There are common attributes and methods between kids and trainers. What is the best choice for designing and writing the codes of these two classes? Explain your answer Kid.java public class Kid { private static int idStatic=0; private int id; private String name; private int age; private char gender; public Kid(String name, int age, char gender) { super(); this.id = idStatic++; this.name = name; this.age = age; this.gender = gender; } public static int getIdStatic() { return idStatic; } public int getId() { return id; } public String getName() { return name; } public int getAge() { return age; } public char getGender() { return gender; } @Override public String toString() { return "Kid [id=" + id + ", name=" + name + ", age=" + age + ", gender=" + gender + "]"; } } Trainer.java public class Trainer { private static int idStatic = 0; private int id; private String name; private int age; private char gender; private double salary; public Trainer(String name, int age, char gender, double salary) { super(); this.id = idStatic++; ; this.name = name; this.age = age; this.gender = gender; this.salary = salary; } public static int getIdStatic() { return idStatic; } public int getId() { return id; } public String getName() { return name; } public int getAge() { return age; } public char getGender() { return gender; } public double getSalary() { return salary; } @Override public String toString() { return "Trainer [id=" + id + ", name=" + name + ", age=" + age + ", gender=" + gender + ", salary=" + salary + "]"; } } Group.java import java.util.ArrayList; public class Group { private static int staticNumber = 0; private int groupNumber; private Trainer trainer; private String sportName; private ArrayList kids; private final static int MAX_LIMIT = 10; public Group(Trainer t, String sport) { groupNumber = staticNumber++; this.trainer = t; this.sportName = sport; kids = new ArrayList<>(); } public static int getStaticNumber() { return staticNumber; } public int getGroupNumber() { return groupNumber; } public Trainer getTrainer() { return trainer; } public String getSportName() { return sportName; } public ArrayList getKids() { return kids; } public boolean addKid(Kid k) { if (kids.size() < MAX_LIMIT) { kids.add(k); return true; } else { return false; } } public boolean removeKid(Kid k) { return kids.remove(k); } }
There are common attributes and methods between kids and trainers. What is the best choice for designing and writing the codes of these two classes? Explain your answer
Kid.java
public class Kid {
private static int idStatic=0;
private int id;
private String name;
private int age;
private char gender;
public Kid(String name, int age, char gender) {
super();
this.id = idStatic++;
this.name = name;
this.age = age;
this.gender = gender;
}
public static int getIdStatic() {
return idStatic;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public char getGender() {
return gender;
}
@Override
public String toString() {
return "Kid [id=" + id + ", name=" + name + ", age=" + age + ", gender=" + gender + "]";
}
}
Trainer.java
public class Trainer {
private static int idStatic = 0;
private int id;
private String name;
private int age;
private char gender;
private double salary;
public Trainer(String name, int age, char gender, double salary) {
super();
this.id = idStatic++;
;
this.name = name;
this.age = age;
this.gender = gender;
this.salary = salary;
}
public static int getIdStatic() {
return idStatic;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public char getGender() {
return gender;
}
public double getSalary() {
return salary;
}
@Override
public String toString() {
return "Trainer [id=" + id + ", name=" + name + ", age=" + age + ", gender=" + gender + ", salary=" + salary
+ "]";
}
}
Group.java
import java.util.ArrayList;
public class Group {
private static int staticNumber = 0;
private int groupNumber;
private Trainer trainer;
private String sportName;
private ArrayList<Kid> kids;
private final static int MAX_LIMIT = 10;
public Group(Trainer t, String sport) {
groupNumber = staticNumber++;
this.trainer = t;
this.sportName = sport;
kids = new ArrayList<>();
}
public static int getStaticNumber() {
return staticNumber;
}
public int getGroupNumber() {
return groupNumber;
}
public Trainer getTrainer() {
return trainer;
}
public String getSportName() {
return sportName;
}
public ArrayList<Kid> getKids() {
return kids;
}
public boolean addKid(Kid k) {
if (kids.size() < MAX_LIMIT) {
kids.add(k);
return true;
} else {
return false;
}
}
public boolean removeKid(Kid k) {
return kids.remove(k);
}
}

Step by step
Solved in 2 steps

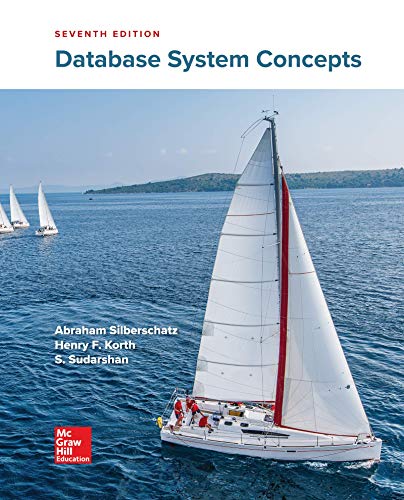
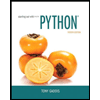
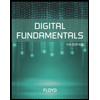
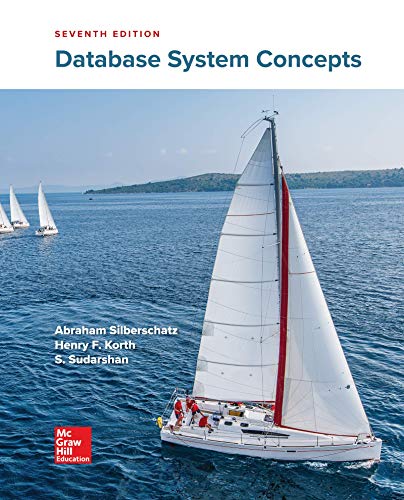
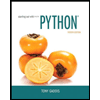
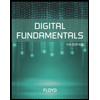
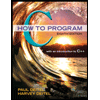
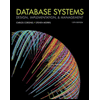
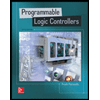