implement
these tests. Save and then run the program. Test the program using the main function shown
below. Also shown is the expected output.
int main() {
//instatiate the rightTriangle object
rightTriangle testriangle(3, 4);
cout « "The perimeter is " <« testTriangle.getPerimeter() « endl;
cout « "The area is " « testīriangle.getArea () « endl;
rightTriangle anotherTriangle;
cout « "The perimeter is " <« another Triangle.getPerimeter() « endl;
cout « "The area is " « anotherTriangle.getarea () <« endl;
return e;
Sample output is shown below:
Select Mcsosot Viasl Sudio Debag Console
The perimeter is 12
The area is 6
The perimeter is -1
The area is -1
C:\csc134\rightTriangle\Debug\rightTriangle.exe (process 18496) exited with code 0.
Press any key to close this window
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
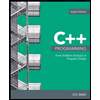
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
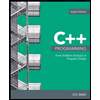
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning