Create a social network, Chirper, that lets the user add new Chirps and like existing Chirps. It's lonely because only one person can add and like messages -- so there's really not much "social" about it. At least you can practice using objects, references, and function overloading in C++!
Create a social network, Chirper, that lets the user add new Chirps and like existing Chirps. It's lonely because only one person can add and like messages -- so there's really not much "social" about it. At least you can practice using objects, references, and function overloading in C++!
Here's an example of how this program will run
You can "chirp" a new message to Chirper, or "like" an existing chirp, or "exit". What do you want to do? chirp
What's your message? This is my first chirp!
Chirper has 1 chirps:
1. This is my first chirp! (0 likes)
You can "chirp" a new message to Chirper, or "like" an existing chirp, or "exit". What do you want to do? like
Which index do you want to like? 1
Chirper has 1 chirps:
1. This is my first chirp! (1 likes)
You can "chirp" a new message to Chirper, or "like" an existing chirp, or "exit". What do you want to do? chirp
What's your message? Second chirp is the best chirp.
Chirper has 2 chirps:
1. This is my first chirp! (1 likes)
2. Second chirp is the best chirp (0 likes)
You can "chirp" a new message to Chirper, or "like" an existing chirp, or "exit". What do you want to do? exit
Goodbye!
Create the Chirp class
Chirp objects need two member variables:
one to track the message, and
one to track the number of likes.
For the Chirp class constructors, use member initializer notation to initialize the member variables. You should define the following constructors:
A default constructor, which initializes the message to the empty string "", and initializes the number of likes to 0.
A non-default constructor, which accepts a message as input and initializes the message to that input.
A non-default constructor, which accepts a message as input and initializes the message to that input, and also accepts a like count as input, and initializes the like count to that.
The Chirp class should have the following member functions:
SetMessage which takes a std::string,
GetMessage which returns a std::string,
GetLikes which returns an integer,
AddLike which takes in no input increments the number of likes by 1, and
AddLike which takes in an integer input to increment the number of likes by. Notice that the function names are the same, but accept different parameters. This is the power of function overloading!
Put the Chirp function declarations in chirp.h and the Chirp function definitions in chirp.cc. Reference functions review.
Create main.cc
main.cc will implement the not-so-social network, Chirper. We've provided some input and output code, but haven't yet created any Chirp objects. You need to:
Create a std::
Create new Chirp objects when the user wants to add a new message.
Add likes to Chirps based on user input.
Complete the functionality to print the full list of chirps to the console. You should print the total number of chirps, and then you should have one line for each chirp, starting with the (1-based) index of the chirp, the message, and then the number of likes, for example:
Chirper has 2 chirps: 1. This is my first chirp! (1 likes) 2. 2021 can't come soon enough (3 likes)
Notes: we aren't checking for grammar (1 chirps vs 1 chirp). You can assume that the user will not enter invalid input.
Ensure you include chirp.h in main.cc so the compiler can find the function declarations.
main.cc file
#include <iostream>
#include <string>
#include <vector>
#include "chirp.h"
int main() {
std::string input;
const std::string prompt =
"\nYou can \"chirp\" a new message to Chirper, "
"\"like\" an existing chirp, or \"exit\". What do you want to do? ";
std::cout << prompt;
std::getline(std::cin, input);
// ======= YOUR CODE HERE =======
// 1. Create a vector to hold your chirps.
// Don't forget to #include <vector> and
// the header file for the Chirp class.
//
while (input != "exit") {
if (input == "chirp") {
std::string user_message;
std::cout << "What's your message? ";
std::getline(std::cin, user_message);
// ==== YOUR CODE HERE ======
// 2. Create a new Chirp object and set the message to
// user_message. Add the new chirp to the vector.
//
} else if (input == "like") {
std::string user_index_string;
std::cout << "Which index do you want to like? ";
std::getline(std::cin, user_index_string);
int user_index = std::stoi(user_index_string);
// ========== YOUR CODE HERE ========
// 3. Add a like to the Chirp object at index user_index
// in the vector. You will need to offset user_index
// to the zero-based indexing that matches your vector.
//
}
// ========= YOUR CODE HERE =======
// 4. Print the contents of the chirp vector. You will need
// to loop through the entries in the vector.
// Check your formatting matches the README:
// Show the user indices starting at 1 instead of 0,
// and include the number of likes.
//
std::cout << prompt;
std::getline(std::cin, input);
}
return 0;
}
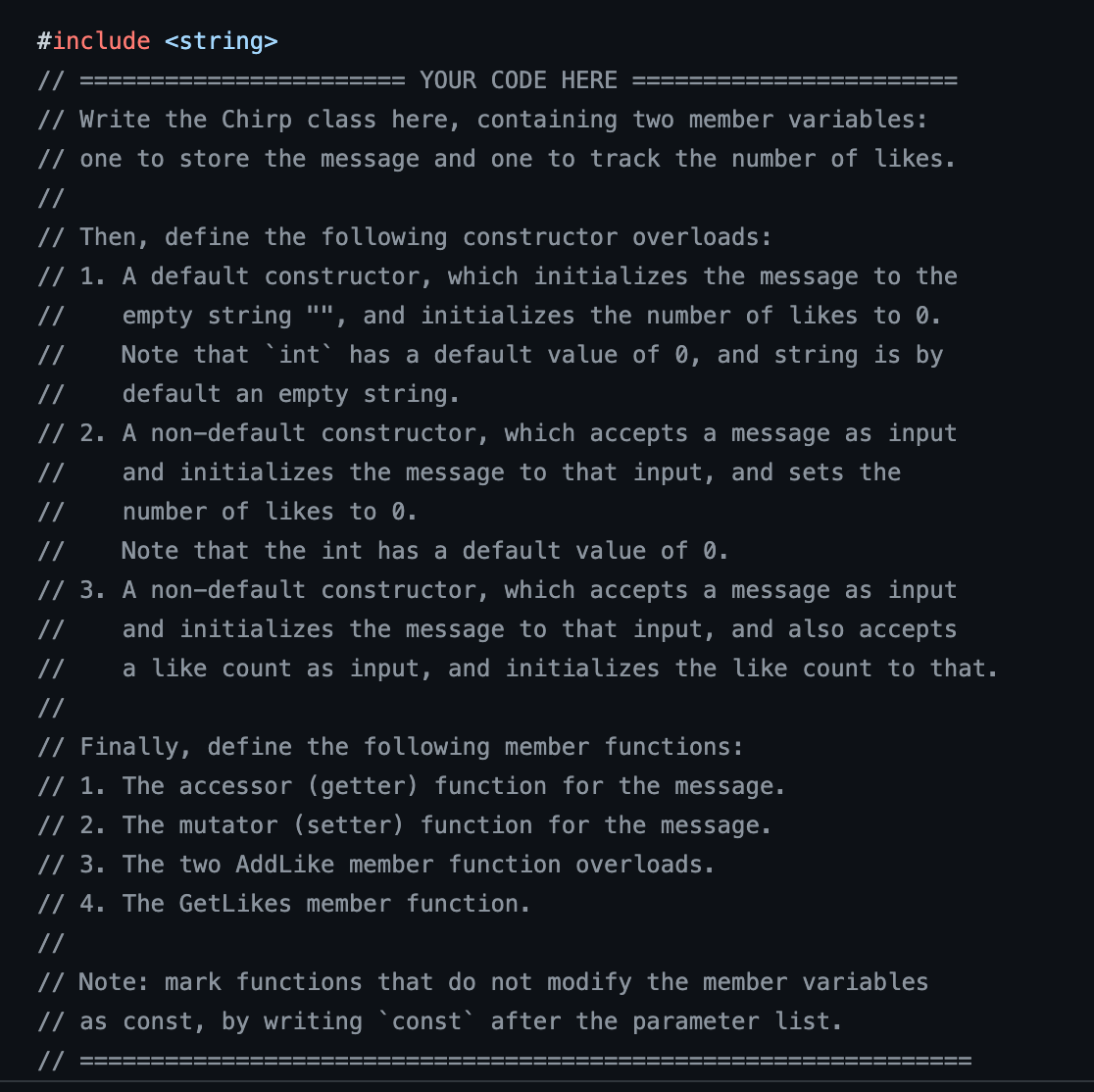
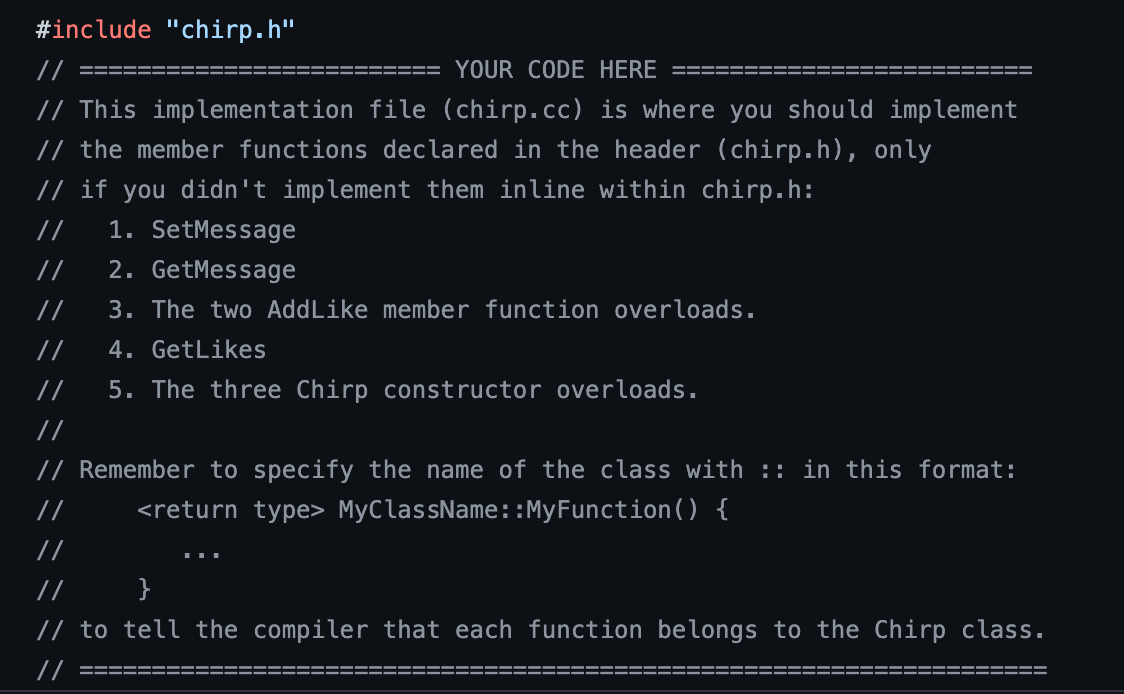

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

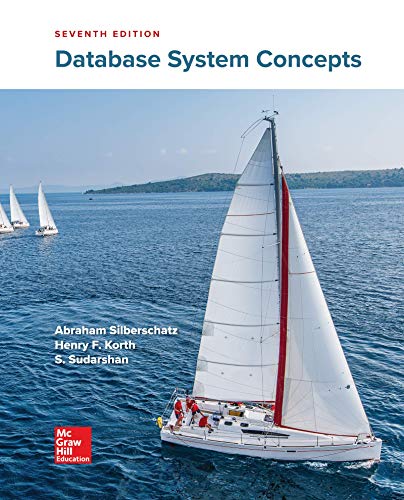
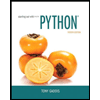
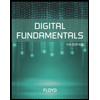
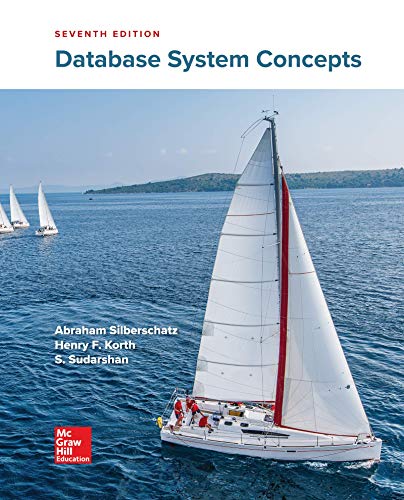
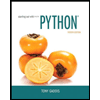
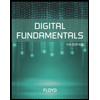
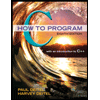
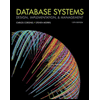
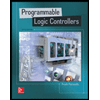